filmov
tv
C++ Weekly - Ep 289 - Returning From The `void`
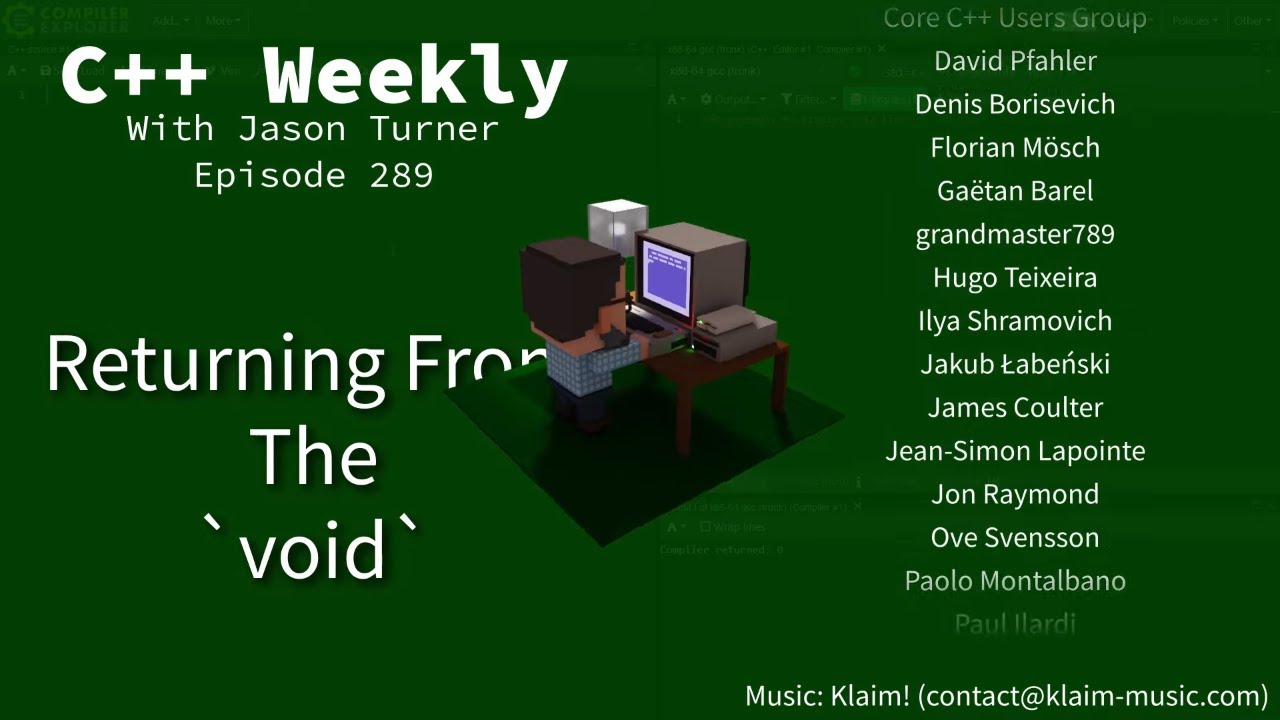
Показать описание
☟☟ Awesome T-Shirts! Sponsors! Books! ☟☟
T-SHIRTS AVAILABLE!
WANT MORE JASON?
SUPPORT THE CHANNEL
GET INVOLVED
JASON'S BOOKS
► C++23 Best Practices
► C++ Best Practices
JASON'S PUZZLE BOOKS
► Object Lifetime Puzzlers Book 1
► Object Lifetime Puzzlers Book 2
► Object Lifetime Puzzlers Book 3
► Copy and Reference Puzzlers Book 1
► Copy and Reference Puzzlers Book 2
► Copy and Reference Puzzlers Book 3
► OpCode Puzzlers Book 1
RECOMMENDED BOOKS
AWESOME PROJECTS
O'Reilly VIDEOS
T-SHIRTS AVAILABLE!
WANT MORE JASON?
SUPPORT THE CHANNEL
GET INVOLVED
JASON'S BOOKS
► C++23 Best Practices
► C++ Best Practices
JASON'S PUZZLE BOOKS
► Object Lifetime Puzzlers Book 1
► Object Lifetime Puzzlers Book 2
► Object Lifetime Puzzlers Book 3
► Copy and Reference Puzzlers Book 1
► Copy and Reference Puzzlers Book 2
► Copy and Reference Puzzlers Book 3
► OpCode Puzzlers Book 1
RECOMMENDED BOOKS
AWESOME PROJECTS
O'Reilly VIDEOS
C++ Weekly - Ep 289 - Returning From The `void`
Marimayam | Ep 289 -An argument towards the 'Pretty Chair | Mazhavil Manorama
Casa Iubirii (29.10.2024) - Episodul 289 | Sezonul 3 | Editie COMPLETA
Un si grand soleil - Episode 289 (Saison 2) | Les révélations
Ep:289 STRATEGIES TO MAKE A KETO LIFESTYLE PERMANENT
L'gosseux d'bois Ep 289 - Une dépense pour le chalet partie 1
STILL struggling to get to Grenada: OUR PROP FELL OFF! (Ep 289)
WATANZAD - EP 289 - Pakistani Spy Saved Boy from Harasser Police Constable - Roxen Original
Barbie - The Best of the Twins | Ep.289
Passing By Reference Demo | Ep. 289 | C Programming Language
Has The Tour de France Really Changed?! | The GCN Show Ep. 289
Tanisha's final call! | S1 | Ep.290 | Faltu
Pahinja Parawa Episode 289 Sindhi Drama | Sindhi Dramas 2022
Barbie - What Happened To Your Hair! | Ep.293
Barbie - The School Play | Ep.291
Rajya Sabha Question Hour : Ep - 289 (Eng)
The Roth 401K and Meal Planning Made Easy | EP 289
arcade x ashqui bazi hy taash ki #shaheersheikh #rheasharma #rhesha #mishbir #lovestatus #sadstatus
Orhun, Hira’ya misafir oluyor ❤️ | Esaret 289.Bölüm
Self-Care ep. 289
Turn the Page Podcast - Episode 289 C: Katherine Reay
|| His Name is Jesus || Episode 289 || APPEARED BEFORE ANNAS ||
🤞har janam sath 🥳 sameer naina💖cute couples 😘
Fishing, Riverside Overnight Shelter: Survival Alone | EP.289
Комментарии