filmov
tv
C Programming Tutorial 44 - Coding Challenge
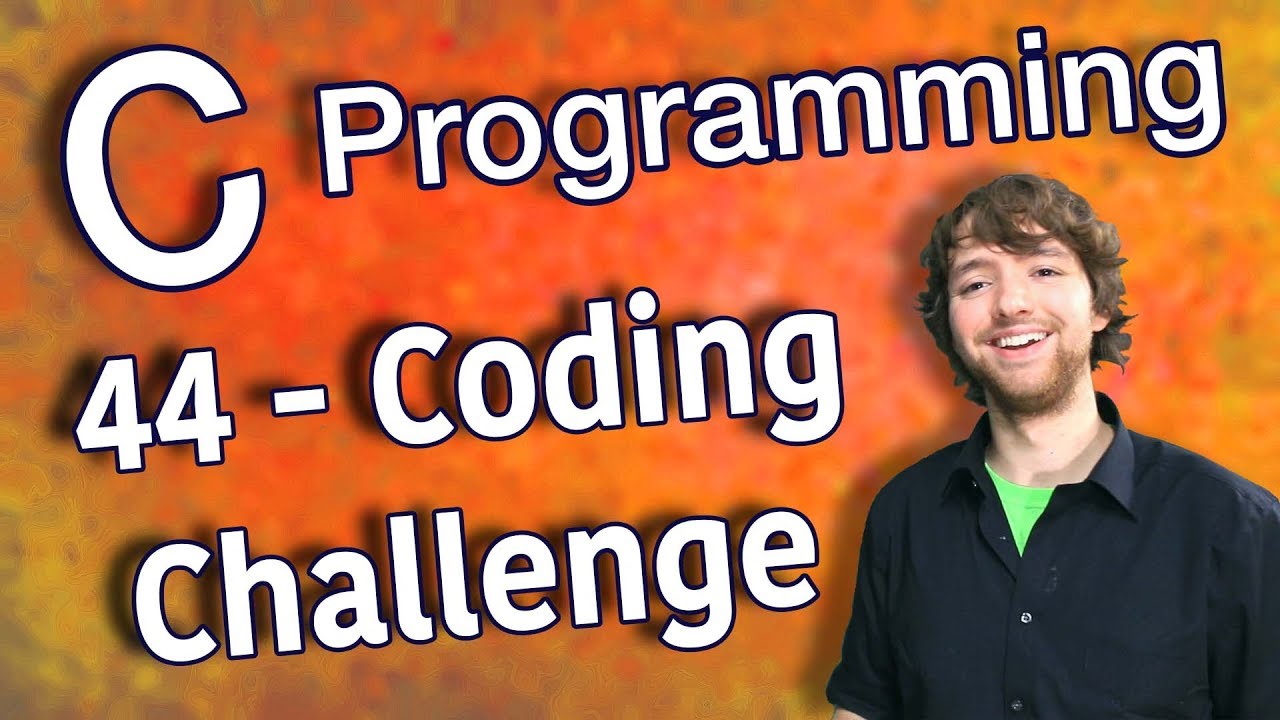
Показать описание
~~~~~~~~~~~~~~~ CONNECT ~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~ SUPPORT ME ~~~~~~~~~~~~~~
🅑 Bitcoin - 3HnF1SWTzo1dCU7RwFLhgk7SYiVfV37Pbq
🅔 Eth - 0x350139af84b60d075a3a0379716040b63f6D3853
C Programming Tutorial 44 - Coding Challenge
C Programming Tutorial - 44 - Arrays and Pointers
C Programming Tutorial 44, Enumerations
C Programming Tutorial - 44: Functions (Part-2) Parameter Lists, Actual & Formal Parameters
C Programming Tutorial 44 Arrays and Pointers HD
C Programming Tutorial 44 Arrays and Pointers
C Programming Tutorial # 44 - Deleting Data From A File - Code - Part 1 [HD]
C Programming Tutorial 44 Arrays and Pointers
How to Secure Coveted Sagrada Familia Tickets Online | Instantly Book Gaudi House Museum Tickets
C Programming Tutorial 44 (Arrays and Pointers)
C++Tutorial for Beginners 44 - Const; a Vital Tool for Reducing Bugs
C Programming Tutorial 44 Enumerations
C Programming Tutorial 44 - Deleting Data From A File - Code - Part 1
C Programming All-in-One Tutorial Series (10 HOURS!)
C Programming Tutorial - 44 - Dereference Pointer
C Programming Tutorial 44 Arrays and Pointersvia torchbrowser com 1
C Programming Tutorial 44 Arrays and Pointers
if and if-else Statement in C - C Programming Tutorial 44
C Programming Tutorial for Beginners
C++ Programming Tutorial 44 - Creating a Guessing Game
C Programming Tutorial # 44 - Deleting Data From A File - Code - Part 3 [HD]
C Programming Tutorial # 44 - Deleting Data From A File - Code - Part 2 [HD]
Learn C Language In 10 Minutes!! C Language Tutorial
part 44 1000 c language programs in telugu | C langauge tutorials in telugu | Learn c in telugu
Комментарии