filmov
tv
JWT Authentication System in Django and React.js Full Stack Application | Complete Project Tutorial
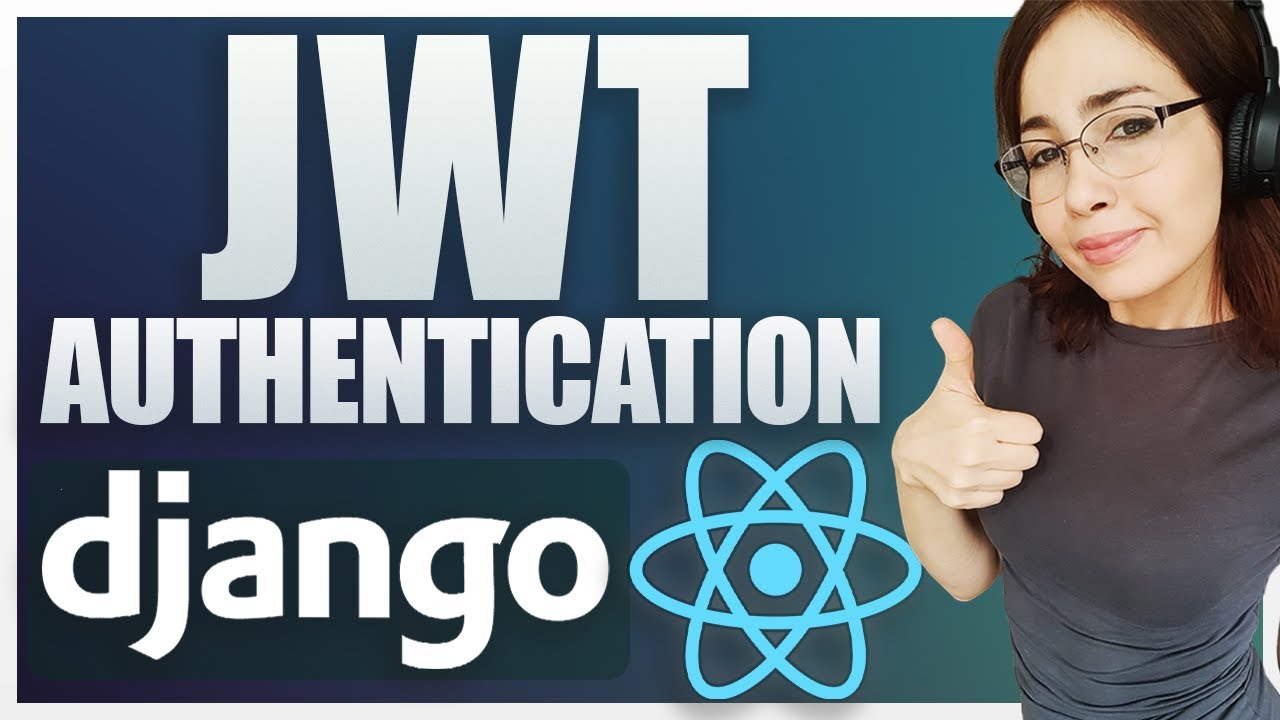
Показать описание
We will create Restful APIs to handle user registration, login, logout, user information retrieval, and token refreshing using Django Rest Framework. After that we will consume our API endpoints by making HTTP requests using Axios in our frontend application.
Following along this video should help you:
Learn more about authentication and authorization and gain a deeper understanding of how authentication and authorization mechanisms work, especially with JWT tokens and the Django Rest Framework.
Understand RESTful API Development, and how to develop RESTful APIs using Django Rest Framework, including creating endpoints for user registration, login, logout, and retrieving user information.
Learn about Frontend-Backend Integration: You'll learn how to integrate a frontend application (built with React) with a backend API (built with Django), including making HTTP requests, handling responses, and managing user sessions.
🕗 Chapters:
00:00 - Objectives
Project Setup:
00:46 - Create Project Directory + frontend + backend directories
Backend Setup:
01:26 - Create venv environment in backend directory
02:07 - Install Django
02:32 - Start Django project
03:11 - Create Accounts Application
Models:
03:40 - Define Custom User Model
Forms:
06:39 - Create User Creation Form
07:48 - Create User Change form for updating user info in Django admin
Admin:
08:34 - Create Super User
09:40 - Register User Model and Forms in Admin
Auth APIs:
12:07 Install Django Rest Framework
12:48 - Install Django Rest Framework Simple JWT and set it up as Rest Framework’s Default Authentication Class
13:35 - Configure JWT Settings
Serializers:
15:16 - Serializers
15:55 - Define Custom User Serializer
16:29 - Define User Registration Serializer
21:52 - Define User Login Serializer
Views:
24:07 - Define User Registration View (Inherit from GenericAPIView)
28:57 - Define User Login View (Inherit from GenericAPIView)
31:30 - Define User Logout View
URLs:
33:53 - Configure URL patterns
Cross-Origin Resource Sharing (CORS) :
37:88 - Install Django CORS Headers and set allowed origins
Frontend:
39:36 - Create React Application with Vite
40:54 - Create pages/routes [ Layout , Home , Register , Login ]
Routing:
42:09 - Install React Router DOM and Create Routes
Registration/Login on the Frontend:
55:44 - Install Axios
57:13 - Post Request to Register Endpoint
01:09:29 - Make Post Request to Login Endpoint
01:12:08 - Dynamically render content based on whether user is Authenticated/Logged in.
01:01:14 - Check if user is Authenticated
01:15:32 - Create an Endpoint to Get information about user
01:18:56 - Make Get Request
01:21:00 - Logout Users by making a post request to logout endpoint ( Blacklist refresh Token )
🔗Important Resources and Links:
Django Docs:
Django Rest Framework Docs:
Django Rest Framework Simple JWT Docs:
Axios Docs:
ES7+ React/Redux/React-Native snippets
Github Repo:
🧡 If you have found this content useful consider becoming my Patreon: 💜
#django
#reactjs
#jwt
#fullstack
#authentication
#codingtutorial
#guide
#tutorial
#api
Комментарии