filmov
tv
Multiply Strings - Leetcode 43 - Python
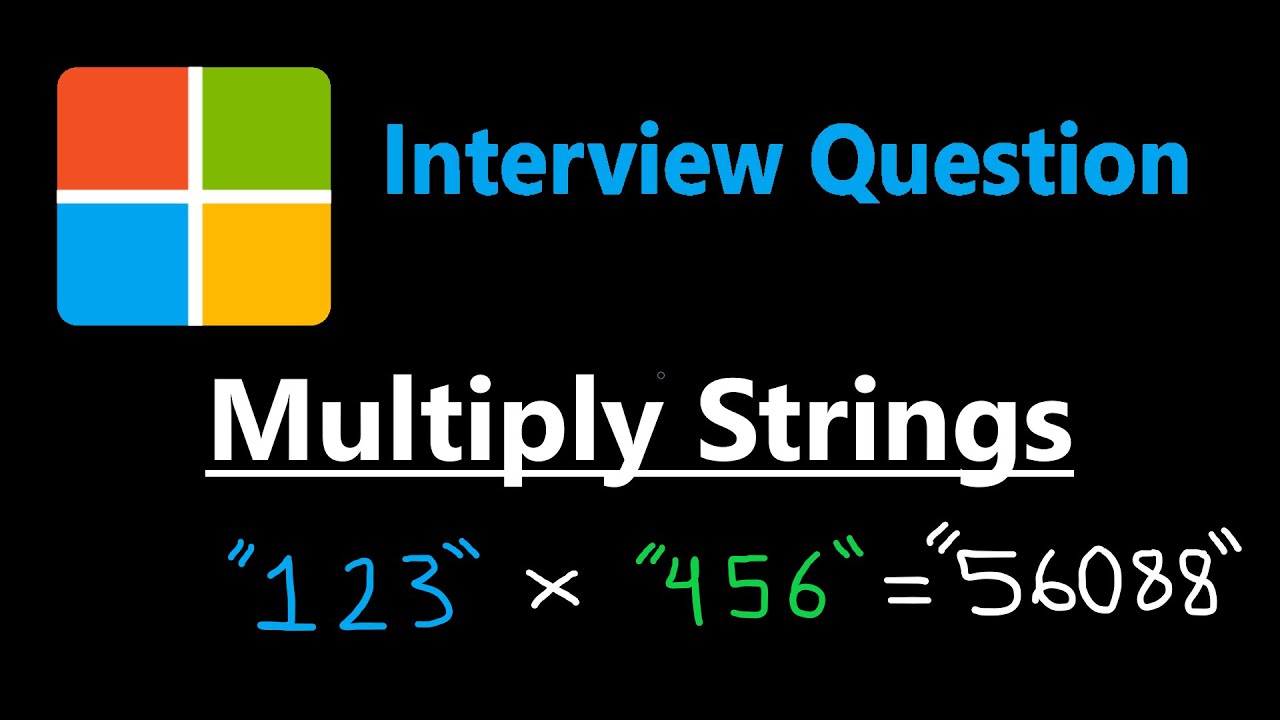
Показать описание
0:00 - Read the problem
1:31 - Drawing Explanation
10:48 - Coding Explanation
leetcode 43
#python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Multiply Strings - Leetcode 43 - Python
leetcode 43. Multiply Strings
LeetCode 43. Multiply Strings Explantion and Solution
Multiply Strings Python Solution - LeetCode #43
Multiply Strings || Leetcode 43 || C++ Solution
Multiply Strings | Leetcode 43 | Live coding session 🔥🔥🔥
LeetCode: 43. Multiply Strings (Visualized)
🔥Leetcode 43 - Multiply Strings - Python | #amazon,#meta,#google 🏆 #leetcode #leetcodesolutions...
LeetCode in C# | 43. Multiply Strings
Multiply Strings | Leetcode 43 Solution in Hindi
Microsoft Coding Interview Question | Leetcode 43 | Multiply Strings
Leet Code 43 : Multiply Strings Problem | Tutort Academy
43. Multiply Strings | Leetcode | Medium | Java | Hindi | Google
leetCode #43 Multiply Strings | JSer - algorithm and JavaScript
43. Multiply Strings - Day 7/30 Leetcode November Challenge
LeetCode 43 | Multiply Strings | Math | Java
Multiply Strings - LeetCode #43 - C++
LeetCode 43 Multiply Strings
43. Multiply Strings (Brute Force, Grade School Multiplication, Karatsuba Algorithm) - LeetCode
43. Multiply Strings | English | Medium
C++ leetcode 43. Multiply Strings
Leetcode Question 43 'Multiply Strings' in Javascript
🔥Leetcode Daily! Leetcode 43 - Multiply Strings - Python | #amazon , #meta, #google 🏆 #shorts #reel...
[Leetcode 43] Multiply Strings
Комментарии