filmov
tv
How to Fix setTimeout Issues in JavaScript Promises
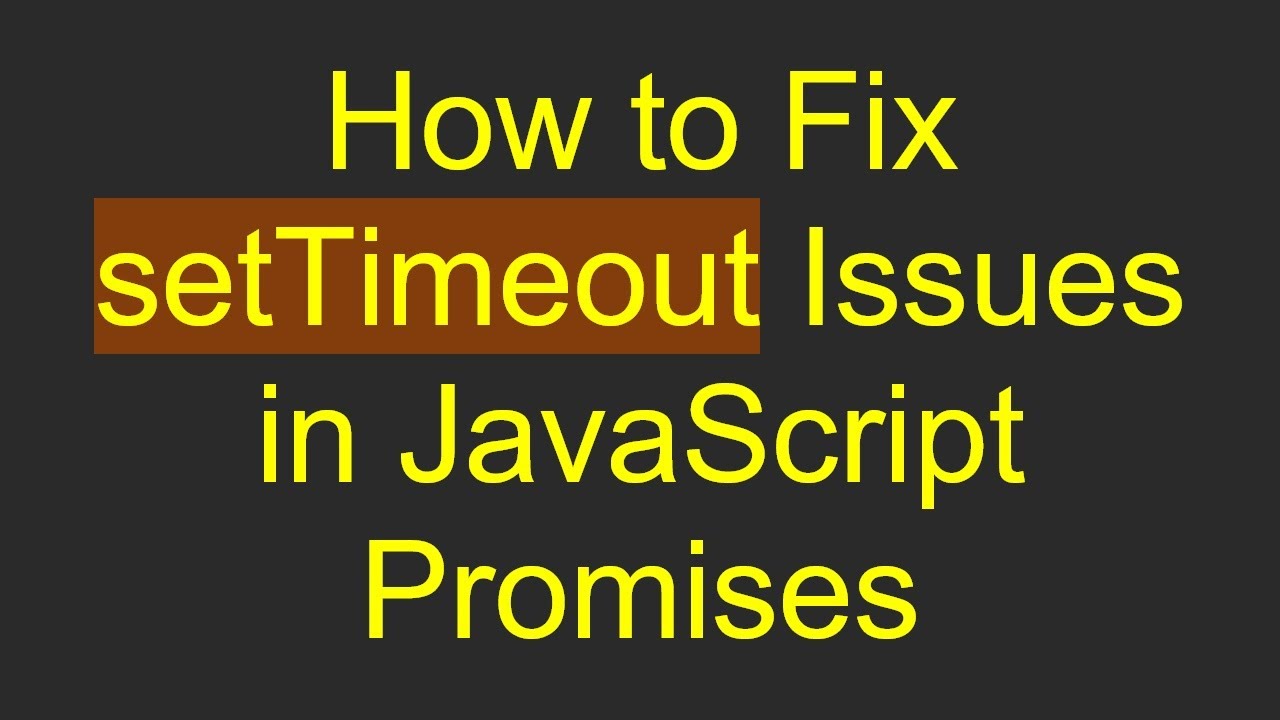
Показать описание
Learn how to properly use `setTimeout` with JavaScript promises to ensure callbacks are executed as intended. Fix common pitfalls that lead to unexpected behavior in your asynchronous code.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: setTimeout is not honored when resolving callback function in a Promise
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Issue: setTimeout with Promises
JavaScript has become a cornerstone of modern web development, especially when it comes to handling asynchronous tasks. However, developers often run into issues when mixing callbacks with promises. One common problem arises with the setTimeout function not behaving as expected when used within promises. If you've ever found your callback functions being executed immediately instead of after a set delay, you're not alone. Today, we'll explore why this happens and how to fix it.
The Problem
If you're trying to create an asynchronous flow that includes scrolling a webpage and invoking a callback after a delay, you might run into this scenario. Let's consider the example code provided in the introduction:
[[See Video to Reveal this Text or Code Snippet]]
In this code, promisePageUp(callback) is called immediately, and its return value is passed to resolve(). This is not the intended behavior, as you want the resolve to be executed after the timeout and not immediately.
The Solution: Passing Function References
To resolve this issue, you need to pass a function reference to setTimeout() rather than executing the function outright. Here's how you can fix the problematic lines of code.
Corrected Code
Replace the following lines of code:
[[See Video to Reveal this Text or Code Snippet]]
with:
[[See Video to Reveal this Text or Code Snippet]]
This allows setTimeout() to call your function later, at the appropriate time.
Tackle the Callback Issue Similarly
You’ll also encounter a similar issue with this line:
[[See Video to Reveal this Text or Code Snippet]]
This should be changed to:
[[See Video to Reveal this Text or Code Snippet]]
Both corrections point to a crucial aspect of using asynchronous functions in JavaScript: make sure to pass a reference to the function rather than executing it immediately.
The Distinction with Scrolling Delay
Interestingly, in your code:
[[See Video to Reveal this Text or Code Snippet]]
This line is correct because the function pageScroll is being passed as a reference, allowing setTimeout() to invoke it later.
A Note on Code Structure
Mixing promises and callbacks can lead to complex and hard-to-maintain code. It's advisable to "promisify" your codebase, where you replace callbacks with promises across the board. Here are a few suggestions for improving your structure:
Use Promises throughout: If you're working with a function that manages asynchronous operations, try to keep your entire codebase consistent by utilizing promises instead of mixing in callbacks.
Create a Promisified setTimeout: For a smoother experience, consider using a promisified version of setTimeout(). This can simplify your code structure and improve readability.
Conclusion
In summary, the setTimeout function should have a reference passed to it, not an immediate function execution. By following the adjustments shared in this post, you can ensure more predictable asynchronous behavior in your JavaScript applications. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: setTimeout is not honored when resolving callback function in a Promise
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Issue: setTimeout with Promises
JavaScript has become a cornerstone of modern web development, especially when it comes to handling asynchronous tasks. However, developers often run into issues when mixing callbacks with promises. One common problem arises with the setTimeout function not behaving as expected when used within promises. If you've ever found your callback functions being executed immediately instead of after a set delay, you're not alone. Today, we'll explore why this happens and how to fix it.
The Problem
If you're trying to create an asynchronous flow that includes scrolling a webpage and invoking a callback after a delay, you might run into this scenario. Let's consider the example code provided in the introduction:
[[See Video to Reveal this Text or Code Snippet]]
In this code, promisePageUp(callback) is called immediately, and its return value is passed to resolve(). This is not the intended behavior, as you want the resolve to be executed after the timeout and not immediately.
The Solution: Passing Function References
To resolve this issue, you need to pass a function reference to setTimeout() rather than executing the function outright. Here's how you can fix the problematic lines of code.
Corrected Code
Replace the following lines of code:
[[See Video to Reveal this Text or Code Snippet]]
with:
[[See Video to Reveal this Text or Code Snippet]]
This allows setTimeout() to call your function later, at the appropriate time.
Tackle the Callback Issue Similarly
You’ll also encounter a similar issue with this line:
[[See Video to Reveal this Text or Code Snippet]]
This should be changed to:
[[See Video to Reveal this Text or Code Snippet]]
Both corrections point to a crucial aspect of using asynchronous functions in JavaScript: make sure to pass a reference to the function rather than executing it immediately.
The Distinction with Scrolling Delay
Interestingly, in your code:
[[See Video to Reveal this Text or Code Snippet]]
This line is correct because the function pageScroll is being passed as a reference, allowing setTimeout() to invoke it later.
A Note on Code Structure
Mixing promises and callbacks can lead to complex and hard-to-maintain code. It's advisable to "promisify" your codebase, where you replace callbacks with promises across the board. Here are a few suggestions for improving your structure:
Use Promises throughout: If you're working with a function that manages asynchronous operations, try to keep your entire codebase consistent by utilizing promises instead of mixing in callbacks.
Create a Promisified setTimeout: For a smoother experience, consider using a promisified version of setTimeout(). This can simplify your code structure and improve readability.
Conclusion
In summary, the setTimeout function should have a reference passed to it, not an immediate function execution. By following the adjustments shared in this post, you can ensure more predictable asynchronous behavior in your JavaScript applications. Happy coding!