filmov
tv
Calling a JavaScript function in multiple ways | #javascript #javascriptshorts #hacks
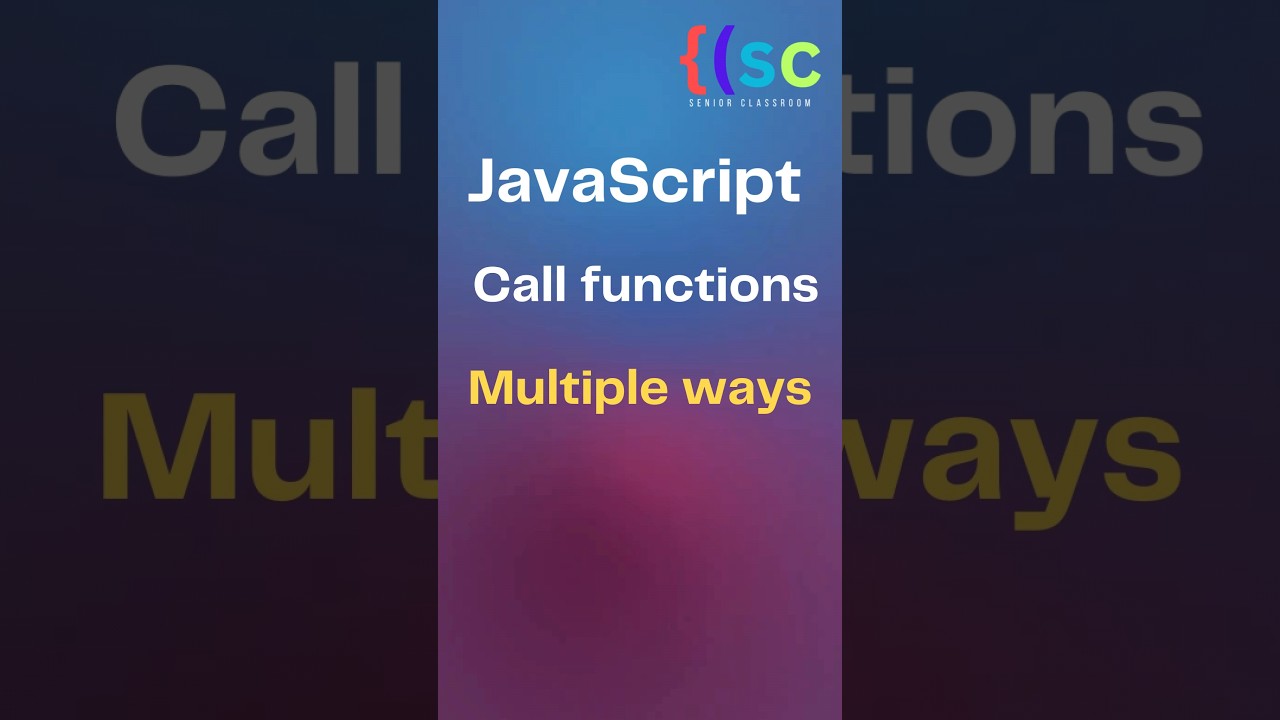
Показать описание
Calling a JavaScript function in multiple ways is a common practice in web development, enhancing the flexibility and reusability of the code. Here are several methods to invoke a JavaScript function:
Direct Call:
---------------
The simplest way to call a function is directly by its name followed by parentheses.
function greet() {
}
greet(); // Output: Hello, World!
Event Handlers:
-------------
Functions can be called in response to events, such as clicks or form submissions.
Inline HTML Event Attributes:
------------
Functions can be called directly from HTML using event attributes.
Using call and apply Methods:
-------------
The call and apply methods can invoke functions with a specified this value and arguments.
function introduce(name, age) {
}
Using bind Method:
-----------
The bind method creates a new function that, when called, has its this keyword set to the provided value.
greetAlice(); // Output: Hello, World!
As a Method in an Object:
--------------
Functions can be methods within objects and can be called using the dot notation.
const person = {
name: 'John',
greet: function() {
}
};
Using the new Operator:
---------------
Functions can be called as constructors using the new operator.
function Person(name) {
}
const john = new Person('John');
Immediately Invoked Function Expressions (IIFE):
--------------------------
Functions can be called immediately after they are defined.
(function() {
})(); // Output: This function runs immediately.
Using setTimeout and setInterval:
------------------
Functions can be called after a delay or at regular intervals using setTimeout and setInterval.
setTimeout(greet, 1000); // Calls greet after 1 second
setInterval(greet, 2000); // Calls greet every 2 seconds
By leveraging these different methods, developers can optimize how and when functions are called, leading to more dynamic and responsive web applications.
Direct Call:
---------------
The simplest way to call a function is directly by its name followed by parentheses.
function greet() {
}
greet(); // Output: Hello, World!
Event Handlers:
-------------
Functions can be called in response to events, such as clicks or form submissions.
Inline HTML Event Attributes:
------------
Functions can be called directly from HTML using event attributes.
Using call and apply Methods:
-------------
The call and apply methods can invoke functions with a specified this value and arguments.
function introduce(name, age) {
}
Using bind Method:
-----------
The bind method creates a new function that, when called, has its this keyword set to the provided value.
greetAlice(); // Output: Hello, World!
As a Method in an Object:
--------------
Functions can be methods within objects and can be called using the dot notation.
const person = {
name: 'John',
greet: function() {
}
};
Using the new Operator:
---------------
Functions can be called as constructors using the new operator.
function Person(name) {
}
const john = new Person('John');
Immediately Invoked Function Expressions (IIFE):
--------------------------
Functions can be called immediately after they are defined.
(function() {
})(); // Output: This function runs immediately.
Using setTimeout and setInterval:
------------------
Functions can be called after a delay or at regular intervals using setTimeout and setInterval.
setTimeout(greet, 1000); // Calls greet after 1 second
setInterval(greet, 2000); // Calls greet every 2 seconds
By leveraging these different methods, developers can optimize how and when functions are called, leading to more dynamic and responsive web applications.