filmov
tv
Creating a Reusable Function in JavaScript: Optimize Your Code!
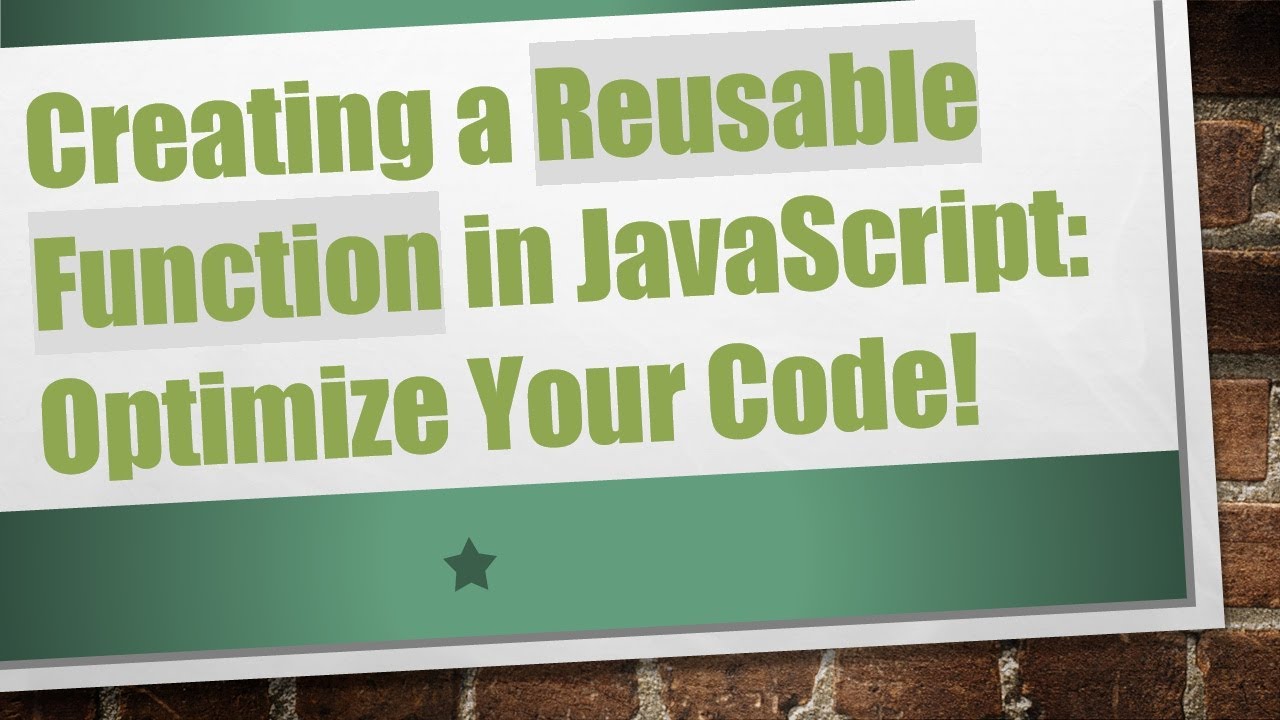
Показать описание
Learn how to create a reusable function in JavaScript to avoid code duplication and enhance maintainability.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: javascript reusable function
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Reusable Function in JavaScript: Optimize Your Code!
When programming, especially in JavaScript, one common challenge developers face is code duplication. In particular, when functions perform similar actions, it makes sense to refactor them into a single reusable function. This article will explore how to achieve this by using a practical example involving selection functionality in user interfaces.
The Problem: Code Duplication in Functions
Suppose you have two functions that are nearly identical but are targeting different state variables within a React component. Here’s a look at those functions:
Function One:
[[See Video to Reveal this Text or Code Snippet]]
Function Two:
[[See Video to Reveal this Text or Code Snippet]]
As you can see, both functions essentially perform the same logic: they check if an id is already selected and either add or remove it from the respective array. This redundancy can lead to maintenance issues and scattered logic throughout your codebase.
The Solution: A Reusable Function
To optimize the code, you can create a single function that can handle both cases. The key is to pass a parameter that specifies which state variable to manipulate. Here’s how:
The Refactored Function:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Refactored Function
Function Parameters: The onChange function now accepts two parameters - id (the identifier of the item to be selected or deselected) and key (which string indicates which state variable to use, such as 'selectedDevice' or 'selectedSection').
State Update: The same splice and push logic is applied, ensuring functionality remains consistent with the original implementations.
Using the Refactored Function: An Example
In your component, you can now call this function for both cases like so:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of Reusable Functions
Reduced Code Duplication: By consolidating similar logic, you can streamline your codebase, making it easier to read and maintain.
Increased Flexibility: A single function can handle multiple cases, making it simpler to implement changes in the future.
Simplified Testing: With a single function to test, debugging becomes straightforward.
Conclusion
Creating a reusable function in JavaScript not only saves time but also contributes to clean, maintainable code. The example we've discussed demonstrates a practical scenario of how to refactor repeated code into a single useable function, enhancing your programming efficiency and effectiveness.
So the next time you find yourself repeating code, ask: "Can I make this reusable?" Your future self will thank you!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: javascript reusable function
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Reusable Function in JavaScript: Optimize Your Code!
When programming, especially in JavaScript, one common challenge developers face is code duplication. In particular, when functions perform similar actions, it makes sense to refactor them into a single reusable function. This article will explore how to achieve this by using a practical example involving selection functionality in user interfaces.
The Problem: Code Duplication in Functions
Suppose you have two functions that are nearly identical but are targeting different state variables within a React component. Here’s a look at those functions:
Function One:
[[See Video to Reveal this Text or Code Snippet]]
Function Two:
[[See Video to Reveal this Text or Code Snippet]]
As you can see, both functions essentially perform the same logic: they check if an id is already selected and either add or remove it from the respective array. This redundancy can lead to maintenance issues and scattered logic throughout your codebase.
The Solution: A Reusable Function
To optimize the code, you can create a single function that can handle both cases. The key is to pass a parameter that specifies which state variable to manipulate. Here’s how:
The Refactored Function:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Refactored Function
Function Parameters: The onChange function now accepts two parameters - id (the identifier of the item to be selected or deselected) and key (which string indicates which state variable to use, such as 'selectedDevice' or 'selectedSection').
State Update: The same splice and push logic is applied, ensuring functionality remains consistent with the original implementations.
Using the Refactored Function: An Example
In your component, you can now call this function for both cases like so:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of Reusable Functions
Reduced Code Duplication: By consolidating similar logic, you can streamline your codebase, making it easier to read and maintain.
Increased Flexibility: A single function can handle multiple cases, making it simpler to implement changes in the future.
Simplified Testing: With a single function to test, debugging becomes straightforward.
Conclusion
Creating a reusable function in JavaScript not only saves time but also contributes to clean, maintainable code. The example we've discussed demonstrates a practical scenario of how to refactor repeated code into a single useable function, enhancing your programming efficiency and effectiveness.
So the next time you find yourself repeating code, ask: "Can I make this reusable?" Your future self will thank you!