filmov
tv
Interview Question: Max Stack
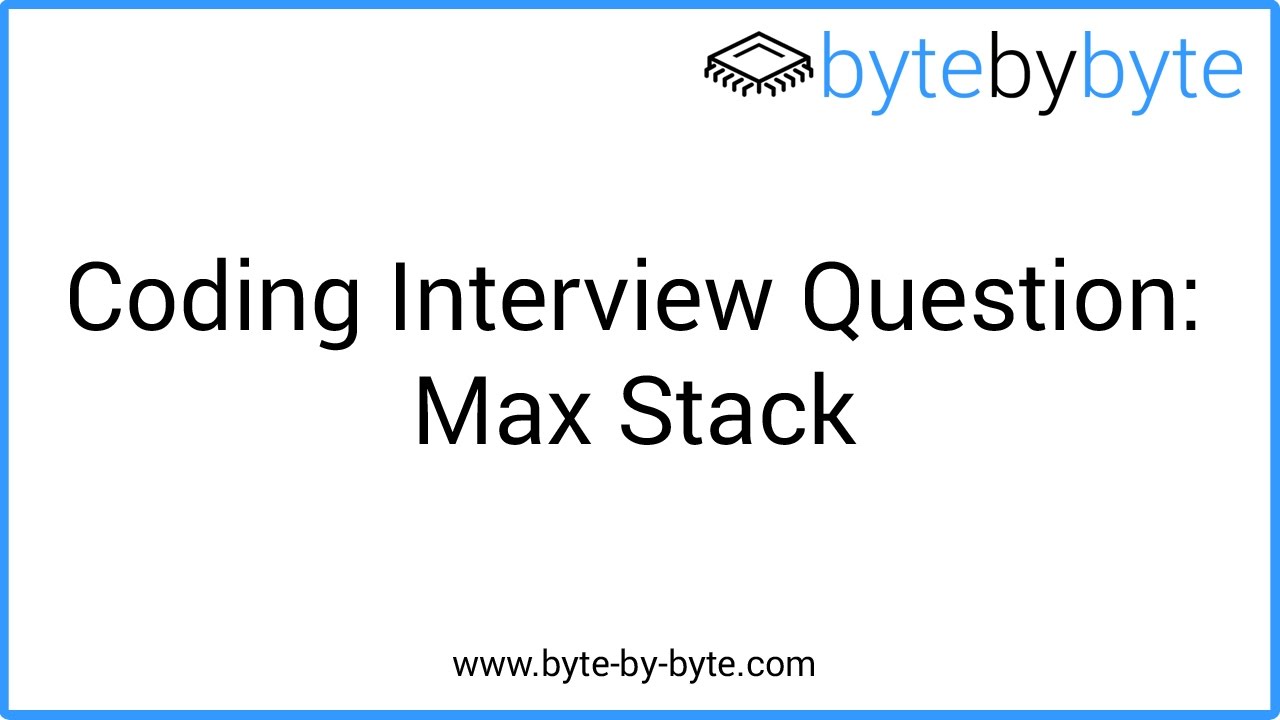
Показать описание
In this video, I show how to create a stack that with push(), pop(), and max() functions.
Do you have a big interview coming up with Google or Facebook? Do you want to ace your coding interviews once and for all? If so, Byte by Byte has everything that you need to go to get your dream job. We've helped thousands of students improve their interviewing and we can help you too.
You can also find me on
Interview Question: Max Stack
Max Stack: 716 - leetcode premium LinkedIn interview question
Interview Question: N Stacks
Stack With Max | Elements of Programming Interviews
Coding Interview | Stack Questions
Leetcode 716. Max Stack | Python Solution | LinkedIn Interview Question
I gave 127 interviews. Top 5 Algorithms they asked me.
Design Min Stack - Amazon Interview Question - Leetcode 155 - Python
Interview Question: Stack from Queues
How I Got Good at Coding Interviews
Adobe Coding Interview Question - Max Depth of Binary Tree (Height) - Leetcode 104
Whiteboard Coding Interviews: 6 Steps to Solve Any Problem
Maximum Frequency Stack - Leetcode 895 - Python
Google Coding Interview Question - Validate Stack Sequences (LeetCode)
Top 5 Data Structures they asked me in 127 interviews
Elon Musk Laughs at the Idea of Getting a PhD... and Explains How to Actually Be Useful!
Microsoft Interview Question - MinStack in O(1)
Developer Last Expression 😂 #shorts #developer #ytshorts #uiux #python #flutterdevelopment
8 patterns to solve 80% Leetcode problems
3 Tips I’ve learned after 2000 hours of Leetcode
Implement A Max Stack - A Stack With A .max() API (Similar To 'Min Stack' on LeetCode)
Apple Coding Interview Question! | Trapping Rain Water - Leetcode 42
Interview Question: Reverse Stack
Amazon Coding Interview Question - Min Stack (LeetCode)
Комментарии