filmov
tv
Loop Inside React JSX
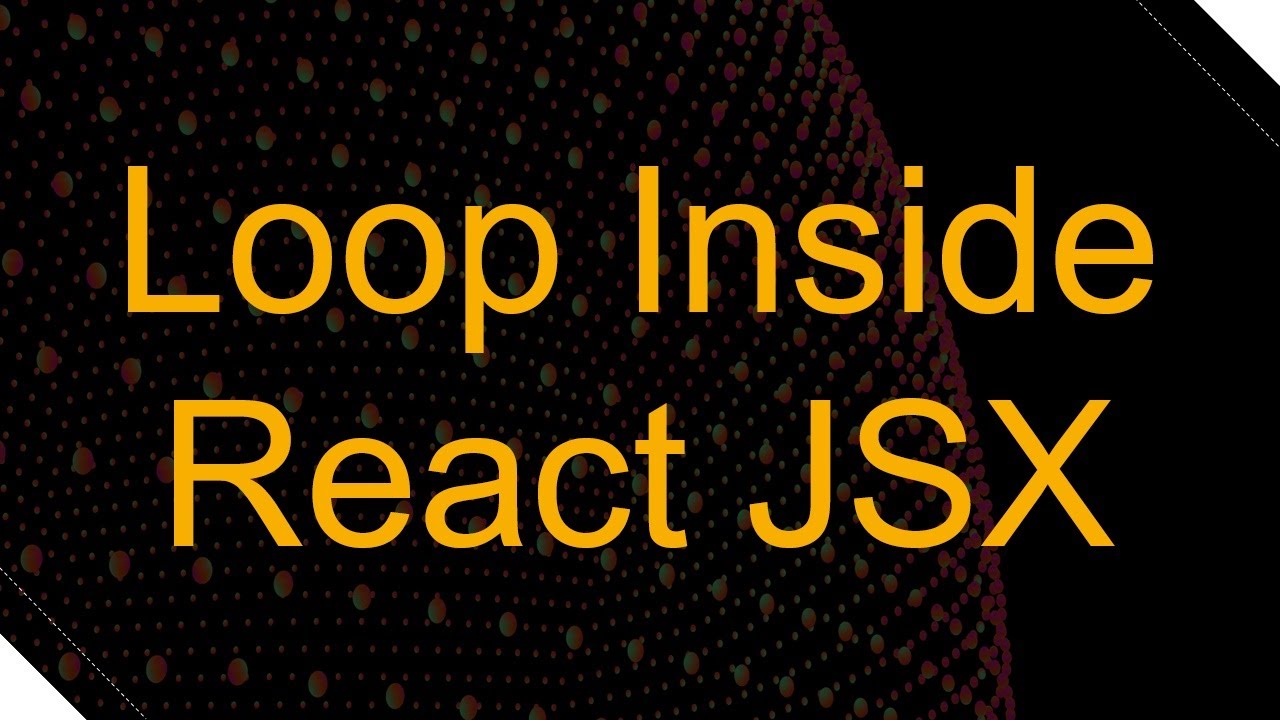
Показать описание
Summary: Learn how to effectively use loops inside React JSX to render lists of elements dynamically and efficiently in your React applications.
---
Loop Inside React JSX
React, a popular JavaScript library for building user interfaces, often requires developers to render lists of elements dynamically. This is commonly achieved by using loops within JSX, React's syntax extension for JavaScript. Understanding how to effectively use loops in JSX is crucial for creating efficient and maintainable React applications.
Why Loop in JSX?
When working with arrays of data, such as items in a list, rendering each item individually would be cumbersome and inefficient. By using loops, we can generate multiple JSX elements dynamically, making our code more concise and easier to manage.
Common Methods for Looping in JSX
Using map()
The most common and idiomatic way to loop through data in React is by using the map() method. This method is called on arrays to create a new array by applying a function to each element.
Here is a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, items is an array passed as a prop to the ItemList component. The map() method iterates over each item in the array, and for each item, it returns a <li> element. The key attribute is used to give each element a unique identifier, which helps React optimize rendering.
Using forEach()
While less common in JSX, the forEach() method can also be used to loop through data. However, since forEach() does not return a new array, you need to manually push the elements to an array:
[[See Video to Reveal this Text or Code Snippet]]
Using for Loops
Traditional for loops can also be used, although they are less elegant compared to map(). This approach is more verbose and generally not recommended unless necessary:
[[See Video to Reveal this Text or Code Snippet]]
Best Practices
Always Use Keys: When rendering lists, always provide a unique key for each element. This helps React identify which items have changed, are added, or are removed, optimizing the rendering process.
Prefer map(): The map() method is concise and readable, making it the preferred choice for looping in JSX.
Avoid Index as Key: If possible, avoid using the array index as the key, especially if the list can change. Using unique identifiers from the data itself is a better practice.
Conclusion
Looping inside React JSX is a fundamental technique for rendering dynamic lists efficiently. By utilizing methods like map(), you can keep your code clean and maintainable. Remember to use unique keys and adhere to best practices to ensure optimal performance in your React applications.
---
Loop Inside React JSX
React, a popular JavaScript library for building user interfaces, often requires developers to render lists of elements dynamically. This is commonly achieved by using loops within JSX, React's syntax extension for JavaScript. Understanding how to effectively use loops in JSX is crucial for creating efficient and maintainable React applications.
Why Loop in JSX?
When working with arrays of data, such as items in a list, rendering each item individually would be cumbersome and inefficient. By using loops, we can generate multiple JSX elements dynamically, making our code more concise and easier to manage.
Common Methods for Looping in JSX
Using map()
The most common and idiomatic way to loop through data in React is by using the map() method. This method is called on arrays to create a new array by applying a function to each element.
Here is a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, items is an array passed as a prop to the ItemList component. The map() method iterates over each item in the array, and for each item, it returns a <li> element. The key attribute is used to give each element a unique identifier, which helps React optimize rendering.
Using forEach()
While less common in JSX, the forEach() method can also be used to loop through data. However, since forEach() does not return a new array, you need to manually push the elements to an array:
[[See Video to Reveal this Text or Code Snippet]]
Using for Loops
Traditional for loops can also be used, although they are less elegant compared to map(). This approach is more verbose and generally not recommended unless necessary:
[[See Video to Reveal this Text or Code Snippet]]
Best Practices
Always Use Keys: When rendering lists, always provide a unique key for each element. This helps React identify which items have changed, are added, or are removed, optimizing the rendering process.
Prefer map(): The map() method is concise and readable, making it the preferred choice for looping in JSX.
Avoid Index as Key: If possible, avoid using the array index as the key, especially if the list can change. Using unique identifiers from the data itself is a better practice.
Conclusion
Looping inside React JSX is a fundamental technique for rendering dynamic lists efficiently. By utilizing methods like map(), you can keep your code clean and maintainable. Remember to use unique keys and adhere to best practices to ensure optimal performance in your React applications.