filmov
tv
Build Array from Permutation | Follow Up Qn | Detailed Explanation | Leetcode 1920 |codestorywithMIK
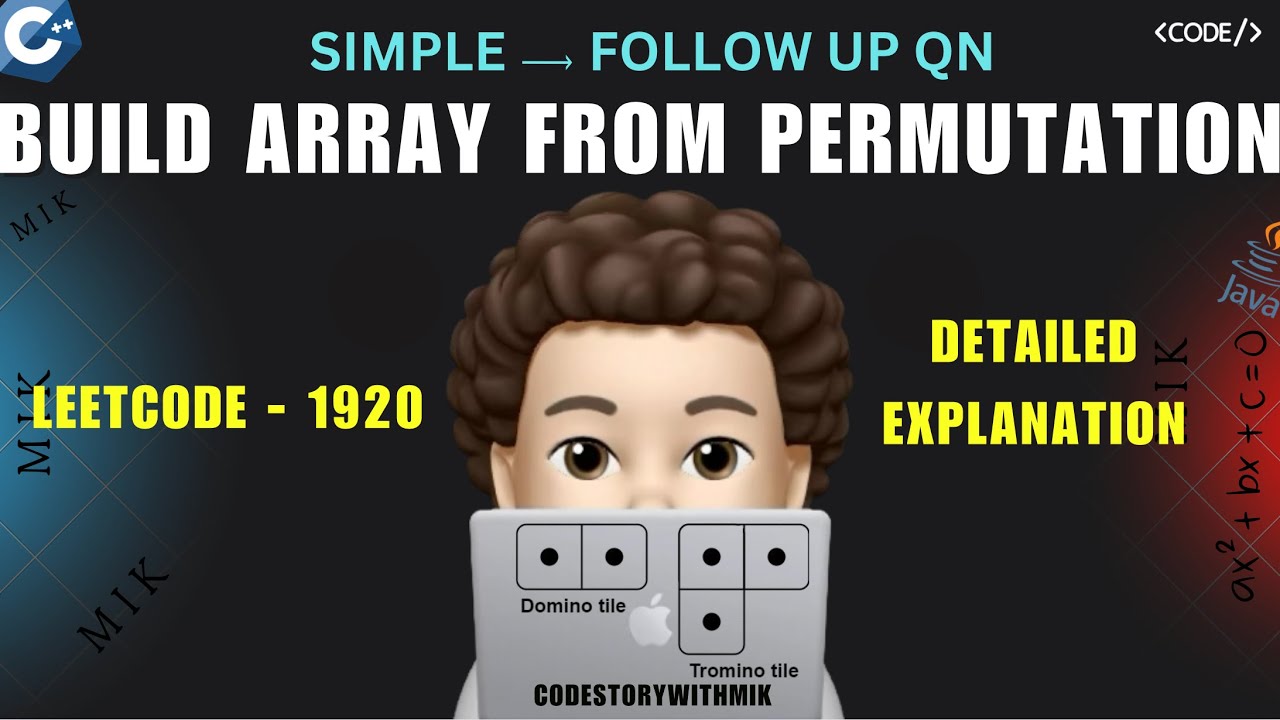
Показать описание
Hi Everyone, this is the 85th video of our Playlist "Leetcode Easy".
Now we will be solving a good but easy Problem - Build Array from Permutation | Follow Up Qn | Detailed Explanation | Leetcode 1920 | codestorywithMIK
I will explain it in full detail so that it becomes easy to understand. We will find the reason behind everything so that we understand why we did what we did.
And we will do a complete dry run.
Problem Name : Build Array from Permutation | Follow Up Qn | Detailed Explanation | Leetcode 1920 | codestorywithMIK
Company Tags : will update later
Code Github(C++ & JAVA) - will update later tonight
╔═╦╗╔╦╗╔═╦═╦╦╦╦╗╔═╗
║╚╣║║║╚╣╚╣╔╣╔╣║╚╣═╣
╠╗║╚╝║║╠╗║╚╣║║║║║═╣
╚═╩══╩═╩═╩═╩╝╚╩═╩═╝
Video Summary :
The problem requires constructing an array such that ans[i] = nums[nums[i]].
Instead of using extra space, the solution encodes both old and new values into each element using the formula:
nums[i] = nums[i] + n * (nums[nums[i]] % n), where % n ensures correct value extraction.
In the second pass, nums[i] is divided by n to retrieve only the newly encoded value, achieving an in-place transformation.
This approach uses mathematical encoding to meet the O(1) space constraint.
✨ Timelines✨
00:00 - Introduction
0:19 - Motivation
0:49 - Problem Explanation
3:53 - Simple Approach
4:41 - Follow Up Qn
8:20 - Detailed with example
21:50 - Story To Code
24:19 - Coding it up
#MIK #mik #Mik
#coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge #leetcodequestions #leetcodechallenge #hindi #india #coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge #leetcodequestions #leetcodechallenge #hindi #india #hindiexplanation #hindiexplained #easyexplaination #interview#interviewtips #interviewpreparation #interview_ds_algo #hinglish #github #design #data #google #video #instagram #facebook #leetcode #computerscience #leetcodesolutions #leetcodequestionandanswers #code #learning #dsalgo #dsa #coding #programming #100daysofcode #developers #techjobs #datastructures #algorithms #webdevelopment #softwareengineering #computerscience #pythoncoding #codinglife #coderlife #javascript #datascience #leetcode #leetcodesolutions #leetcodedailychallenge #codinginterview #interviewprep #technicalinterview #interviewtips #interviewquestions #codingchallenges #interviewready #dsa #hindi #india #hindicoding #hindiprogramming #hindiexplanation #hindidevelopers #hinditech #hindilearning #helpajobseeker #jobseekers #jobsearchtips #careergoals #careerdevelopment #jobhunt #jobinterview #github #designthinking #learningtogether #growthmindset #digitalcontent #techcontent #socialmediagrowth #contentcreation #instagramreels #videomarketing #codestorywithmik #codestorywithmick #codestorywithmikc #codestorywitmik #codestorywthmik #codstorywithmik #codestorywihmik #codestorywithmiik #codeistorywithmik #codestorywithmk #codestorywitmick #codestorymik #codestorwithmik
Комментарии