filmov
tv
PROBLEM SET 1: DEEP THOUGHT | SOLUTION (CS50 PYTHON)
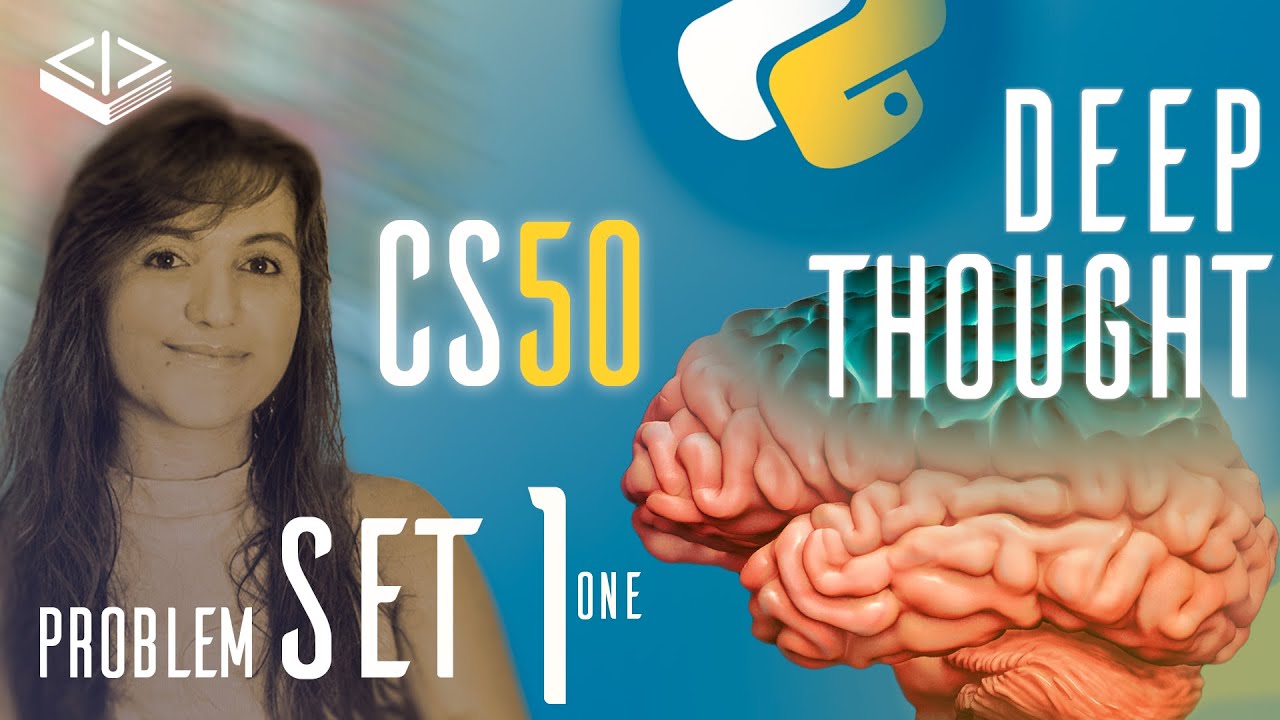
Показать описание
––– DISCLAIMER –––
The following videos are for educational purposes only. Cheating or any other activities are highly discouraged!! Using another person’s code breaks the academic honesty guidelines. This solution is for those who have finished the problem sets and want to watch for educational purposes, learning experience, and exploring alternative ways to approach problems and is NOT meant for those actively doing the problem sets. All problem sets presented in this video are owned by Harvard University.
–––
PROBLEM SET 1: DEEP THOUGHT | SOLUTION (CS50 PYTHON)
Deep Thought -Problem Set 1 (CS50's Introduction to Programming with Python)
PROBLEM SET 1 Deep Thought Solution CS50P
CS50P PSet1 ~ Deep Thought | Solution
SOLVE PROBLEM SET 1 CS50 PROGRAMMING WITH PYTHON: DEEP THOUGHT IN 2024 (FREE COURSE)
CS50 Python | Week 1 | Conditionals | Problem Set 1 | Deep Thought
Deep Thoughts - CS50P Problem Set 1
HarvardX CS50 Python: Deep Thought (PSET 1)
PROBLEM SET 1: DEEP THOUGHT | SOLUTION | Pythonista_geek | Codewithme | #CS50P
CS50 Python 2023 | Deep Thought - Walkthrough & Solution (Problem Set 0, Week 0)
CS50 Week_1 Deep Thought | Problem Set 1 | Solution | By Theven thugs
Harvard CS50p Problem Set 1 Deep Thought Çözümü
CS50P - Python program for deep thought - Problem set 1
Problem Set 1 Deep Thought Solution CS50 Introduction to Programming with Python
CS50P | Week 01 | Problem 01 | Deep Thought
PROBLEM SET 1: MEAL TIME | SOLUTION (CS50 PYTHON)
CS50 Python | Week 1 | Conditionals | Problem Set 1 | Home Federal Savings Bank
CS50P - Problem Set 1
Answering Your Questions + Solving CS50 Python: Tip Calculator and Deep Thought
Walkthrough of CS50 Python Problem Set 1
CS50P - Problem Set 1 - Meal Time
File Extensions -Problem Set 1 (CS50's Introduction to Programming with Python)
Solving CS50-Python Problem Set 0, 1 | Day 1 Highlights
PROBLEM SET 1: FILE EXTENSIONS | SOLUTION (CS50 PYTHON)
Комментарии