filmov
tv
Binary Search Array 2022 (Step-By-Step Breakdown)
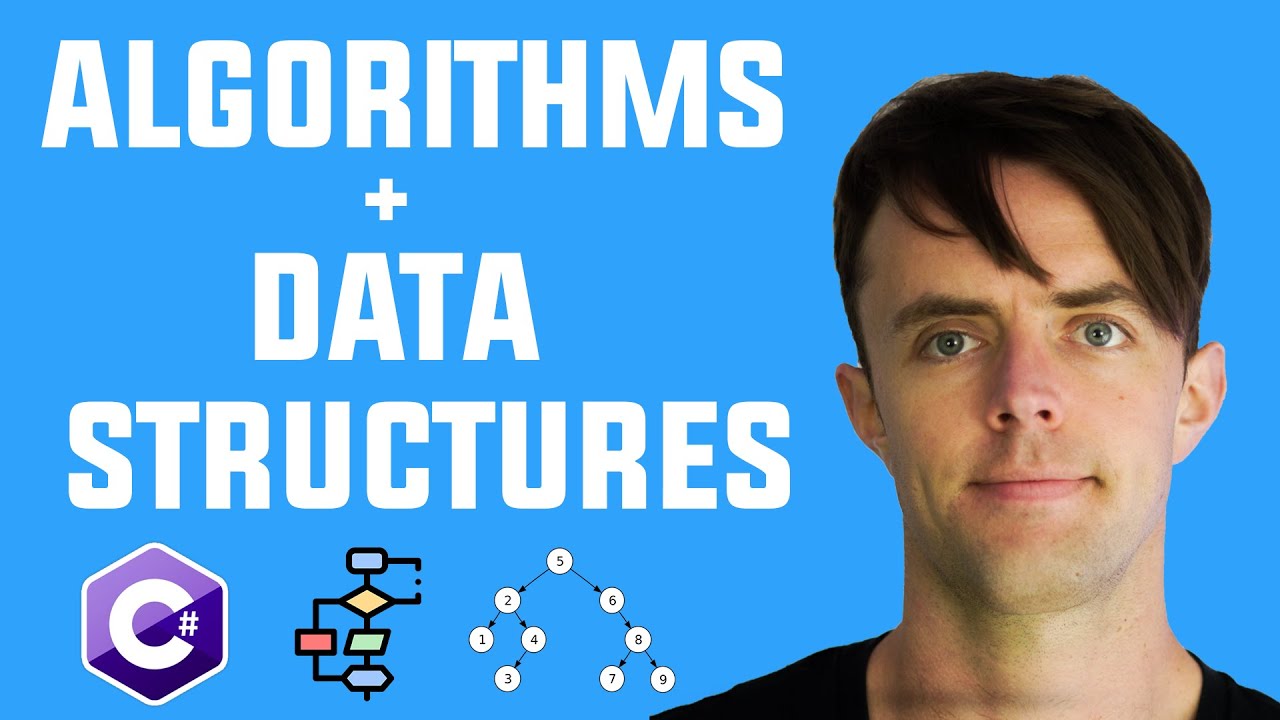
Показать описание
Binary Search Array 2022 (Step-By-Step Breakdown)
Binary Search Array 2022 (Step-By-Step Breakdown)
#9. Search Element In Array Using Binary Search - Step By Step Logic Explained
Linear Search Array C# 2022 (Step-By-Step Breakdown)
Binary Search Algorithm in 100 Seconds
Binary Search Algorithm in C# - Learn to Search Collections efficiently!
How to Do a Binary Search in C# (Simple)
Binary & Ternary Search from scratch (+ Minimum Cost to Make Array Equal)
Intro To Trees + Binary Search Tree (2022) Step-By-Step Breakdown
JavaScript Algorithms - 17 - Binary Search Solution
Learn Binary Search with Visualizer | JavaScript
Binary Search in C++ and Java | Recursively and Iteratively | DSA-One Course #22 | Anuj Bhaiya
BINARY SEARCH USING C
Binary Search | Leetcode 704 | Array | Binary Search
The 3 Levels of Binary Search
3 Binary Search on Reverse Sorted Array
Searching an Element in Array using Binary Search.
Linear Search VS Binary Search💥 | Step By Step Explanation with Animation✨
Binary Search in 12 seconds #shorts
Binary Search #animation
2. Binary Search Code | Searching an element in sorted array | Understand Basics 🚀🚀
Binary Search Program (Modified)
When to use '=' in binary search condition?
Binary Search Code In Python.. 😘😘.#like #subscriber #youtubeshorts #sub4sub #youtubeislife #viral...
Binary Search in array #shorts
Комментарии