filmov
tv
Distinct Subsequences - Dynamic Programming - Leetcode 115 - Python
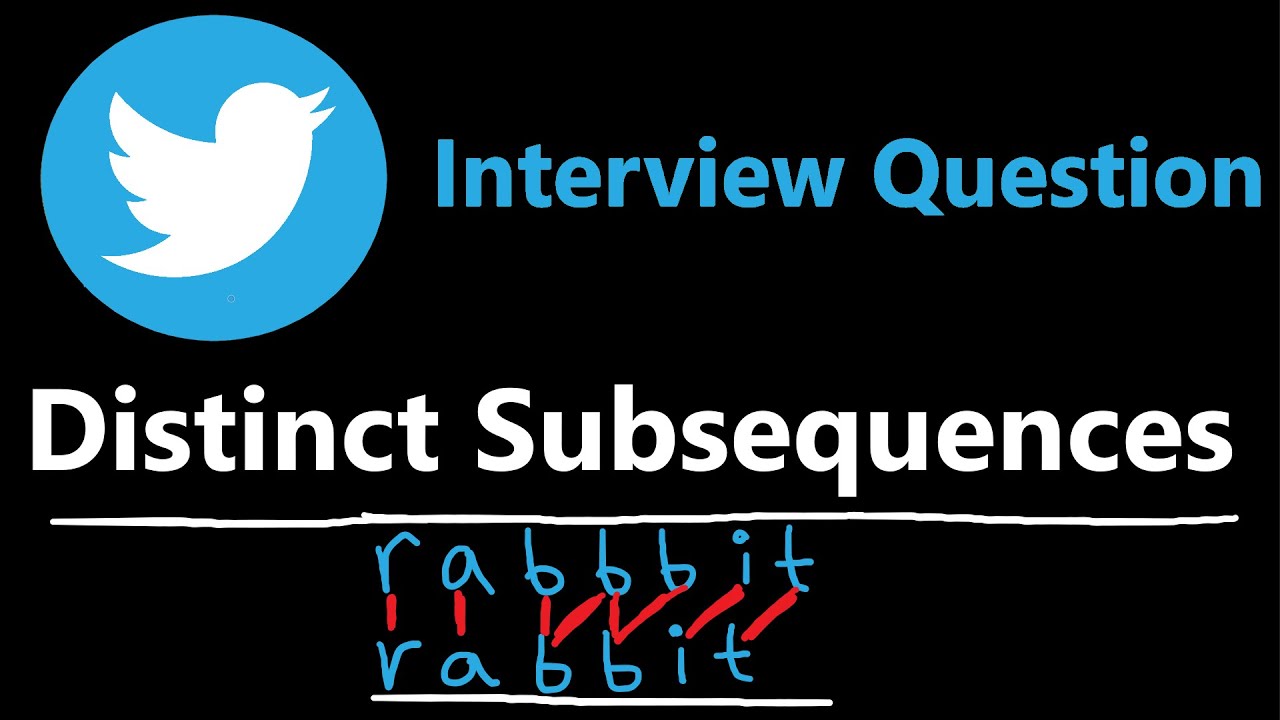
Показать описание
0:00 - Read the problem
1:05 - Drawing Explanation
9:00 - Coding Explanation
leetcode 115
#twitter #interview #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Distinct Subsequences - Dynamic Programming - Leetcode 115 - Python
DP 32. Distinct Subsequences | 1D Array Optimisation Technique 🔥
LeetCode 115. Distinct Subsequences
21DC: (D20P2) Distinct Subsequences
[Java] Leetcode 115. Distinct Subsequences [DP Subsequence #5]
Leetcode - Distinct Subsequences (Python)
LeetCode 115 Distinct Subsequences Explanation and Solution
Count Distinct Subsequences Dynamic Programming | Leetcode Hard Solution
LeetCode 115. Distinct Subsequences (Hard) | Dynamic Programming | C++
leetcode 115 Distinct Subsequences
Distinct subsequences / Distinct occurrences - Dynamic programming
Leetcode 115 Distinct Subsequences
115. Distinct Subsequences | LEETCODE HARD | DYNAMIC PROGRAMMING | CODE EXPLAINER
Distinct Subsequences | Leetcode 115 | Live coding session
Leetcode: 115 | Distinct Subsequences | Dynamic Programming
115. Distinct Subsequences (Leetcode Hard)
115. Distinct Subsequences (Dynamic Programming, Recursion with Memoization) - LeetCode
Distinct Subsequences (LeetCode)
Distinct Subsequences
115. Distinct Subsequences - Day 19/30 Leetcode September Challenge
940. Distinct Subsequences II (Leetcode Hard)
Distinct Subsequences | Detailed Explanation: Intuitions & Observations
LeetCode 940. Distinct Subsequences II (Hard) | Dynamic Programming | C++
Distinct Subsequences - LeetCode #115 - C++
Комментарии