filmov
tv
5 - Binary Search Algorithm Code in C#
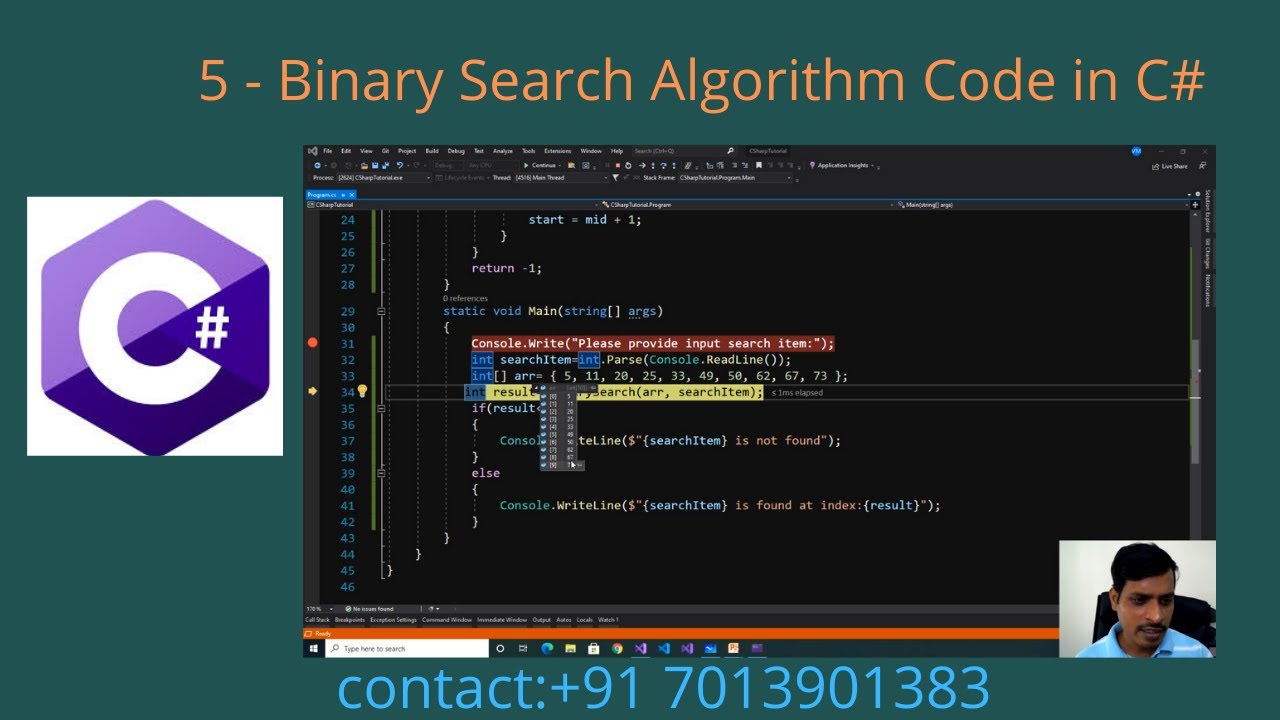
Показать описание
Binary search, also known as half-interval search, logarithmic search, or binary chop, is a search algorithm that finds the position of a target value within a sorted array.
Binary search begins by comparing an element in the middle of the array with the target value.
If the target value matches the element, its position in the array is returned. If the target value is less than the element, the search continues in the lower half of the array.
If the target value is greater than the element, the search continues in the upper half of the array.
By doing this, the algorithm eliminates the half in which the target value cannot lie in each iteration.
We have discussed Binary Search algorithm code in C# in this video but logic is common for any programming language.
Time Complexity:
Best Case: O(1)
Average Case: O(log n)
Worst case: O(log n)
Space Complexity: O(1)
Binary search begins by comparing an element in the middle of the array with the target value.
If the target value matches the element, its position in the array is returned. If the target value is less than the element, the search continues in the lower half of the array.
If the target value is greater than the element, the search continues in the upper half of the array.
By doing this, the algorithm eliminates the half in which the target value cannot lie in each iteration.
We have discussed Binary Search algorithm code in C# in this video but logic is common for any programming language.
Time Complexity:
Best Case: O(1)
Average Case: O(log n)
Worst case: O(log n)
Space Complexity: O(1)
Binary search in 4 minutes
Binary Search Algorithm Explained (Full Code Included) - Python Algorithms Series for Beginners
Binary Search Algorithm - Simply Explained
Linear search vs Binary search
3 Simple Steps for Solving Any Binary Search Problem
Binary Search Algorithm - Computerphile
Algorithms: Binary Search
Introduction to Binary Search (Data Structures & Algorithms #10)
Pre GATE 2025 Exam Solutions: Data Science & Artificial Intelligence | Detailed Solutions|ACE On...
Binary Search in 3 min (Python)
Binary Search Algorithm - White Board Animation Based Explanation,Java Code Walkthrough
[Asymptotics2, Video 5] Binary Search Intuitive
Introduction to Binary Search
7.2 What is Binary Search | Binary Search Algorithm with example | Data Structures Tutorials
Binary Search examples | Successful Search | DAA | Lec-13 | Bhanu Priya
Binary Search - Algorithm and Pseudo-code
5 - Binary Search Algorithm Code in Python
2.6.2 Binary Search Recursive Method
Learn Binary Search in 10 minutes 🪓
Binary Search (Sorted Array) - O(log n) [ Best EXPLAINATION Algorithm]
Analysis of Binary Search Algorithm | Time complexity of Binary Search Algorithm | O(1) | O(log n)
Let's understand Binary Search #binarysearch #beginners #included #python #project #raushanran...
#69 Python Tutorial for Beginners | Binary Search Using Python
The Binary Search Technique You NEED to KNOW!
Комментарии