filmov
tv
Python Tkinter Tutorial | Weapon Wheel Part 2
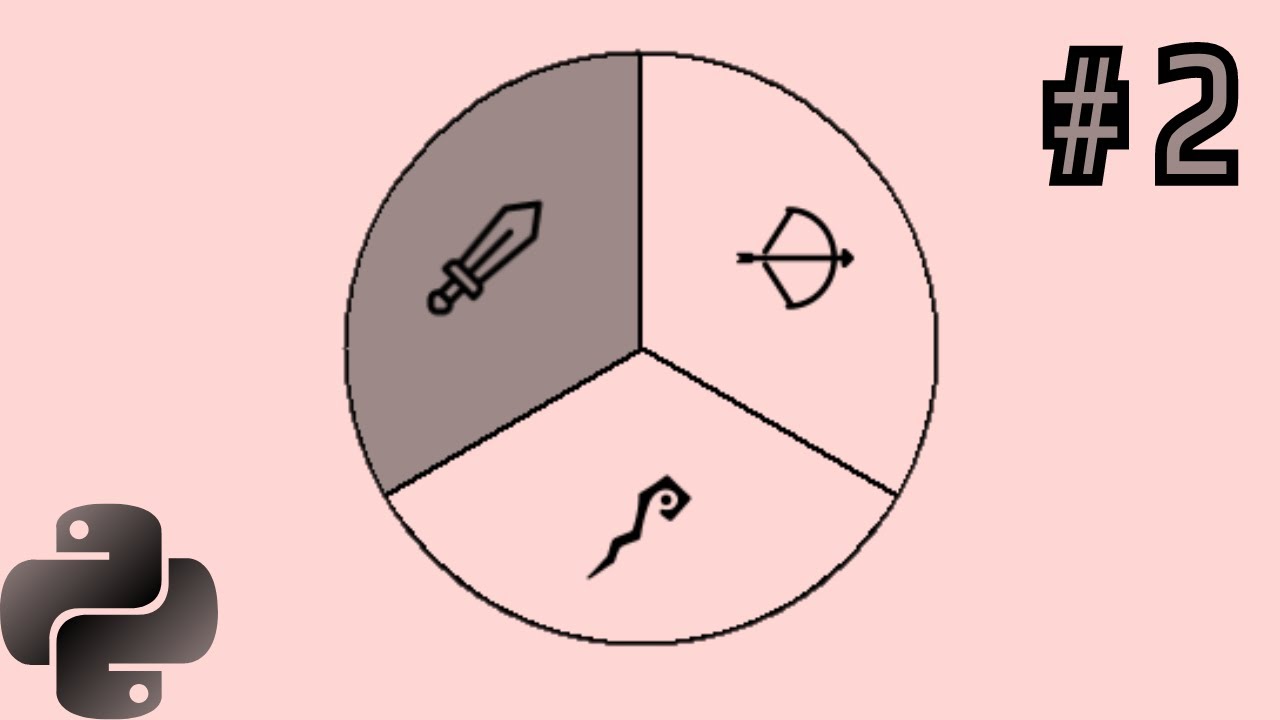
Показать описание
In this video we continue working on our weapon wheel in Python Tkinter! We add a hover effect and display the currently selected weapon.
Python Tkinter | Project Tutorials
Python Tkinter | Widget Tutorials
TkDocs
TkDocs | Canvas
Python Tkinter | Project Tutorials
Python Tkinter | Widget Tutorials
TkDocs
TkDocs | Canvas
Python Tkinter Tutorial | Weapon Wheel Part 1
Python Tkinter Tutorial | Weapon Wheel Part 3
Python Tkinter Tutorial | Weapon Wheel Part 2
Python Tkinter Game: Weapon Swapper
Pro Gun Spinning - T.K.Hero
Making FPS Games in Python
Best Programming Languages #programming #coding #javascript
Create GUI in python using Tkinter Tutorial
Just 11 Lines of Code to Make this In PYTHON | Flower Python Code.
Crushing Crunchy & Soft Things by Car! EXPERIMENT: Car vs Coca Cola, Fanta, Mirinda Balloons
Make 3D FPS Game in Python (Ursina Engine)
Bro’s hacking life 😭🤣
Python Tkinter - keypad login
STOP Learning These Programming Languages (for Beginners)
Make your first GUI program in Python using tkinter || tkinter - 'Hello World'
Black Jack Demo (Python & TKinter)
Let's code a SNAKE GAME in python! 🐍
Python! Creating Selection Buttons with TKinter
Python Tkinter Game: Red vs Blue
How to Make a Game in Python: Tkinter, Pygame, and Kivy Comparison and Tutorial!
python programming with tkinter #1 gui applications with python
xavier memes #memes
TKinter Python Tutorials
Python3 GUI Programming with Tkinter (Tutorial 006) - Polonia Multi loto numbers generator
Комментарии