filmov
tv
Converting Integer to Binary in Python with Leading Zeros
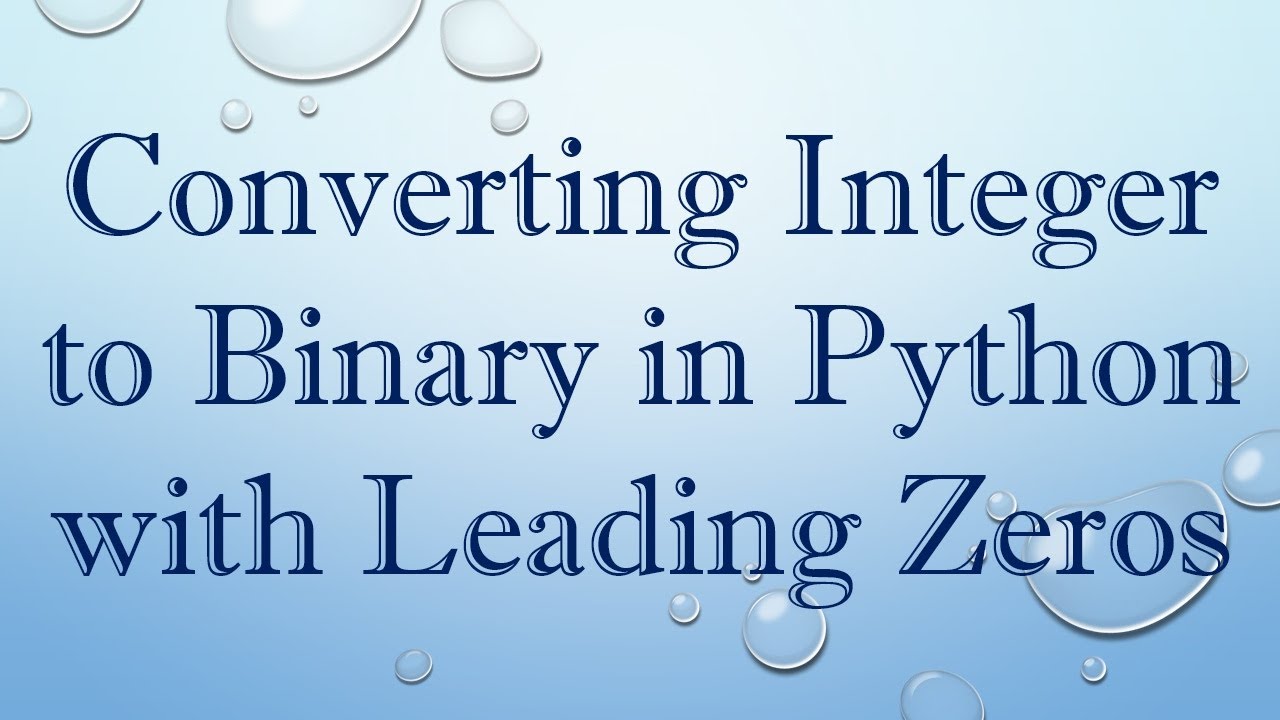
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to convert an integer to binary in Python while preserving leading zeros. Explore step-by-step instructions and examples for effective binary conversion.
---
Binary representation of integers is a fundamental concept in computer science and programming. In Python, converting an integer to its binary equivalent is a common task, but preserving leading zeros in the binary representation can be crucial in certain applications. This guide will guide you through the process of converting an integer to binary in Python while ensuring that leading zeros are retained.
Method 1: Using bin() Function
Python provides a built-in bin() function that converts an integer to its binary representation. However, by default, this function does not include leading zeros. Here's a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, bin(decimal_number) returns a string that starts with "0b," indicating that it's a binary representation. We use slicing ([2:]) to exclude these first two characters and get the binary representation without leading zeros.
Method 2: Using format() Function
Another approach is to use the format() function, which allows more control over the formatting of the binary representation:
[[See Video to Reveal this Text or Code Snippet]]
In this example, f'0{width}b' is a format specifier that ensures the binary representation has leading zeros and a minimum width of width. Adjust the width variable based on the number of leading zeros you want.
Method 3: Manual Conversion
For a more manual approach, you can create a custom function to convert an integer to binary with leading zeros:
[[See Video to Reveal this Text or Code Snippet]]
Here, zfill(width) pads the binary representation with zeros on the left until it reaches the specified width.
Conclusion
In this guide, we explored different methods to convert an integer to binary in Python while maintaining leading zeros. Whether you prefer using the built-in bin() function, the format() function, or a custom manual approach, understanding these techniques will empower you to handle binary representations effectively in your Python programs.
---
Summary: Learn how to convert an integer to binary in Python while preserving leading zeros. Explore step-by-step instructions and examples for effective binary conversion.
---
Binary representation of integers is a fundamental concept in computer science and programming. In Python, converting an integer to its binary equivalent is a common task, but preserving leading zeros in the binary representation can be crucial in certain applications. This guide will guide you through the process of converting an integer to binary in Python while ensuring that leading zeros are retained.
Method 1: Using bin() Function
Python provides a built-in bin() function that converts an integer to its binary representation. However, by default, this function does not include leading zeros. Here's a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, bin(decimal_number) returns a string that starts with "0b," indicating that it's a binary representation. We use slicing ([2:]) to exclude these first two characters and get the binary representation without leading zeros.
Method 2: Using format() Function
Another approach is to use the format() function, which allows more control over the formatting of the binary representation:
[[See Video to Reveal this Text or Code Snippet]]
In this example, f'0{width}b' is a format specifier that ensures the binary representation has leading zeros and a minimum width of width. Adjust the width variable based on the number of leading zeros you want.
Method 3: Manual Conversion
For a more manual approach, you can create a custom function to convert an integer to binary with leading zeros:
[[See Video to Reveal this Text or Code Snippet]]
Here, zfill(width) pads the binary representation with zeros on the left until it reaches the specified width.
Conclusion
In this guide, we explored different methods to convert an integer to binary in Python while maintaining leading zeros. Whether you prefer using the built-in bin() function, the format() function, or a custom manual approach, understanding these techniques will empower you to handle binary representations effectively in your Python programs.