filmov
tv
How to Convert a List of Characters into a String in Python
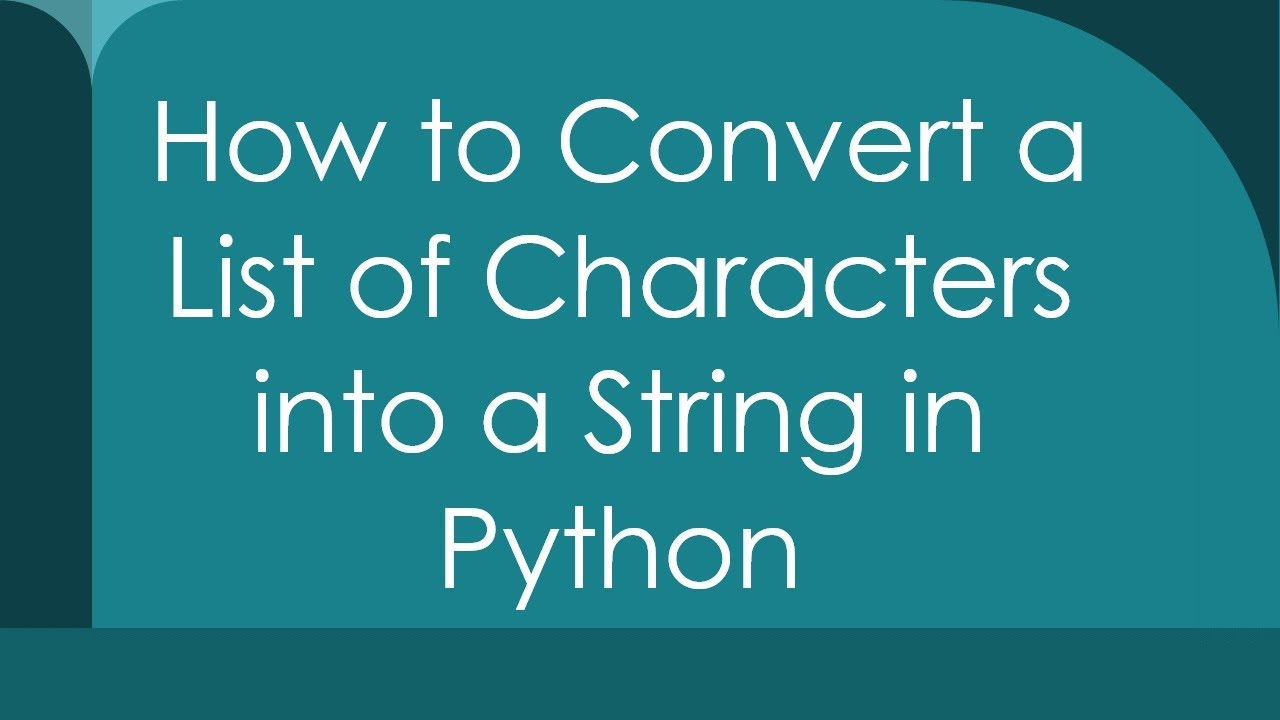
Показать описание
Summary: Learn how to convert a list of characters into a string in Python using different methods, including join() and list comprehension.
---
Converting a list of characters into a string is a common task in Python programming. This can be useful in various scenarios, such as processing user input, manipulating text data, or preparing data for output. In this post, we will explore different methods to achieve this conversion effectively.
Method 1: Using the join() Method
The most common and efficient way to convert a list of characters into a string is by using the join() method. This method concatenates all the elements of the list into a single string.
[[See Video to Reveal this Text or Code Snippet]]
In the example above, the join() method is called on an empty string (''), which acts as a separator. Since we don't need any separators between the characters, we use an empty string. The method then combines all the elements of char_list into one string.
Method 2: Using a For Loop
Another method to convert a list of characters into a string is by using a for loop. This approach manually concatenates each character in the list to form the final string.
[[See Video to Reveal this Text or Code Snippet]]
While this method is straightforward, it is less efficient than using join() due to the repeated string concatenation in the loop.
Method 3: Using List Comprehension
List comprehension can also be employed to convert a list of characters into a string. This method is a more concise way to achieve the same result as using a for loop.
[[See Video to Reveal this Text or Code Snippet]]
In this example, the list comprehension creates a new list of characters, which is then passed to the join() method to form the final string.
Conclusion
Converting a list of characters into a string in Python can be done using several methods, with join() being the most efficient and commonly used approach. Whether you choose to use join(), a for loop, or list comprehension, the result will be the same—a single string composed of the characters from the original list.
By understanding these methods, you can choose the one that best fits your coding style and specific use case, ensuring your code is both efficient and readable.
---
Converting a list of characters into a string is a common task in Python programming. This can be useful in various scenarios, such as processing user input, manipulating text data, or preparing data for output. In this post, we will explore different methods to achieve this conversion effectively.
Method 1: Using the join() Method
The most common and efficient way to convert a list of characters into a string is by using the join() method. This method concatenates all the elements of the list into a single string.
[[See Video to Reveal this Text or Code Snippet]]
In the example above, the join() method is called on an empty string (''), which acts as a separator. Since we don't need any separators between the characters, we use an empty string. The method then combines all the elements of char_list into one string.
Method 2: Using a For Loop
Another method to convert a list of characters into a string is by using a for loop. This approach manually concatenates each character in the list to form the final string.
[[See Video to Reveal this Text or Code Snippet]]
While this method is straightforward, it is less efficient than using join() due to the repeated string concatenation in the loop.
Method 3: Using List Comprehension
List comprehension can also be employed to convert a list of characters into a string. This method is a more concise way to achieve the same result as using a for loop.
[[See Video to Reveal this Text or Code Snippet]]
In this example, the list comprehension creates a new list of characters, which is then passed to the join() method to form the final string.
Conclusion
Converting a list of characters into a string in Python can be done using several methods, with join() being the most efficient and commonly used approach. Whether you choose to use join(), a for loop, or list comprehension, the result will be the same—a single string composed of the characters from the original list.
By understanding these methods, you can choose the one that best fits your coding style and specific use case, ensuring your code is both efficient and readable.