filmov
tv
Programming Interview 11: Print all subset of an array
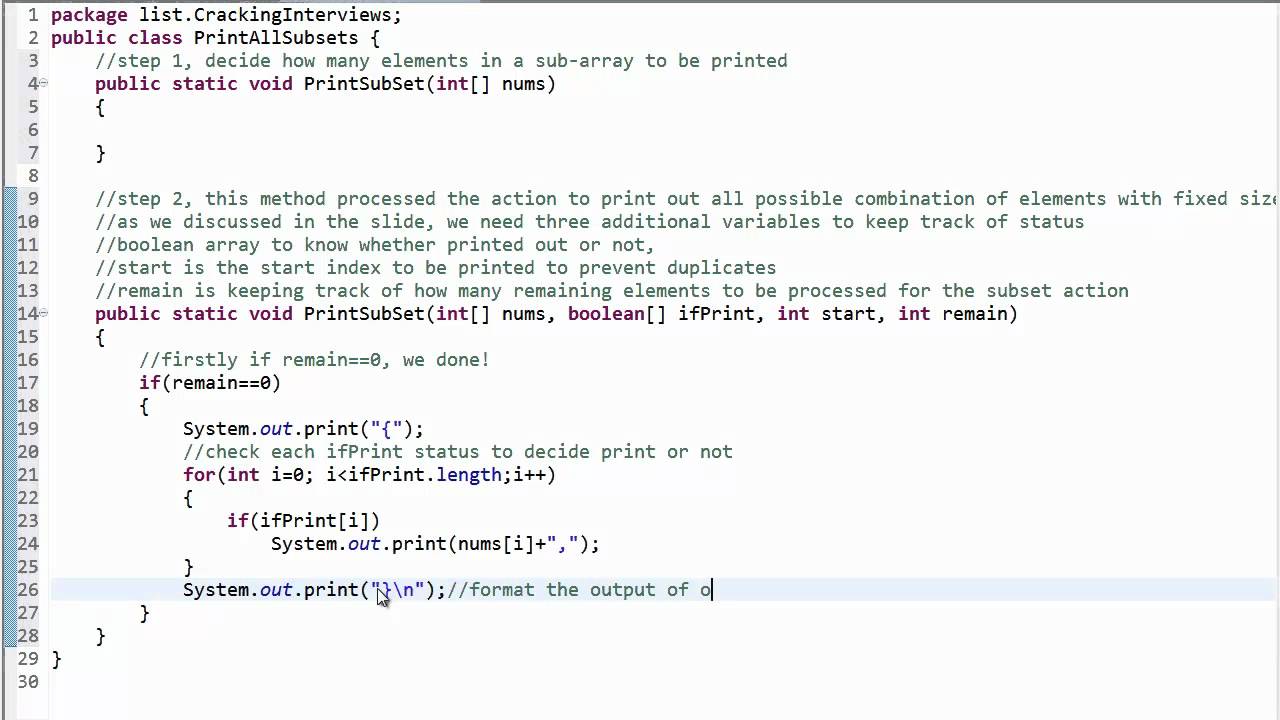
Показать описание
Step by step to crack Programming Interview questions 11: Print all subset of an array
Solution:
Step 1: Decide how many elements in a sub-set:
---Possible number of subset: 0 to array length;
Step 2: Recursively process elements from left to right:
---A boolean array to keep track of what elements to print;
---An alternative can be using swapping ;
---A start-index to know the next start index to be processed;
---A value to represent the remaining elements to be processed.
Thank you for watching.
Solution:
Step 1: Decide how many elements in a sub-set:
---Possible number of subset: 0 to array length;
Step 2: Recursively process elements from left to right:
---A boolean array to keep track of what elements to print;
---An alternative can be using swapping ;
---A start-index to know the next start index to be processed;
---A value to represent the remaining elements to be processed.
Thank you for watching.
Most Asked Coding Interview Question (Don't Skip !!😮) #shorts
Microsoft Coding Interview Question - Print Words Vertically (LeetCode)
Coding Interview Questions And Answers | Programming Interview Questions And Answers | Simplilearn
Interview Question | C Programming Language
Coding Interviews Need Backtracking! | Permutations - Leetcode 46
Top 25 Java 8 coding interview questions & answers | DevByteSchool
How to Print in Different Programming Languages
Python for Coding Interviews - Everything you need to Know
.NET CORE tutorials || Demo - 3 || by Mr. Mohan Reddy On 20-11-2024 @7PM IST
The HARDEST part about programming 🤦♂️ #code #programming #technology #tech #software #developer...
setTimeout + Closures Interview Question 🔥 | Namaste 🙏 JavaScript Ep. 11
Tricks for writing any Pattern program #shorts #youtubeshorts #python #java #coding
How can you duplicate an array? - Cracking the Java Coding Interview
Top 40 C Programming Interview Questions | C Programming Interview Questions And Answers|Simplilearn
Best programming language in 2023 || Top programming language from 2000 to 2023 😨🤯||#itdevelopment...
Solve Any Pattern Question With This Trick!
How to solve any Star Pattern Program
Programming Interview 6: String Permutation
Solve any Star Pattern program in Python
Python or Java? #codingninjas #coding #java #python
Programming Language Tier List
C++ Interview Questions And Answers | C++ Interview Questions And Answers For Freshers | Simplilearn
Sum of two number in c++ programming | c++ coding | programming in c++ language | cpp programming
Print all Permutation of given string, programming Interview Question
Комментарии