filmov
tv
🎯SQL Server Query Tuning Series: Leveraging Query Hints to Boost Execution Efficiency🎯
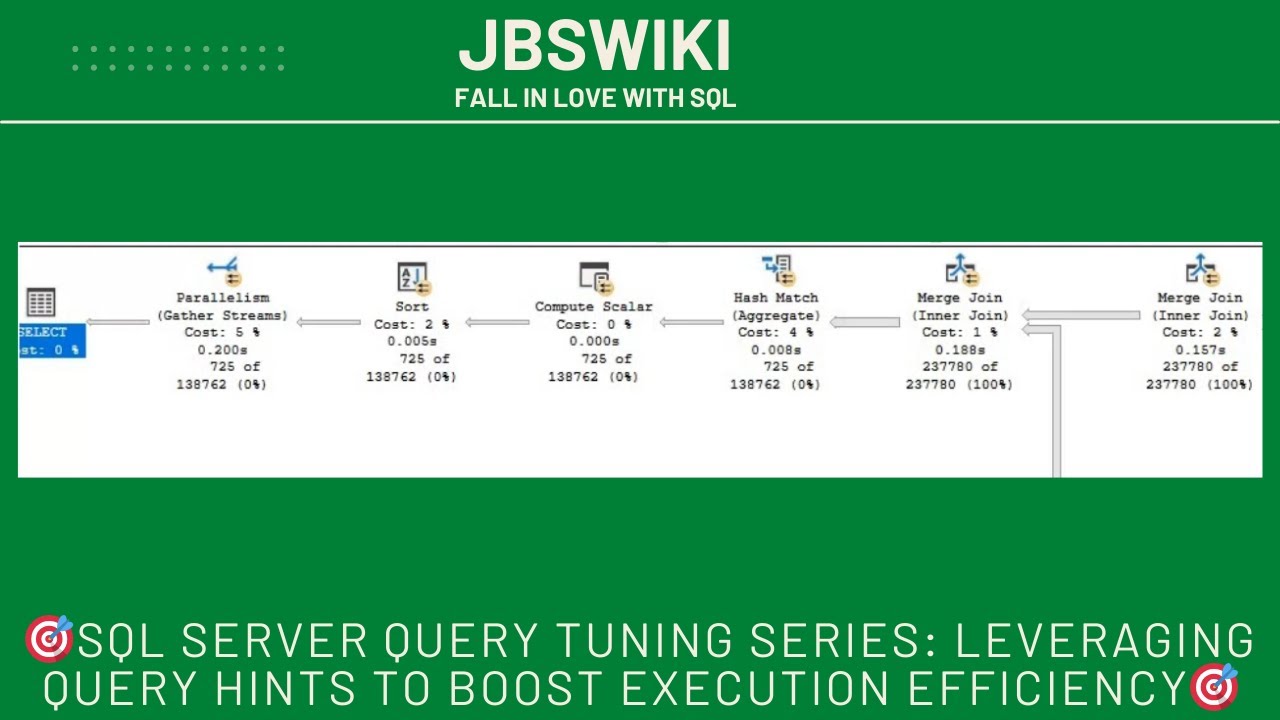
Показать описание
🔍 What Are Query Hints?
Query hints in SQL Server are directives that provide specific instructions to the query optimizer on how to execute a query. The optimizer typically chooses the best execution plan based on available resources, statistics, and internal rules. However, in certain cases, you may want to override the default behavior to gain better performance or to handle specific conditions.
SQL Server query hints allow you to:
Influence locking behavior.
Force index usage.
Manage parallelism.
Modify join behaviors.
Control query execution plans.
🧠 Why Use Query Hints?
Sometimes, SQL Server's optimizer might not select the best execution plan, especially in complex environments where statistics might be outdated or insufficient. 🚦 In these scenarios, using query hints can:
Force index usage when SQL Server chooses an inefficient index or ignores a better one.
Control parallelism in query execution, making sure you're neither overloading your CPU nor under-utilizing it.
Manage locking and isolation levels to prevent or control locking issues in heavily transactional systems.
Improve performance for specific queries in ways that are impossible using only general index tuning and query rewriting.
🛠️ How to Use Query Hints?
Query hints can be applied using the OPTION clause of your T-SQL query. Here’s the basic syntax:
SELECT column_list
FROM table
WHERE conditions
OPTION (query_hint);
Now, let's go over some of the most important query hints you’ll encounter in SQL Server tuning and how you can apply them.
1. FORCESEEK 🔍🔨
FORCESEEK forces SQL Server to use a seek operation on the specified index, which is typically more efficient than a scan when you're retrieving a small number of rows.
When you have a highly selective query (such as one based on a primary key) and SQL Server decides to scan the entire table due to outdated or insufficient statistics.
SELECT Name, Age
FROM Employees
WHERE EmployeeID = 123
OPTION (FORCESEEK);
In this case, we force SQL Server to perform an index seek on the EmployeeID column instead of scanning the table. 🚀
2. FORCESCAN 🔄🔍
In contrast to FORCESEEK, FORCESCAN forces SQL Server to perform an index scan on the table. This can be useful when a query benefits from reading a large number of rows.
When your query retrieves a significant portion of the data, scanning the index could be faster than seeking and jumping between pages.
SELECT Name, Department
FROM Employees
OPTION (FORCESCAN);
By specifying FORCESCAN, you’re telling SQL Server to scan the index, which can be more efficient when pulling a large number of rows from the table. 📋
3. NOLOCK 🔓
The NOLOCK hint allows you to retrieve data without acquiring locks. This hint can be useful in high-transaction environments where read operations are frequent, and waiting for locks could degrade performance.
If you need to run a SELECT query on a heavily updated table and can tolerate reading uncommitted data (i.e., dirty reads), NOLOCK can drastically reduce blocking.
SELECT OrderID, OrderDate
FROM Orders
WITH (NOLOCK);
This hint instructs SQL Server not to lock the rows or table, which improves concurrency in environments with high transaction volumes. ⚡
4. MAXDOP ⚙️💻
The MAXDOP (maximum degree of parallelism) hint limits the number of processors used to execute a query. SQL Server's default setting may lead to inefficient CPU usage in some cases.
When you have a query that would otherwise take advantage of parallelism, but the overhead of coordinating tasks across multiple CPUs is not worth the potential speed increase.
SELECT ProductID, SUM(Quantity)
FROM Sales
GROUP BY ProductID
OPTION (MAXDOP 1);
In this case, the query will execute on a single CPU core, reducing overhead in environments with constrained CPU resources. 🖥️
5. RECOMPILE ♻️
The RECOMPILE hint tells SQL Server to recompile the query every time it runs. This is particularly useful for queries that are highly sensitive to the parameters passed in and where the cached plan may be inefficient for certain sets of parameters.
For queries where parameter sniffing can lead to inefficient plans.
SELECT Name, Department
FROM Employees
WHERE Department = @Department
OPTION (RECOMPILE);
In this scenario, using the RECOMPILE hint ensures that SQL Server generates a new execution plan for each run of the query. This prevents the reuse of potentially inefficient plans. 🔄
6. OPTIMIZE FOR 🔧🎯
The OPTIMIZE FOR hint helps in cases of parameter sniffing by allowing you to specify which value SQL Server should optimize the plan for.
When parameter sniffing causes SQL Server to generate suboptimal plans for certain parameter values.
SELECT Name, Department
FROM Employees
WHERE Department = @Department
In this case, SQL Server optimizes the plan as if the parameter value @Department is always 'IT', even though it might be something else during execution. 🎯
Query hints in SQL Server are directives that provide specific instructions to the query optimizer on how to execute a query. The optimizer typically chooses the best execution plan based on available resources, statistics, and internal rules. However, in certain cases, you may want to override the default behavior to gain better performance or to handle specific conditions.
SQL Server query hints allow you to:
Influence locking behavior.
Force index usage.
Manage parallelism.
Modify join behaviors.
Control query execution plans.
🧠 Why Use Query Hints?
Sometimes, SQL Server's optimizer might not select the best execution plan, especially in complex environments where statistics might be outdated or insufficient. 🚦 In these scenarios, using query hints can:
Force index usage when SQL Server chooses an inefficient index or ignores a better one.
Control parallelism in query execution, making sure you're neither overloading your CPU nor under-utilizing it.
Manage locking and isolation levels to prevent or control locking issues in heavily transactional systems.
Improve performance for specific queries in ways that are impossible using only general index tuning and query rewriting.
🛠️ How to Use Query Hints?
Query hints can be applied using the OPTION clause of your T-SQL query. Here’s the basic syntax:
SELECT column_list
FROM table
WHERE conditions
OPTION (query_hint);
Now, let's go over some of the most important query hints you’ll encounter in SQL Server tuning and how you can apply them.
1. FORCESEEK 🔍🔨
FORCESEEK forces SQL Server to use a seek operation on the specified index, which is typically more efficient than a scan when you're retrieving a small number of rows.
When you have a highly selective query (such as one based on a primary key) and SQL Server decides to scan the entire table due to outdated or insufficient statistics.
SELECT Name, Age
FROM Employees
WHERE EmployeeID = 123
OPTION (FORCESEEK);
In this case, we force SQL Server to perform an index seek on the EmployeeID column instead of scanning the table. 🚀
2. FORCESCAN 🔄🔍
In contrast to FORCESEEK, FORCESCAN forces SQL Server to perform an index scan on the table. This can be useful when a query benefits from reading a large number of rows.
When your query retrieves a significant portion of the data, scanning the index could be faster than seeking and jumping between pages.
SELECT Name, Department
FROM Employees
OPTION (FORCESCAN);
By specifying FORCESCAN, you’re telling SQL Server to scan the index, which can be more efficient when pulling a large number of rows from the table. 📋
3. NOLOCK 🔓
The NOLOCK hint allows you to retrieve data without acquiring locks. This hint can be useful in high-transaction environments where read operations are frequent, and waiting for locks could degrade performance.
If you need to run a SELECT query on a heavily updated table and can tolerate reading uncommitted data (i.e., dirty reads), NOLOCK can drastically reduce blocking.
SELECT OrderID, OrderDate
FROM Orders
WITH (NOLOCK);
This hint instructs SQL Server not to lock the rows or table, which improves concurrency in environments with high transaction volumes. ⚡
4. MAXDOP ⚙️💻
The MAXDOP (maximum degree of parallelism) hint limits the number of processors used to execute a query. SQL Server's default setting may lead to inefficient CPU usage in some cases.
When you have a query that would otherwise take advantage of parallelism, but the overhead of coordinating tasks across multiple CPUs is not worth the potential speed increase.
SELECT ProductID, SUM(Quantity)
FROM Sales
GROUP BY ProductID
OPTION (MAXDOP 1);
In this case, the query will execute on a single CPU core, reducing overhead in environments with constrained CPU resources. 🖥️
5. RECOMPILE ♻️
The RECOMPILE hint tells SQL Server to recompile the query every time it runs. This is particularly useful for queries that are highly sensitive to the parameters passed in and where the cached plan may be inefficient for certain sets of parameters.
For queries where parameter sniffing can lead to inefficient plans.
SELECT Name, Department
FROM Employees
WHERE Department = @Department
OPTION (RECOMPILE);
In this scenario, using the RECOMPILE hint ensures that SQL Server generates a new execution plan for each run of the query. This prevents the reuse of potentially inefficient plans. 🔄
6. OPTIMIZE FOR 🔧🎯
The OPTIMIZE FOR hint helps in cases of parameter sniffing by allowing you to specify which value SQL Server should optimize the plan for.
When parameter sniffing causes SQL Server to generate suboptimal plans for certain parameter values.
SELECT Name, Department
FROM Employees
WHERE Department = @Department
In this case, SQL Server optimizes the plan as if the parameter value @Department is always 'IT', even though it might be something else during execution. 🎯