filmov
tv
4.C in Telugu Functions part 2
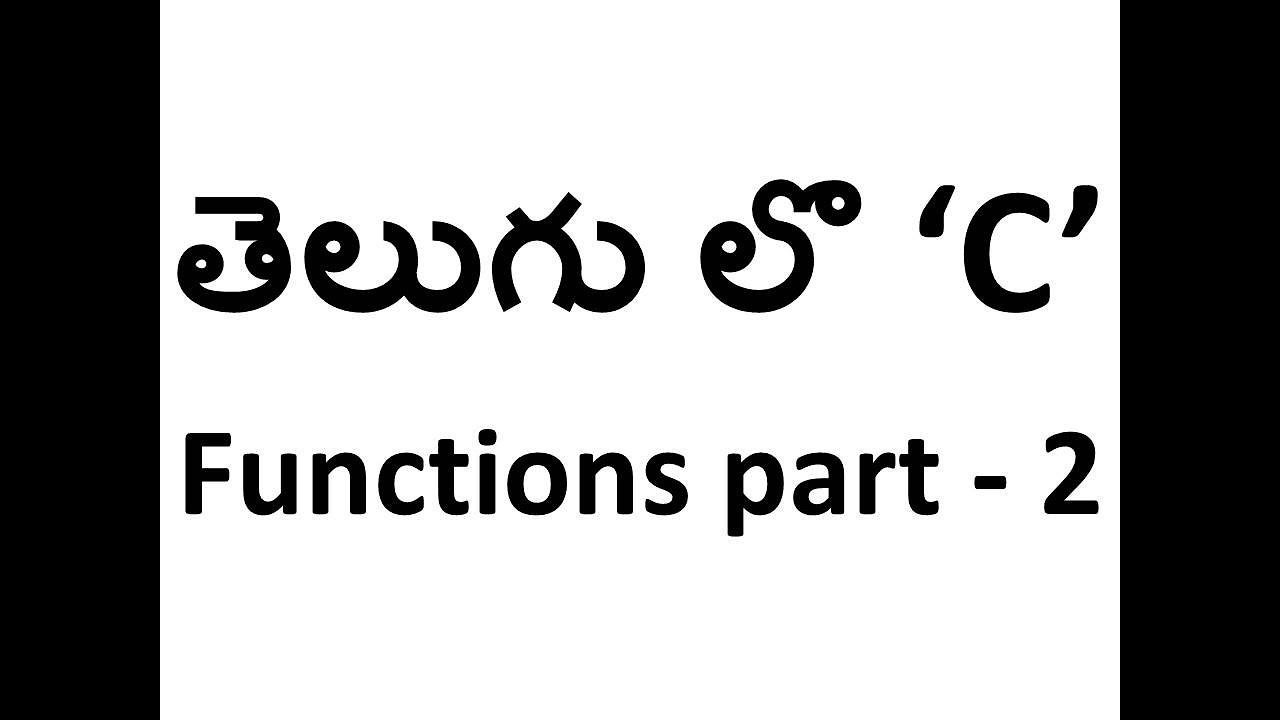
Показать описание
In C programming, all executable code resides within a function. A function is a named block of code that performs a task and then returns control to a caller. Note that other programming languages may distinguish between a "function", "subroutine", "subprogram", "procedure", or "method" -- in C, these are all functions.
A function is often executed (called) several times, from several different places, during a single execution of the program. After finishing a subroutine, the program will branch back (return) to the point after the call.
Functions are a powerful programming tool.
A function is like a black box. It takes in input, does something with it, then spits out an answer.
Note that a function may not take any inputs at all, or it may not return anything at all. In the above example, if we were to make a function of that loop, we may not need any inputs, and we aren't returning anything at all (Text output doesn't count - when we speak of returning we mean to say meaningful data that the program can use).
We have some terminology to refer to functions:
A function, call it f, that uses another function g, is said to call g. For example, f calls g to print the squares of ten numbers.
A function's inputs are known as its arguments
A function g that gives some kind of answer back to f is said to return that answer. For example, g returns the sum of its arguments.
atof(char*), atoi(char*), atol(char*)
strtod(char * str, char ** endptr ), strtol(char *str, char **endptr), strtoul(char *str, char **endptr)
rand(), srand()
malloc(size_t), calloc (size_t elements, size_t elementSize), realloc(void*, int)
free (void*)
exit(int), abort()
atexit(void (*func)())
getenv
system
qsort(void *, size_t number, size_t size, int (*sortfunc)(void*, void*))
abs, labs
div, ldiv
assert(int)
(constants only)
int raise(int sig). This
void* signal(int sig, void (*func)(int))
isalnum, isalpha, isblank
iscntrl, isdigit, isgraph
islower, isprint, ispunct
isspace, isupper, isxdigit
tolower, toupper
struct lconv* localeconv(void);
char* setlocale(int, const char*);
va_start (va_list, ap)
va_arg (ap, (type))
va_end (ap)
va_copy (va_list, va_list)
memcpy, memmove
memchr, memcmp, memset
strcat, strncat, strchr, strrchr
strcmp, strncmp, strccoll
strcpy, strncpy
strerror
strlen
strspn, strcspn
strpbrk
strstr
strtok
strxfrm
A function is often executed (called) several times, from several different places, during a single execution of the program. After finishing a subroutine, the program will branch back (return) to the point after the call.
Functions are a powerful programming tool.
A function is like a black box. It takes in input, does something with it, then spits out an answer.
Note that a function may not take any inputs at all, or it may not return anything at all. In the above example, if we were to make a function of that loop, we may not need any inputs, and we aren't returning anything at all (Text output doesn't count - when we speak of returning we mean to say meaningful data that the program can use).
We have some terminology to refer to functions:
A function, call it f, that uses another function g, is said to call g. For example, f calls g to print the squares of ten numbers.
A function's inputs are known as its arguments
A function g that gives some kind of answer back to f is said to return that answer. For example, g returns the sum of its arguments.
atof(char*), atoi(char*), atol(char*)
strtod(char * str, char ** endptr ), strtol(char *str, char **endptr), strtoul(char *str, char **endptr)
rand(), srand()
malloc(size_t), calloc (size_t elements, size_t elementSize), realloc(void*, int)
free (void*)
exit(int), abort()
atexit(void (*func)())
getenv
system
qsort(void *, size_t number, size_t size, int (*sortfunc)(void*, void*))
abs, labs
div, ldiv
assert(int)
(constants only)
int raise(int sig). This
void* signal(int sig, void (*func)(int))
isalnum, isalpha, isblank
iscntrl, isdigit, isgraph
islower, isprint, ispunct
isspace, isupper, isxdigit
tolower, toupper
struct lconv* localeconv(void);
char* setlocale(int, const char*);
va_start (va_list, ap)
va_arg (ap, (type))
va_end (ap)
va_copy (va_list, va_list)
memcpy, memmove
memchr, memcmp, memset
strcat, strncat, strchr, strrchr
strcmp, strncmp, strccoll
strcpy, strncpy
strerror
strlen
strspn, strcspn
strpbrk
strstr
strtok
strxfrm