filmov
tv
How to encrypt and decrypt text using python simple
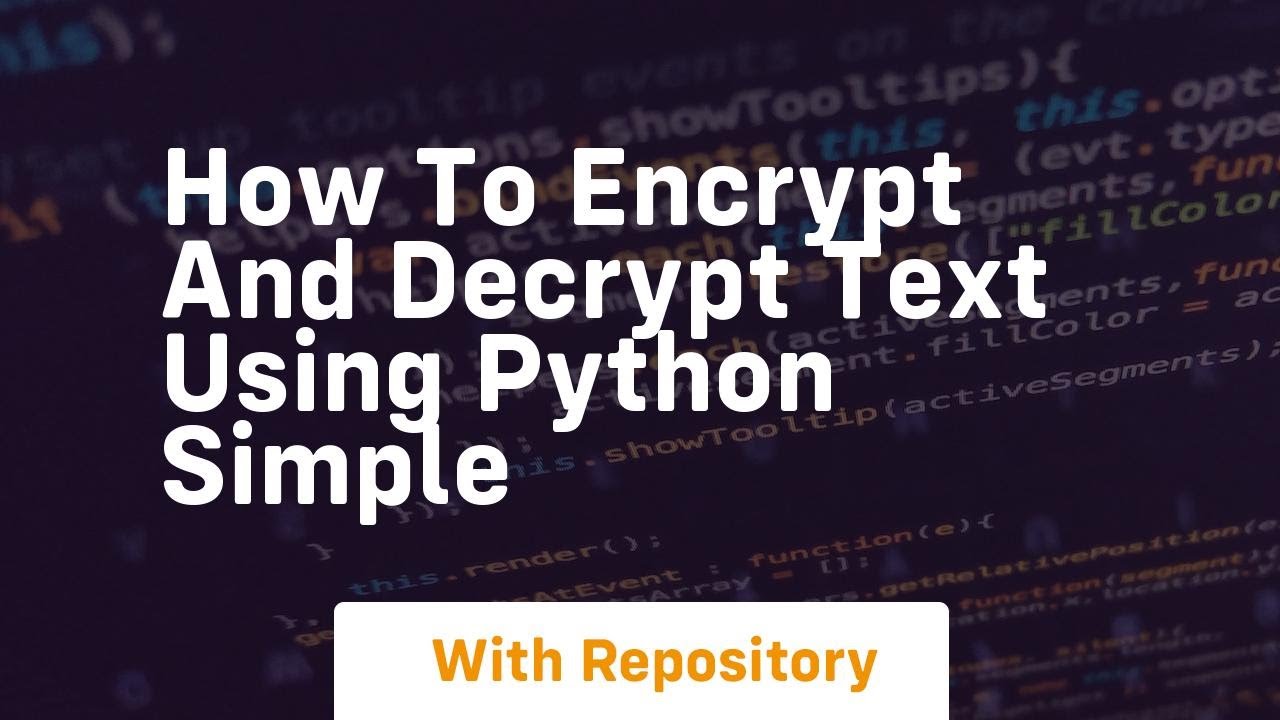
Показать описание
encrypting and decrypting text in python can be done using various libraries, but one of the most popular and straightforward methods is through the `cryptography` library. below is a tutorial that explains how to encrypt and decrypt text using this library.
### step 1: install the `cryptography` library
before we start coding, you need to install the `cryptography` library if you haven't already. you can do this using pip:
### step 2: import necessary modules
in your python script, you need to import the necessary modules from the `cryptography` library.
### step 3: generate a key
the first step in encryption is to generate a key. this key will be used both for encryption and decryption of your text.
### step 4: encrypt text
to encrypt text, use the `encrypt` method of the `fernet` object.
### step 5: decrypt text
to decrypt the text, use the `decrypt` method of the `fernet` object.
### full example code
here is the full code that combines all the steps mentioned above:
### important notes
1. **key storage**: the key generated should be stored securely. if you lose the key, you won't be able to decrypt the data.
2. **security considerations**: this method is suitable for simple encryption needs. for more complex requirements, consider additional measures such as key rotation, using secure storage for keys, and more.
3. **data types**: the `encrypt` and `decrypt` methods only work with bytes, so make sure to encode and decode strings as needed.
### conclusion
you now have a simple implementation of text encryption and decryption in python using the `cryptography` library. this method provides a straightforward way to secure your text data.
...
#python decrypt base64
#python decrypt file
#python decrypt online
#python decrypt md5
#python decrypt
python decrypt base64
python decrypt file
python decrypt online
python decrypt md5
python decrypt
python decrypt pgp file
python decrypt sha256
python decrypt password
python decrypt aes
python decrypt with private key
python encrypt json
python encrypt code
python encrypt password
python encrypt string with password
python encryption algorithms
python encrypt and decrypt string
python encryption online
python encrypt file
### step 1: install the `cryptography` library
before we start coding, you need to install the `cryptography` library if you haven't already. you can do this using pip:
### step 2: import necessary modules
in your python script, you need to import the necessary modules from the `cryptography` library.
### step 3: generate a key
the first step in encryption is to generate a key. this key will be used both for encryption and decryption of your text.
### step 4: encrypt text
to encrypt text, use the `encrypt` method of the `fernet` object.
### step 5: decrypt text
to decrypt the text, use the `decrypt` method of the `fernet` object.
### full example code
here is the full code that combines all the steps mentioned above:
### important notes
1. **key storage**: the key generated should be stored securely. if you lose the key, you won't be able to decrypt the data.
2. **security considerations**: this method is suitable for simple encryption needs. for more complex requirements, consider additional measures such as key rotation, using secure storage for keys, and more.
3. **data types**: the `encrypt` and `decrypt` methods only work with bytes, so make sure to encode and decode strings as needed.
### conclusion
you now have a simple implementation of text encryption and decryption in python using the `cryptography` library. this method provides a straightforward way to secure your text data.
...
#python decrypt base64
#python decrypt file
#python decrypt online
#python decrypt md5
#python decrypt
python decrypt base64
python decrypt file
python decrypt online
python decrypt md5
python decrypt
python decrypt pgp file
python decrypt sha256
python decrypt password
python decrypt aes
python decrypt with private key
python encrypt json
python encrypt code
python encrypt password
python encrypt string with password
python encryption algorithms
python encrypt and decrypt string
python encryption online
python encrypt file