filmov
tv
Handling Union Types in Python Pre-Commit with Pylint: Resolving Type Issues
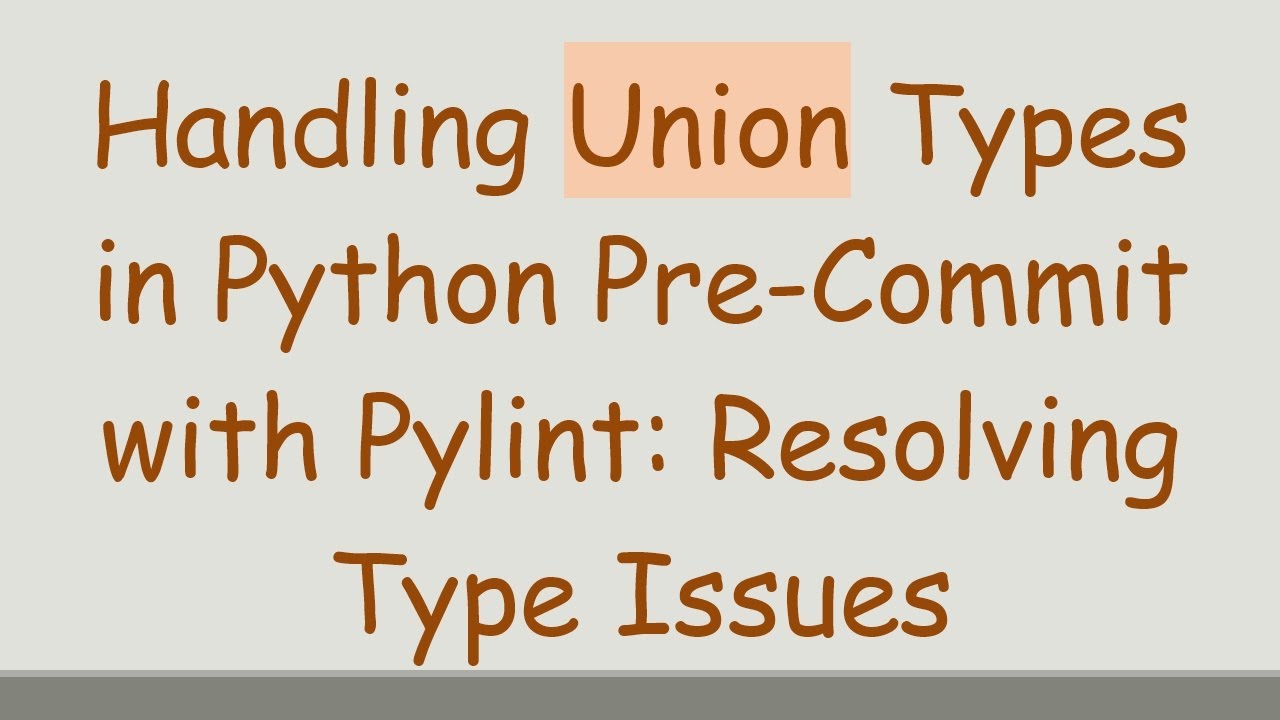
Показать описание
Discover how to tackle type errors in your Python functions when using `Union` types, especially with lists and booleans.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: pre-commit/pylint : two types possible for my input, but it doesn't get that in the instructions
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Handling Union Types in Python Pre-Commit with Pylint: Resolving Type Issues
When working with Python, especially in a type-annotated environment like Pylint or using type hints with Union, you may run into challenges surrounding how data types are perceived, especially when they can represent multiple forms. This guide addresses a common issue that arises when using Union types, particularly when a function's input can be either a boolean or a list.
The Problem
The challenge faced by many developers is that when using Union[List[bool], bool], the handling of the input does not clearly distinguish between the two types leading to type errors. Consider the following context from a user’s experience: they attempted to implement a function that accepts either a boolean value or a list of booleans. However, they faced an error indicating that a value of type Union[List[bool], Literal[True]] is not indexable. This arises because the Python interpreter cannot guarantee that the structured or grid variables are lists, particularly when they encounter a boolean value.
The Code Snippet
Here is the initial code that exhibited the issue:
[[See Video to Reveal this Text or Code Snippet]]
The Error
[[See Video to Reveal this Text or Code Snippet]]
This was problematic due to the ambiguity in the types being used, which led to errors when attempting to index the elements.
The Solution
To resolve this issue, a more explicit condition is required to verify whether structured and grid are indeed lists rather than a boolean. This ensures proper type narrowing, allowing for safe indexing and manipulation. Here’s how you can modify the code:
Step-by-Step Improvements
Replace the condition: Use isinstance to explicitly check that both structured and grid are lists.
Change type annotations: Consider using Optional[List[bool]] instead of Union, which offers the clarity of knowing when a variable might not hold a value.
Here’s the revised code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Changes
Using isinstance: This method checks if the variables are of a specific type (i.e., list), which avoids the ambiguity present in the original boolean checks.
Optional Type: By changing Union to Optional, you specify that the variables can either be None or a list, limiting potential errors in index handling.
Dealing with Mixed Types
If you're working with lists that contain different data types (like strings and integers), you can also address this within your function arguments. The appropriate annotation for a list containing both str and int would be:
[[See Video to Reveal this Text or Code Snippet]]
This allows you to flexibly work with a collection of various data types while maintaining clarity in how each element can be expected.
Conclusion
By using explicit type-checking and the Optional type, you can enhance your Python code's reliability and clarity, especially when working with complex type definitions. This not only resolves errors but also makes your codebase easier to navigate for future developers (or yourself!).
Make sure to review your type annotations and implement this structure to prevent similar errors in the future. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: pre-commit/pylint : two types possible for my input, but it doesn't get that in the instructions
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Handling Union Types in Python Pre-Commit with Pylint: Resolving Type Issues
When working with Python, especially in a type-annotated environment like Pylint or using type hints with Union, you may run into challenges surrounding how data types are perceived, especially when they can represent multiple forms. This guide addresses a common issue that arises when using Union types, particularly when a function's input can be either a boolean or a list.
The Problem
The challenge faced by many developers is that when using Union[List[bool], bool], the handling of the input does not clearly distinguish between the two types leading to type errors. Consider the following context from a user’s experience: they attempted to implement a function that accepts either a boolean value or a list of booleans. However, they faced an error indicating that a value of type Union[List[bool], Literal[True]] is not indexable. This arises because the Python interpreter cannot guarantee that the structured or grid variables are lists, particularly when they encounter a boolean value.
The Code Snippet
Here is the initial code that exhibited the issue:
[[See Video to Reveal this Text or Code Snippet]]
The Error
[[See Video to Reveal this Text or Code Snippet]]
This was problematic due to the ambiguity in the types being used, which led to errors when attempting to index the elements.
The Solution
To resolve this issue, a more explicit condition is required to verify whether structured and grid are indeed lists rather than a boolean. This ensures proper type narrowing, allowing for safe indexing and manipulation. Here’s how you can modify the code:
Step-by-Step Improvements
Replace the condition: Use isinstance to explicitly check that both structured and grid are lists.
Change type annotations: Consider using Optional[List[bool]] instead of Union, which offers the clarity of knowing when a variable might not hold a value.
Here’s the revised code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Changes
Using isinstance: This method checks if the variables are of a specific type (i.e., list), which avoids the ambiguity present in the original boolean checks.
Optional Type: By changing Union to Optional, you specify that the variables can either be None or a list, limiting potential errors in index handling.
Dealing with Mixed Types
If you're working with lists that contain different data types (like strings and integers), you can also address this within your function arguments. The appropriate annotation for a list containing both str and int would be:
[[See Video to Reveal this Text or Code Snippet]]
This allows you to flexibly work with a collection of various data types while maintaining clarity in how each element can be expected.
Conclusion
By using explicit type-checking and the Optional type, you can enhance your Python code's reliability and clarity, especially when working with complex type definitions. This not only resolves errors but also makes your codebase easier to navigate for future developers (or yourself!).
Make sure to review your type annotations and implement this structure to prevent similar errors in the future. Happy coding!