filmov
tv
How to Properly Call a Module Object in Python
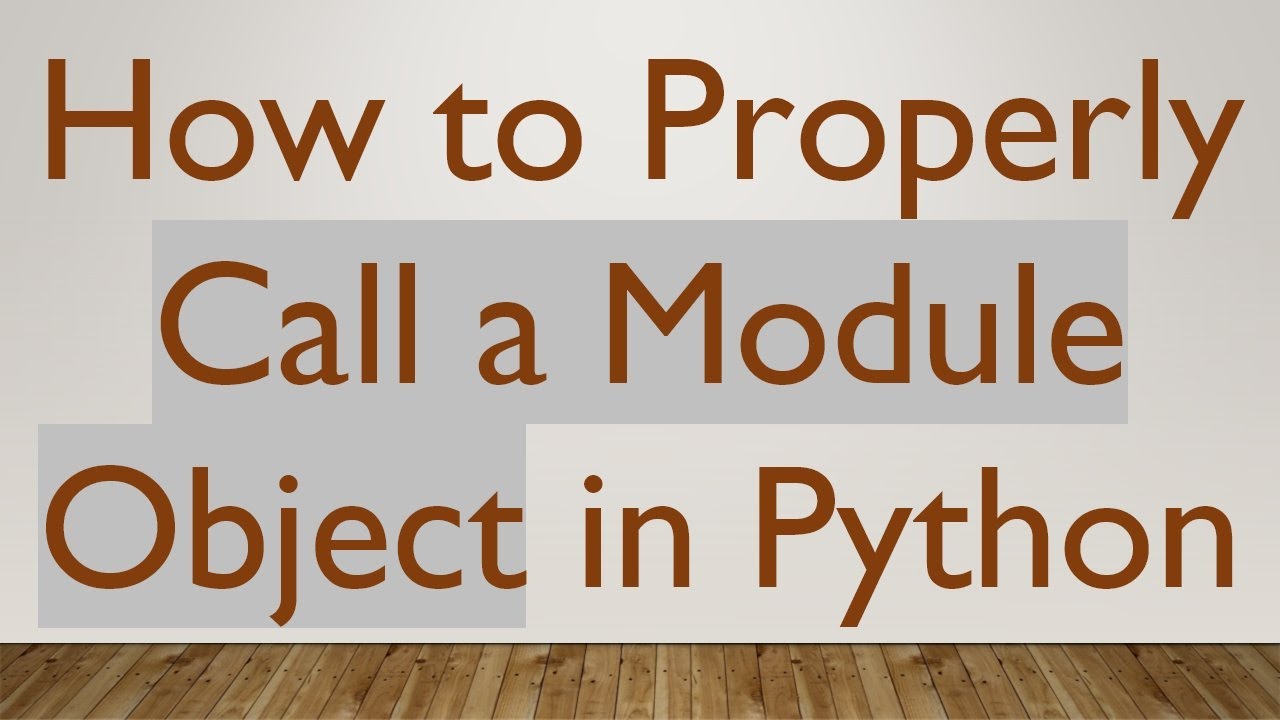
Показать описание
Learn how to fix the TypeError that occurs when you try to call a module object in Python by importing the correct class directly.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to call a module object?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Properly Call a Module Object in Python
If you're a Python developer, you might have faced issues when trying to use classes from a module. A common error that often perplexes beginners is the TypeError: 'module' object is not callable. In this guide, we'll discuss the context in which this error occurs and provide a clear solution to help you get your code up and running smoothly.
Understanding the Problem
Let's say you have a module named ant_colony which contains a class with the same name. You attempt to import this module using the following line of code:
[[See Video to Reveal this Text or Code Snippet]]
Next, you might try to instantiate the ant_colony class from this module like this:
[[See Video to Reveal this Text or Code Snippet]]
However, this results in an error:
[[See Video to Reveal this Text or Code Snippet]]
This error arises because you've imported the entire module instead of the specific class you need. The Python interpreter doesn't recognize ant_colony as a call to a class but rather as a reference to the module itself.
Solution: Import the Class Directly
To fix this error, you should import the ant_colony class directly from the module. Here’s how to do that:
[[See Video to Reveal this Text or Code Snippet]]
Now that you have imported the ant_colony class directly, you can create an instance of the class without any issues:
[[See Video to Reveal this Text or Code Snippet]]
Step-by-Step Guide
Delete the Incorrect Import Statement:
Remove or comment out the line where you imported the module using import ant_colony.
Add the Correct Import Statement:
Replace it with the correct line that imports the specific class:
[[See Video to Reveal this Text or Code Snippet]]
Instantiate the Class:
Use the new import to create an instance of the ant_colony class with the necessary arguments:
[[See Video to Reveal this Text or Code Snippet]]
Run Your Code:
With these changes, rerun your Python script. The error should now be resolved, and you should see your results printed as intended.
Conclusion
In summary, the TypeError: 'module' object is not callable occurs when you try to call a module as if it were a class or function. By directly importing the specific class, you avoid this pitfall and ensure your code works as expected. This quick fix can save you time and frustration while programming in Python.
Happy coding! If you have any more questions about Python modules or need help with similar issues, feel free to reach out!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to call a module object?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Properly Call a Module Object in Python
If you're a Python developer, you might have faced issues when trying to use classes from a module. A common error that often perplexes beginners is the TypeError: 'module' object is not callable. In this guide, we'll discuss the context in which this error occurs and provide a clear solution to help you get your code up and running smoothly.
Understanding the Problem
Let's say you have a module named ant_colony which contains a class with the same name. You attempt to import this module using the following line of code:
[[See Video to Reveal this Text or Code Snippet]]
Next, you might try to instantiate the ant_colony class from this module like this:
[[See Video to Reveal this Text or Code Snippet]]
However, this results in an error:
[[See Video to Reveal this Text or Code Snippet]]
This error arises because you've imported the entire module instead of the specific class you need. The Python interpreter doesn't recognize ant_colony as a call to a class but rather as a reference to the module itself.
Solution: Import the Class Directly
To fix this error, you should import the ant_colony class directly from the module. Here’s how to do that:
[[See Video to Reveal this Text or Code Snippet]]
Now that you have imported the ant_colony class directly, you can create an instance of the class without any issues:
[[See Video to Reveal this Text or Code Snippet]]
Step-by-Step Guide
Delete the Incorrect Import Statement:
Remove or comment out the line where you imported the module using import ant_colony.
Add the Correct Import Statement:
Replace it with the correct line that imports the specific class:
[[See Video to Reveal this Text or Code Snippet]]
Instantiate the Class:
Use the new import to create an instance of the ant_colony class with the necessary arguments:
[[See Video to Reveal this Text or Code Snippet]]
Run Your Code:
With these changes, rerun your Python script. The error should now be resolved, and you should see your results printed as intended.
Conclusion
In summary, the TypeError: 'module' object is not callable occurs when you try to call a module as if it were a class or function. By directly importing the specific class, you avoid this pitfall and ensure your code works as expected. This quick fix can save you time and frustration while programming in Python.
Happy coding! If you have any more questions about Python modules or need help with similar issues, feel free to reach out!