filmov
tv
how to check not null in javascript
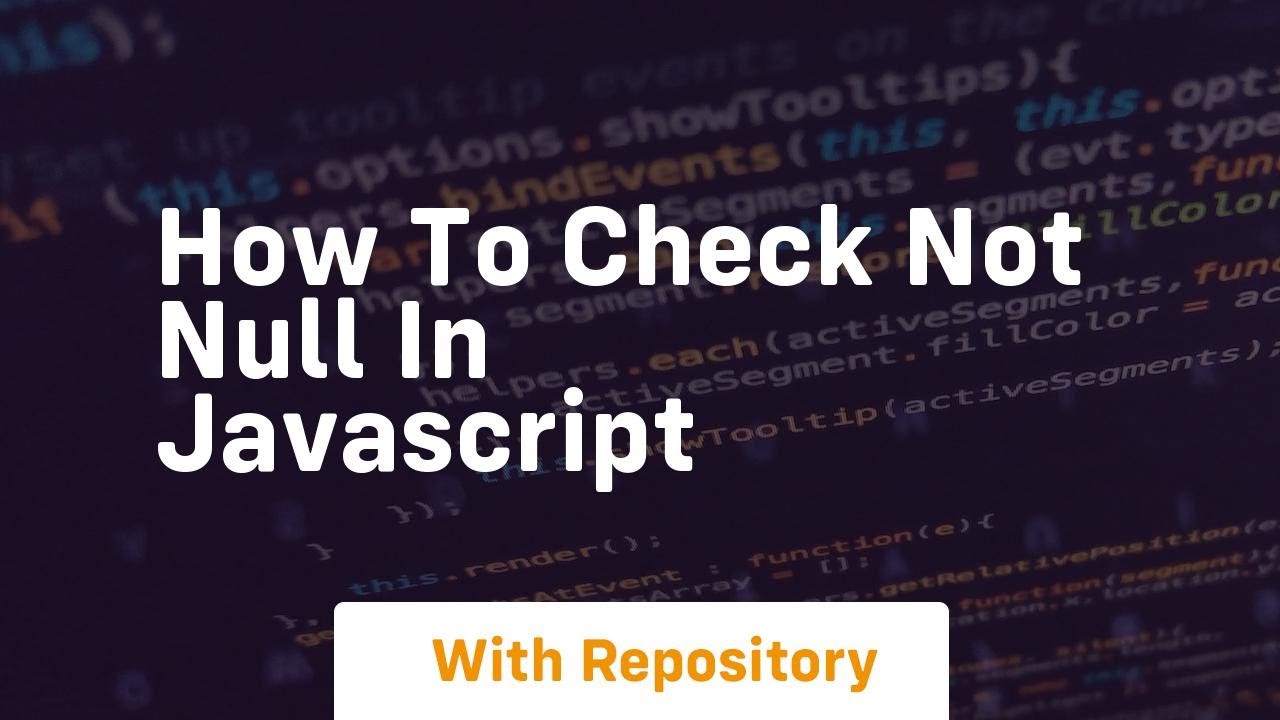
Показать описание
checking if a value is not null in javascript is a common task that helps ensure your code handles data properly. to check if a value is not null in javascript, you can use a simple comparison with the strict equality operator (`===`) or the not equal operator (`!==`).
here's a step-by-step tutorial on how to check if a value is not null in javascript with a code example:
1. using the strict equality operator (`===`):
in this example, we declare a variable `value` and assign it the value `null`. we then use an `if` statement to check if the value is not equal to `null` using the strict equality operator (`!==`). if the value is not null, it will print "value is not null"; otherwise, it will print "value is null".
2. using the not equal operator (`!==`):
in this example, we use the not equal operator (`!=`) to check if the value is not null. the `!=` operator performs type coercion, so it will consider `null` as equal to both `null` and `undefined`. if the value is not null, it will print "value is not null"; otherwise, it will print "value is null".
it's generally recommended to use the strict equality operator (`===`) for checking null values to avoid unexpected type coercion behavior.
by following these steps and examples, you can easily check if a value is not null in javascript and handle your data effectively in your code.
...
#javascript check if object is empty
#javascript check if undefined
#javascript check if array is empty
#javascript check if key exists
#javascript check if array
javascript check if object is empty
javascript check if undefined
javascript check if array is empty
javascript check if key exists
javascript check if array
javascript check if object has property
javascript check if null
javascript check if array contains value
javascript checker
javascript check for undefined
javascript null or undefined check
javascript null
javascript null conditional operator
javascript null operator
javascript null or empty string
javascript null coalesce
javascript null vs undefined
javascript null or undefined
here's a step-by-step tutorial on how to check if a value is not null in javascript with a code example:
1. using the strict equality operator (`===`):
in this example, we declare a variable `value` and assign it the value `null`. we then use an `if` statement to check if the value is not equal to `null` using the strict equality operator (`!==`). if the value is not null, it will print "value is not null"; otherwise, it will print "value is null".
2. using the not equal operator (`!==`):
in this example, we use the not equal operator (`!=`) to check if the value is not null. the `!=` operator performs type coercion, so it will consider `null` as equal to both `null` and `undefined`. if the value is not null, it will print "value is not null"; otherwise, it will print "value is null".
it's generally recommended to use the strict equality operator (`===`) for checking null values to avoid unexpected type coercion behavior.
by following these steps and examples, you can easily check if a value is not null in javascript and handle your data effectively in your code.
...
#javascript check if object is empty
#javascript check if undefined
#javascript check if array is empty
#javascript check if key exists
#javascript check if array
javascript check if object is empty
javascript check if undefined
javascript check if array is empty
javascript check if key exists
javascript check if array
javascript check if object has property
javascript check if null
javascript check if array contains value
javascript checker
javascript check for undefined
javascript null or undefined check
javascript null
javascript null conditional operator
javascript null operator
javascript null or empty string
javascript null coalesce
javascript null vs undefined
javascript null or undefined