filmov
tv
Create a Restful WCF Web Service with POST method using ASP.NET C# | Part 8
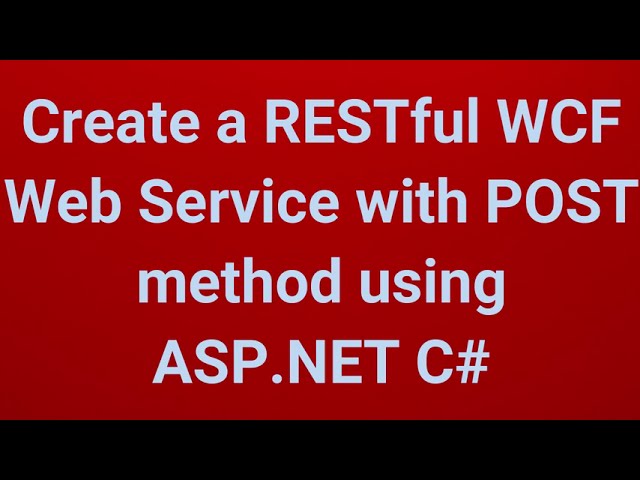
Показать описание
Create a Restful WCF Web Service with POST method using ASP.NET C# :
What is WCF Web Services:
WCF is a framework for building service-oriented applications.
and it allows communication between different applications over various protocols.
Steps:
Open visual studio 2015 and add a new empty website.
Three pages will be added in the project solution
ServiceContract(IEmployeeService) attribute defines the interface as a WCF service.
OperationContract attribute marks the method as a service operation.
WebInvoke attribute configures the HTTP POST method and XML format for request and response.
[ServiceContract]
public interface IEmployeeService
{
[WebInvoke(Method = "POST", RequestFormat = WebMessageFormat.Xml, ResponseFormat = WebMessageFormat.Xml, BodyStyle = WebMessageBodyStyle.Bare, UriTemplate = "/getempbyid")]
[OperationContract]
XmlElement getempbyid(employee emp);
}
[DataContract(Namespace = "AA")]
public class employee
{
[DataMember]
public int empid { get; set; }
}
AspNetCompatibilityRequirements: allows ASP.NET compatibility mode.
*Implements the IEmployeeService interface.
*Defines the employeedata method to retrieve and return employee data as XML.
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class EmployeeService : IEmployeeService
{
public XmlElement getempbyid(employee emp)
{
SqlConnection con = new SqlConnection("Data Source=DESKTOP-HSSNFCU;Initial Catalog=Test;Persist Security Info=True;User ID=sa;Password=1234");
string sql = "SELECT * from Employee where empid="+emp_id;
// Create a new DataSet
DataSet ds = new DataSet();
// Create a SqlDataAdapter
SqlDataAdapter da = new SqlDataAdapter(sql, con);
// Fill the DataSet
da.Fill(ds, "employeedetails");
// Create an XmlDocument
XmlDocument xmlDoc = new XmlDocument();
// Create the root element
XmlElement root = xmlDoc.CreateElement("employeedetails");
xmlDoc.AppendChild(root);
// Get the DataTable from the DataSet
DataTable dataTable = ds.Tables["employeedetails"];
// Iterate through each DataRow and create XML elements
foreach (DataRow row in dataTable.Rows)
{
// Create an employee element
XmlElement employee = xmlDoc.CreateElement("employee");
root.AppendChild(employee);
// Create and append child elements
XmlElement empid = xmlDoc.CreateElement("empid");
empid.InnerText = row["EmpId"].ToString();
employee.AppendChild(empid);
XmlElement empName = xmlDoc.CreateElement("emp_name");
empName.InnerText = row["emp_name"].ToString();
employee.AppendChild(empName);
XmlElement salary = xmlDoc.CreateElement("salary");
salary.InnerText = row["Salary"].ToString();
employee.AppendChild(salary);
XmlElement empCity = xmlDoc.CreateElement("emp_city");
empCity.InnerText = row["emp_city"].ToString();
employee.AppendChild(empCity);
}
string xmlstring = xmlDoc.InnerXml.ToString();
// convert xml string to xml element to return
var doc1 = new XmlDocument();
doc1.LoadXml(xmlstring);
return doc1.DocumentElement;
}
}
What is WCF Web Services:
WCF is a framework for building service-oriented applications.
and it allows communication between different applications over various protocols.
Steps:
Open visual studio 2015 and add a new empty website.
Three pages will be added in the project solution
ServiceContract(IEmployeeService) attribute defines the interface as a WCF service.
OperationContract attribute marks the method as a service operation.
WebInvoke attribute configures the HTTP POST method and XML format for request and response.
[ServiceContract]
public interface IEmployeeService
{
[WebInvoke(Method = "POST", RequestFormat = WebMessageFormat.Xml, ResponseFormat = WebMessageFormat.Xml, BodyStyle = WebMessageBodyStyle.Bare, UriTemplate = "/getempbyid")]
[OperationContract]
XmlElement getempbyid(employee emp);
}
[DataContract(Namespace = "AA")]
public class employee
{
[DataMember]
public int empid { get; set; }
}
AspNetCompatibilityRequirements: allows ASP.NET compatibility mode.
*Implements the IEmployeeService interface.
*Defines the employeedata method to retrieve and return employee data as XML.
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class EmployeeService : IEmployeeService
{
public XmlElement getempbyid(employee emp)
{
SqlConnection con = new SqlConnection("Data Source=DESKTOP-HSSNFCU;Initial Catalog=Test;Persist Security Info=True;User ID=sa;Password=1234");
string sql = "SELECT * from Employee where empid="+emp_id;
// Create a new DataSet
DataSet ds = new DataSet();
// Create a SqlDataAdapter
SqlDataAdapter da = new SqlDataAdapter(sql, con);
// Fill the DataSet
da.Fill(ds, "employeedetails");
// Create an XmlDocument
XmlDocument xmlDoc = new XmlDocument();
// Create the root element
XmlElement root = xmlDoc.CreateElement("employeedetails");
xmlDoc.AppendChild(root);
// Get the DataTable from the DataSet
DataTable dataTable = ds.Tables["employeedetails"];
// Iterate through each DataRow and create XML elements
foreach (DataRow row in dataTable.Rows)
{
// Create an employee element
XmlElement employee = xmlDoc.CreateElement("employee");
root.AppendChild(employee);
// Create and append child elements
XmlElement empid = xmlDoc.CreateElement("empid");
empid.InnerText = row["EmpId"].ToString();
employee.AppendChild(empid);
XmlElement empName = xmlDoc.CreateElement("emp_name");
empName.InnerText = row["emp_name"].ToString();
employee.AppendChild(empName);
XmlElement salary = xmlDoc.CreateElement("salary");
salary.InnerText = row["Salary"].ToString();
employee.AppendChild(salary);
XmlElement empCity = xmlDoc.CreateElement("emp_city");
empCity.InnerText = row["emp_city"].ToString();
employee.AppendChild(empCity);
}
string xmlstring = xmlDoc.InnerXml.ToString();
// convert xml string to xml element to return
var doc1 = new XmlDocument();
doc1.LoadXml(xmlstring);
return doc1.DocumentElement;
}
}