filmov
tv
Convert a String to Datetime in Python
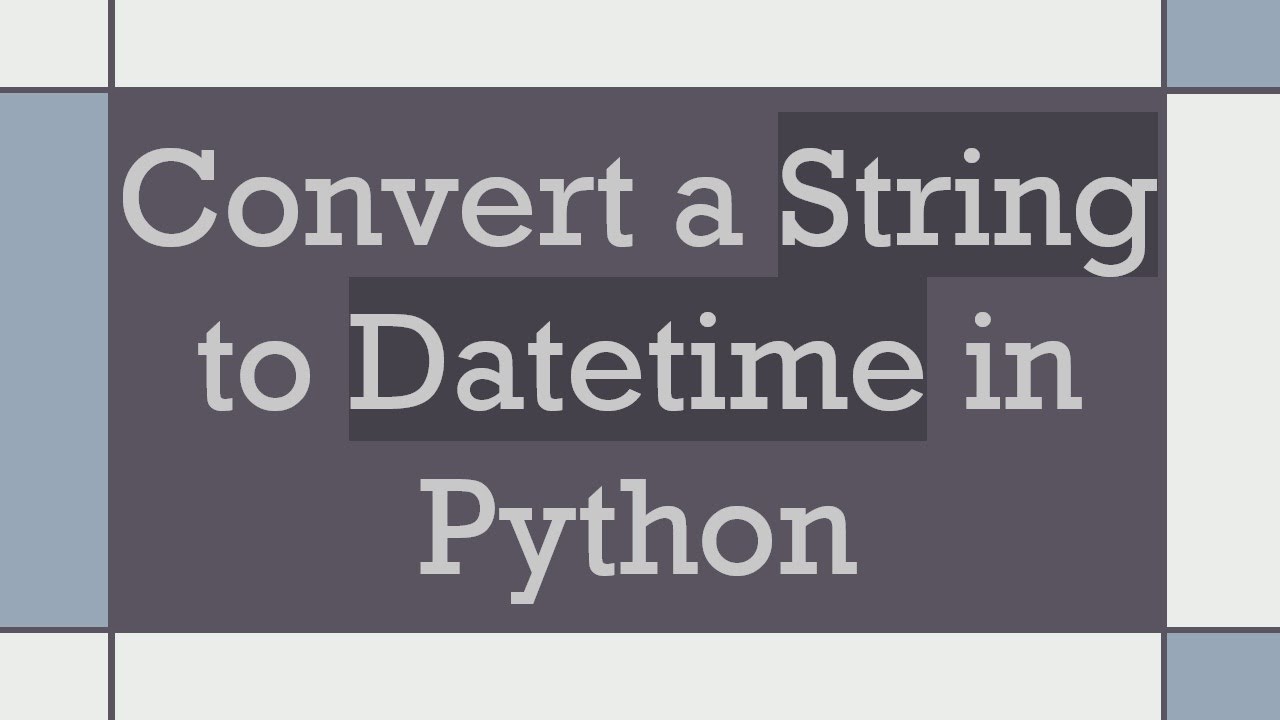
Показать описание
Learn how to convert a string in the format '2017-12-02 23:55:66.333+01:00' to a `datetime` object in Python with our straightforward guide.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert a str like this format '2017-12-02 23:55:66.333+01:00' to datetime in python?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting a String to Datetime in Python: A Step-by-Step Guide
When working with date and time data in Python, you might often encounter strings that represent date and time in various formats. One common scenario is needing to convert a string formatted like '2017-12-02 23:55:66.333+01:00' into a datetime object. However, what happens when this string doesn't quite fit the standard format you expect? This guide will guide you through the process of resolving that discrepancy and successfully converting your string to a datetime object.
The Problem
You may have tried to convert the string using a common method in Python. For instance, the attempt to use the strptime function, which is designed for parsing strings into datetime objects, might have resulted in an error like this:
[[See Video to Reveal this Text or Code Snippet]]
The error indicates that there’s something wrong with the format of the string you are trying to parse. In this case, there are two key issues with the input string.
Identifying Issues in the String
1. Invalid Seconds Value
The string contains seconds (66), which exceed the maximum possible value of 59. Valid seconds should always be in the range of 00 to 59.
2. Missing Microseconds Formatter
To accurately parse the milliseconds portion of the string (.333 here), you need to include a microseconds formatter in your strptime call.
The Solution
To successfully convert the string, you’ll need to adjust both the seconds value and your formatting string.
Step 1: Fix the String
You should modify the string to contain valid seconds. For example:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Correct Format
Then, you'll want to use the correct format with the strptime function. Below is the corrected code:
[[See Video to Reveal this Text or Code Snippet]]
Output
This code will yield the following output:
[[See Video to Reveal this Text or Code Snippet]]
Handling Errors Gracefully
To improve robustness, you may want to include error handling to catch exceptions that arise during conversion. You can use the following modified code to catch specific errors and provide useful feedback:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following these steps, you can effectively convert a string in the format '2017-12-02 23:55:66.333+01:00' to a datetime object in Python. Remember to ensure the string conforms to a valid datetime format, particularly in the range of seconds and the inclusion of microseconds. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert a str like this format '2017-12-02 23:55:66.333+01:00' to datetime in python?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting a String to Datetime in Python: A Step-by-Step Guide
When working with date and time data in Python, you might often encounter strings that represent date and time in various formats. One common scenario is needing to convert a string formatted like '2017-12-02 23:55:66.333+01:00' into a datetime object. However, what happens when this string doesn't quite fit the standard format you expect? This guide will guide you through the process of resolving that discrepancy and successfully converting your string to a datetime object.
The Problem
You may have tried to convert the string using a common method in Python. For instance, the attempt to use the strptime function, which is designed for parsing strings into datetime objects, might have resulted in an error like this:
[[See Video to Reveal this Text or Code Snippet]]
The error indicates that there’s something wrong with the format of the string you are trying to parse. In this case, there are two key issues with the input string.
Identifying Issues in the String
1. Invalid Seconds Value
The string contains seconds (66), which exceed the maximum possible value of 59. Valid seconds should always be in the range of 00 to 59.
2. Missing Microseconds Formatter
To accurately parse the milliseconds portion of the string (.333 here), you need to include a microseconds formatter in your strptime call.
The Solution
To successfully convert the string, you’ll need to adjust both the seconds value and your formatting string.
Step 1: Fix the String
You should modify the string to contain valid seconds. For example:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Correct Format
Then, you'll want to use the correct format with the strptime function. Below is the corrected code:
[[See Video to Reveal this Text or Code Snippet]]
Output
This code will yield the following output:
[[See Video to Reveal this Text or Code Snippet]]
Handling Errors Gracefully
To improve robustness, you may want to include error handling to catch exceptions that arise during conversion. You can use the following modified code to catch specific errors and provide useful feedback:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following these steps, you can effectively convert a string in the format '2017-12-02 23:55:66.333+01:00' to a datetime object in Python. Remember to ensure the string conforms to a valid datetime format, particularly in the range of seconds and the inclusion of microseconds. Happy coding!