filmov
tv
How to Convert a List of Integers to Their Corresponding ASCII Hex Values in Python
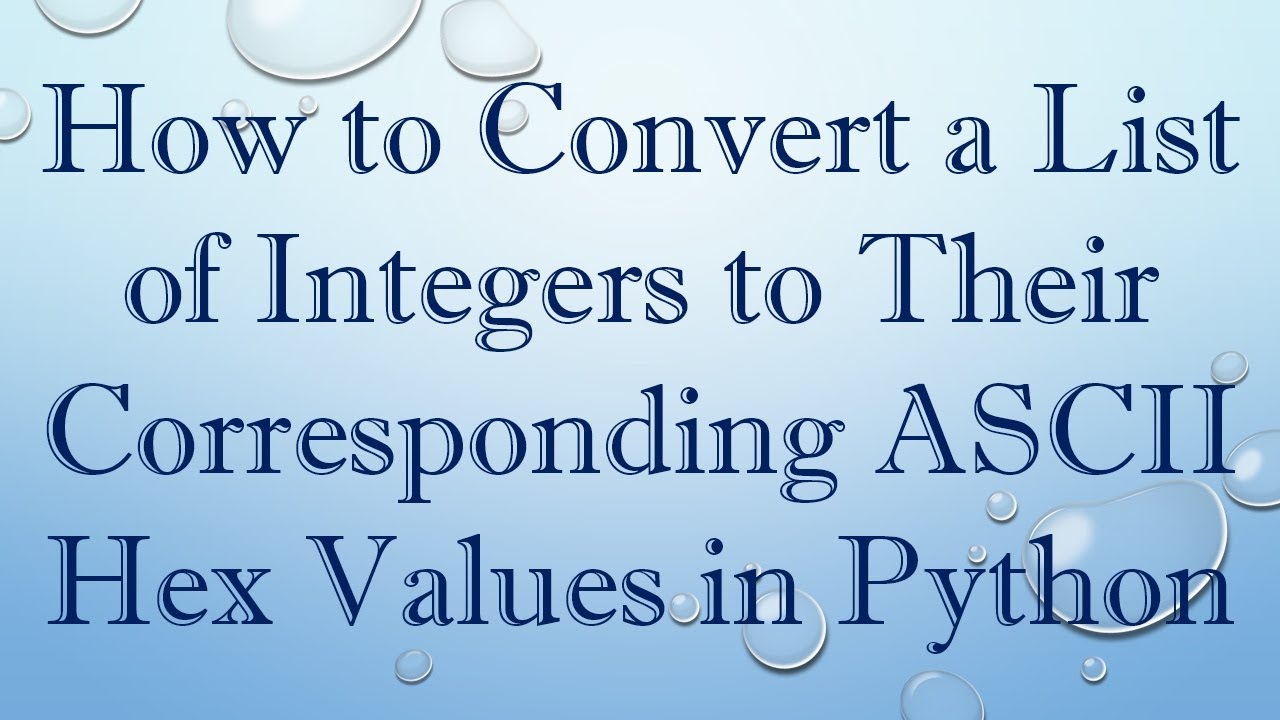
Показать описание
Learn how to efficiently convert a list of integers, regardless of their length, into their corresponding ASCII hex values using Python!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: In python, how would I convert a list of different length numbers to a list the same ascii characters?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting Integers to ASCII Characters in Python
Have you ever found yourself stuck trying to convert a list of integers of varying lengths into ASCII characters? This is a common challenge for those working with data in Python, especially when the requirement to convert those integers into a hexadecimal format arises. In this post, we'll explore how to effectively tackle this problem in a clear and structured way.
Understanding the Problem
Let’s define our starting point. Suppose you have a list of integers like the following:
[[See Video to Reveal this Text or Code Snippet]]
You want to convert each integer into its ASCII character equivalent. Single-digit numbers should be converted to their respective ASCII values, while double-digit numbers need to be dealt with in their entirety. The goal is to obtain the following output in hexadecimal format:
[[See Video to Reveal this Text or Code Snippet]]
However, you encountered issues with your initial attempts, especially when trying to process multi-digit numbers separately. Let's break down the solution.
The Solution
To solve this problem, we will adopt a simple Python list comprehension method. This method efficiently processes each number in the list, checking whether it's a single-digit number or not.
Step-by-Step Breakdown
List Comprehension: We will utilize a list comprehension to iterate through the list.
Condition Check: For each number in the list, we'll use a conditional expression:
If the number is a single digit (less than 10), we’ll convert it to its ASCII character using the ord() function.
If the number is a double-digit or greater, we’ll keep it as it is.
Formatting to Hex: The result will be formatted into hexadecimal representation.
Implementation in Python
Here is how you can implement this logic in Python:
[[See Video to Reveal this Text or Code Snippet]]
Output Explanation
When you run the code above, it will generate the desired output:
[[See Video to Reveal this Text or Code Snippet]]
In this output, each digit from the original list has been converted into its corresponding ASCII hex value, while the double-digit numbers remain unchanged.
Conclusion
Converting integers to ASCII characters in Python can initially seem complicated, particularly when dealing with numbers of varying lengths. However, with the straightforward method outlined above, you can achieve your desired outcome effectively.
The key takeaway is to leverage Python's powerful list comprehension feature combined with simple conditional logic to handle different scenarios gracefully. Now, if you ever need to perform this kind of conversion again, you'll know exactly how to tackle it!
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: In python, how would I convert a list of different length numbers to a list the same ascii characters?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting Integers to ASCII Characters in Python
Have you ever found yourself stuck trying to convert a list of integers of varying lengths into ASCII characters? This is a common challenge for those working with data in Python, especially when the requirement to convert those integers into a hexadecimal format arises. In this post, we'll explore how to effectively tackle this problem in a clear and structured way.
Understanding the Problem
Let’s define our starting point. Suppose you have a list of integers like the following:
[[See Video to Reveal this Text or Code Snippet]]
You want to convert each integer into its ASCII character equivalent. Single-digit numbers should be converted to their respective ASCII values, while double-digit numbers need to be dealt with in their entirety. The goal is to obtain the following output in hexadecimal format:
[[See Video to Reveal this Text or Code Snippet]]
However, you encountered issues with your initial attempts, especially when trying to process multi-digit numbers separately. Let's break down the solution.
The Solution
To solve this problem, we will adopt a simple Python list comprehension method. This method efficiently processes each number in the list, checking whether it's a single-digit number or not.
Step-by-Step Breakdown
List Comprehension: We will utilize a list comprehension to iterate through the list.
Condition Check: For each number in the list, we'll use a conditional expression:
If the number is a single digit (less than 10), we’ll convert it to its ASCII character using the ord() function.
If the number is a double-digit or greater, we’ll keep it as it is.
Formatting to Hex: The result will be formatted into hexadecimal representation.
Implementation in Python
Here is how you can implement this logic in Python:
[[See Video to Reveal this Text or Code Snippet]]
Output Explanation
When you run the code above, it will generate the desired output:
[[See Video to Reveal this Text or Code Snippet]]
In this output, each digit from the original list has been converted into its corresponding ASCII hex value, while the double-digit numbers remain unchanged.
Conclusion
Converting integers to ASCII characters in Python can initially seem complicated, particularly when dealing with numbers of varying lengths. However, with the straightforward method outlined above, you can achieve your desired outcome effectively.
The key takeaway is to leverage Python's powerful list comprehension feature combined with simple conditional logic to handle different scenarios gracefully. Now, if you ever need to perform this kind of conversion again, you'll know exactly how to tackle it!
Happy coding!