filmov
tv
Create a Dictionary from Multiple Columns in Pandas DataFrame
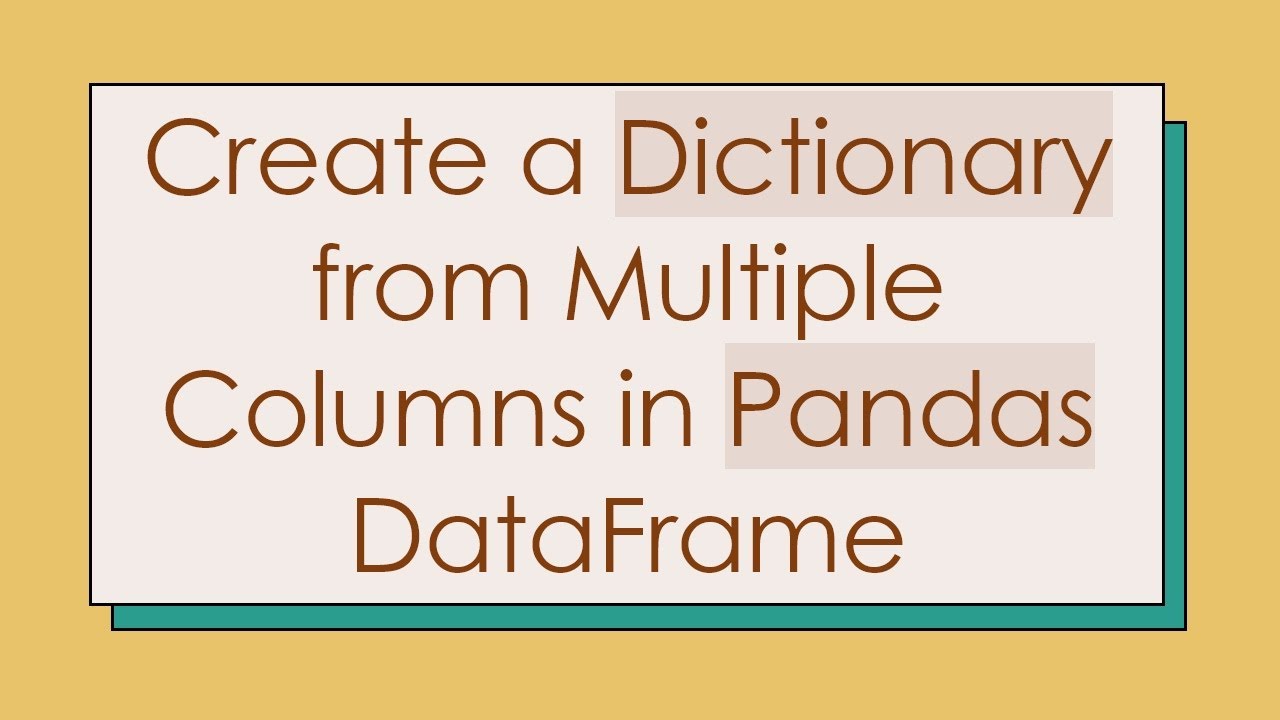
Показать описание
Learn how to efficiently generate a nested dictionary from several columns in a `Pandas` DataFrame based on the position of values.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Create dictionary from several columns based on position of values
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Dictionary from Multiple Columns in a Pandas DataFrame
When working with data in Pandas, you might encounter situations where you need to generate a dictionary from specific columns based on the position of their values. This can be particularly useful when you want to categorize or summarize your data in a structured format. In this guide, we'll walk you through a solution that allows you to create such a dictionary efficiently.
Understanding the Problem
Let's say you have a Pandas DataFrame that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
This DataFrame contains three columns: C1, C2, and value. Your goal is to create a nested dictionary with the following structure:
[[See Video to Reveal this Text or Code Snippet]]
This structure encapsulates the indices of the values corresponding to each unique character in columns C1 and C2, with the respective values associated with them.
Solution Overview
To achieve the desired output, we can utilize the groupby functionality available in Pandas along with a nested dictionary comprehension. This is a more efficient way of generating the dictionary than manually looping through each entry.
Step-by-Step Breakdown
Grouping the Data: We start by grouping the DataFrame by each column (C1 and C2), which allows us to organize data according to unique entries in those columns.
Generating the Dictionary: Using a nested dictionary comprehension, we will scrutinize each group and create the inner dictionary that stores the corresponding values.
Here's how the solution looks in code:
[[See Video to Reveal this Text or Code Snippet]]
Understanding the Code
dict(enumerate(g["value"], 1)): This constructs the inner dictionary where enumerate is used to generate index-value pairs starting at 1.
The resulting dictionary d: After executing the code, it will generate the desired nested dictionary.
Example Output
When you run the above code, the output will be:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Creating a dictionary from multiple columns in a Pandas DataFrame can seem challenging at first, but with the power of groupby and dictionary comprehensions, the task becomes straightforward and efficient. This approach is not only elegant but also enhances the performance and clarity of your code.
Now that you have a firm understanding of how to tackle this problem, feel free to implement this technique in your data manipulation tasks! If you have any questions or additional insights, please share them in the comments below.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Create dictionary from several columns based on position of values
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Dictionary from Multiple Columns in a Pandas DataFrame
When working with data in Pandas, you might encounter situations where you need to generate a dictionary from specific columns based on the position of their values. This can be particularly useful when you want to categorize or summarize your data in a structured format. In this guide, we'll walk you through a solution that allows you to create such a dictionary efficiently.
Understanding the Problem
Let's say you have a Pandas DataFrame that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
This DataFrame contains three columns: C1, C2, and value. Your goal is to create a nested dictionary with the following structure:
[[See Video to Reveal this Text or Code Snippet]]
This structure encapsulates the indices of the values corresponding to each unique character in columns C1 and C2, with the respective values associated with them.
Solution Overview
To achieve the desired output, we can utilize the groupby functionality available in Pandas along with a nested dictionary comprehension. This is a more efficient way of generating the dictionary than manually looping through each entry.
Step-by-Step Breakdown
Grouping the Data: We start by grouping the DataFrame by each column (C1 and C2), which allows us to organize data according to unique entries in those columns.
Generating the Dictionary: Using a nested dictionary comprehension, we will scrutinize each group and create the inner dictionary that stores the corresponding values.
Here's how the solution looks in code:
[[See Video to Reveal this Text or Code Snippet]]
Understanding the Code
dict(enumerate(g["value"], 1)): This constructs the inner dictionary where enumerate is used to generate index-value pairs starting at 1.
The resulting dictionary d: After executing the code, it will generate the desired nested dictionary.
Example Output
When you run the above code, the output will be:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Creating a dictionary from multiple columns in a Pandas DataFrame can seem challenging at first, but with the power of groupby and dictionary comprehensions, the task becomes straightforward and efficient. This approach is not only elegant but also enhances the performance and clarity of your code.
Now that you have a firm understanding of how to tackle this problem, feel free to implement this technique in your data manipulation tasks! If you have any questions or additional insights, please share them in the comments below.