filmov
tv
Understanding Segmentation Faults in C: Fixing Pointer Issues in Structs
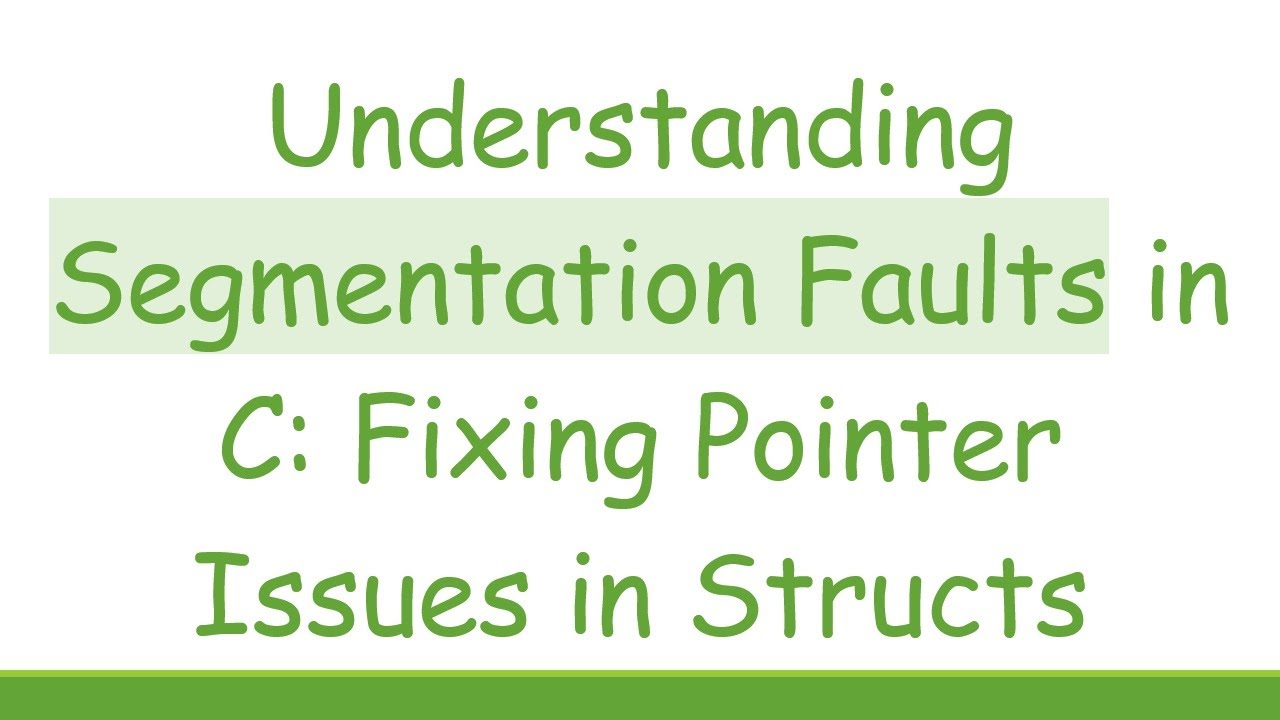
Показать описание
A detailed guide on resolving segmentation fault exceptions when dealing with pointers in C structs, specifically focusing on input handling and memory management.
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Getting segmentation fault exception when trying to write data inside a pointer
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Segmentation Faults in C: Fixing Pointer Issues in Structs
When programming in C, it’s not uncommon to encounter the dreaded segmentation fault exception. This issue often arises when your code tries to access an area of memory that it shouldn't. One scenario that frequently leads to such errors is improper handling of pointers, particularly when dealing with structs. In this guide, we’ll delve into a specific case that many developers, especially beginners, face and offer clear solutions to resolve the segmentation fault.
The Problem: Segmentation Fault During Input
In the code example below, a segmentation fault occurs when the program attempts to write data to a pointer within a struct. This issue manifests itself when the programmer is entering a brand name for a car. Below is a relevant snippet from the code:
[[See Video to Reveal this Text or Code Snippet]]
The segmentation fault is thrown during the execution of the scanf function, which indicates there's a problem with how memory is managed for the brand field inside the Car struct.
Breakdown: Understanding the Code Structure
Before jumping into the solution, let's clarify the relevant parts of the code to understand where things might go wrong:
Struct Definition
[[See Video to Reveal this Text or Code Snippet]]
Here, the brand is defined as a pointer to a character array, which means it can hold a string.
Memory Allocation
[[See Video to Reveal this Text or Code Snippet]]
Memory is allocated for the brand pointer, which is great for storing strings up to 99 characters plus the null terminator.
Input Handling
[[See Video to Reveal this Text or Code Snippet]]
This is where the issue arises. The use of the dereference operator * is incorrect because brand is already a pointer. Using * leads to undefined behavior, likely causing a segmentation fault.
Solution: Correcting the Input Method
Correcting scanf for String Input
To fix this issue, we need to adjust how we capture input into the brand. The corrected version should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Here, %99s ensures that we read a string of up to 99 characters, ensuring we don't overflow the allocated memory.
Removing the Unnecessary Dereference
You should also remove the dereference operator in any subsequent checks on the brand:
Instead of:
[[See Video to Reveal this Text or Code Snippet]]
Use:
[[See Video to Reveal this Text or Code Snippet]]
This checks only the first character of the brand string, which is the intended validation.
Avoiding Memory Errors
Finally, it’s crucial to avoid freeing memory while still using it. Remove the free(tab[i].brand); call inside the loop that stores information about the car. Instead, it should only be called once you are completely done using the allocated memory.
Revised Input Handling Example
Here’s how the revised input part should look:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Segmentation faults due to pointer misuse can be frustrating, but they're often easy to resolve with a few tweaks to your code. By ensuring that you correctly handle memory allocation and input without unnecessary dereferences, you can avoid these pitfalls in your C programming. Continue to practice managing pointers and structs, and you'll master them in no time!
By following these guidelines, you should be able to troubleshoot and fix your segmentation faults effectively. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Getting segmentation fault exception when trying to write data inside a pointer
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Segmentation Faults in C: Fixing Pointer Issues in Structs
When programming in C, it’s not uncommon to encounter the dreaded segmentation fault exception. This issue often arises when your code tries to access an area of memory that it shouldn't. One scenario that frequently leads to such errors is improper handling of pointers, particularly when dealing with structs. In this guide, we’ll delve into a specific case that many developers, especially beginners, face and offer clear solutions to resolve the segmentation fault.
The Problem: Segmentation Fault During Input
In the code example below, a segmentation fault occurs when the program attempts to write data to a pointer within a struct. This issue manifests itself when the programmer is entering a brand name for a car. Below is a relevant snippet from the code:
[[See Video to Reveal this Text or Code Snippet]]
The segmentation fault is thrown during the execution of the scanf function, which indicates there's a problem with how memory is managed for the brand field inside the Car struct.
Breakdown: Understanding the Code Structure
Before jumping into the solution, let's clarify the relevant parts of the code to understand where things might go wrong:
Struct Definition
[[See Video to Reveal this Text or Code Snippet]]
Here, the brand is defined as a pointer to a character array, which means it can hold a string.
Memory Allocation
[[See Video to Reveal this Text or Code Snippet]]
Memory is allocated for the brand pointer, which is great for storing strings up to 99 characters plus the null terminator.
Input Handling
[[See Video to Reveal this Text or Code Snippet]]
This is where the issue arises. The use of the dereference operator * is incorrect because brand is already a pointer. Using * leads to undefined behavior, likely causing a segmentation fault.
Solution: Correcting the Input Method
Correcting scanf for String Input
To fix this issue, we need to adjust how we capture input into the brand. The corrected version should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Here, %99s ensures that we read a string of up to 99 characters, ensuring we don't overflow the allocated memory.
Removing the Unnecessary Dereference
You should also remove the dereference operator in any subsequent checks on the brand:
Instead of:
[[See Video to Reveal this Text or Code Snippet]]
Use:
[[See Video to Reveal this Text or Code Snippet]]
This checks only the first character of the brand string, which is the intended validation.
Avoiding Memory Errors
Finally, it’s crucial to avoid freeing memory while still using it. Remove the free(tab[i].brand); call inside the loop that stores information about the car. Instead, it should only be called once you are completely done using the allocated memory.
Revised Input Handling Example
Here’s how the revised input part should look:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Segmentation faults due to pointer misuse can be frustrating, but they're often easy to resolve with a few tweaks to your code. By ensuring that you correctly handle memory allocation and input without unnecessary dereferences, you can avoid these pitfalls in your C programming. Continue to practice managing pointers and structs, and you'll master them in no time!
By following these guidelines, you should be able to troubleshoot and fix your segmentation faults effectively. Happy coding!