filmov
tv
WHY did this C++ code FAIL?
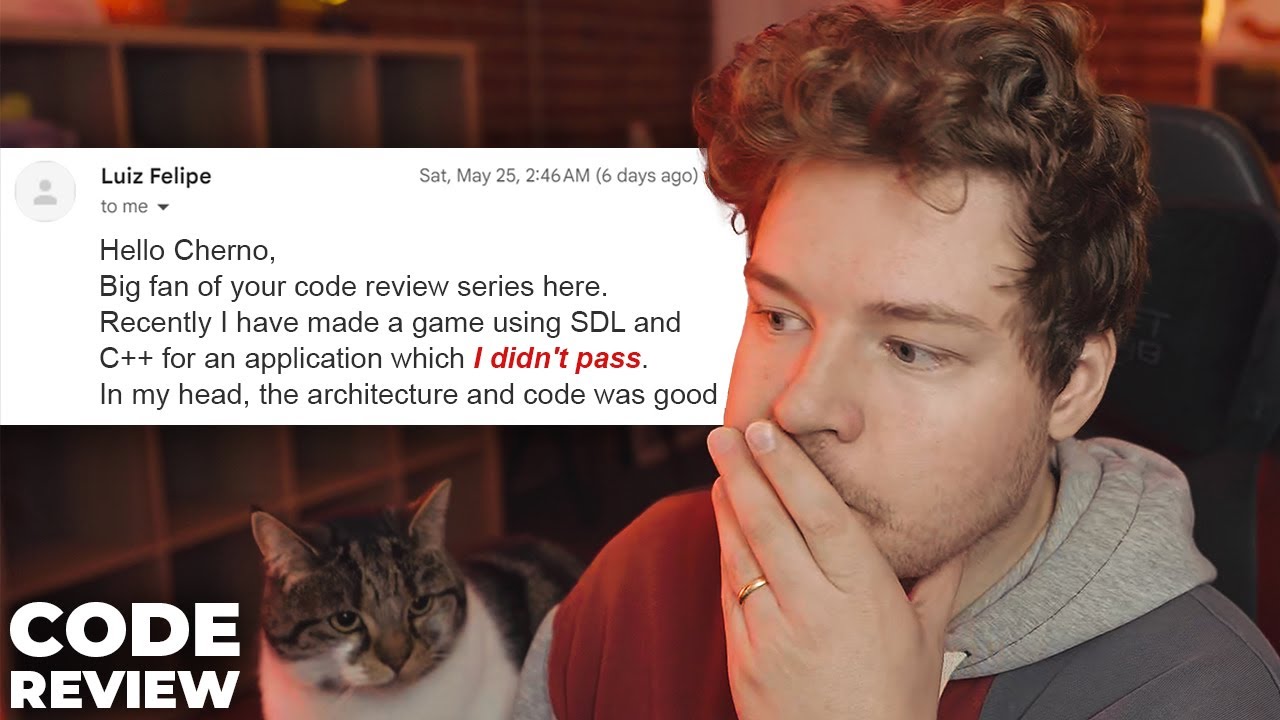
Показать описание
📚 CHAPTERS
0:00 - Hello
1:29 - ALWAYS stack allocate if you can
4:20 - The cat is back
4:36 - API design considerations
7:27 - return 0 in the main function
8:31 - Organization and code conventions
11:22 - Variable intialization
14:44 - Deep class hierarchies
18:12 - Managing states
20:58 - defines
25:32 - Avoid copying causing unnessary heap allocations
26:38 - More on #define
28:50 - Consistent code style
30:33 - Compilation warnings
31:13 - Logging and release builds
32:30 - struct vs class
33:16 - Cleaner code
35:21 - Final thoughts and conclusion
36:48 - Use std::weak_ptr
💰 Links to stuff I use:
This video is sponsored by Brilliant.
coding in c until my program is unsafe
Understanding C program Compilation Process
How I program C
Satisfying ascii animation with C 😉 - The doughnut shaped code that generates a spinning 🍩
What does this weird C program do?
Is C BETTER than C++ for beginners? // Code Review
Why do C Programmers Always Obfuscate Their Code?
I LOVE YOU program in C Language || #shorts || #CloudCODE
C Program to swap two numbers using function call by value #cprogramming #coding #code.
C Language Source Code to Exe | Build Process | Compilation, PreProcessor ( Theory )
Why #include? | Why int main() | Why return 0 | Simple C Program | Log2Base2
Rust: When C Code Isn't Enough
How to run C program in command prompt
How to Set up Visual Studio Code for C and C++ Programming
C vs Python Speed Test #cpp #python #programming #code
How to Set up Visual Studio Code for C and C++ Programming [ 2024]
funny program in c|send this WhatsApp group #shorts#programming#coding#cprogramming#funny#clanguage
FIX: C/C++ PROGRAMS NOT RUNNING PROPERLY ON VS CODE (EASY FIX) | 100% WORKING
How is THIS valid c# code??
How to Set up Visual Studio Code for C and C++ Programming
Student Grade Calculation using Nested if else: C Program
C_06 Execution Process of a C Program | C Programming Tutorials
Donut-shaped C code that generates a 3D spinning donut
coding in c until my program crashes
Комментарии