filmov
tv
How to Remove the Last 2,3,4,N Elements from an Array in JavaScript
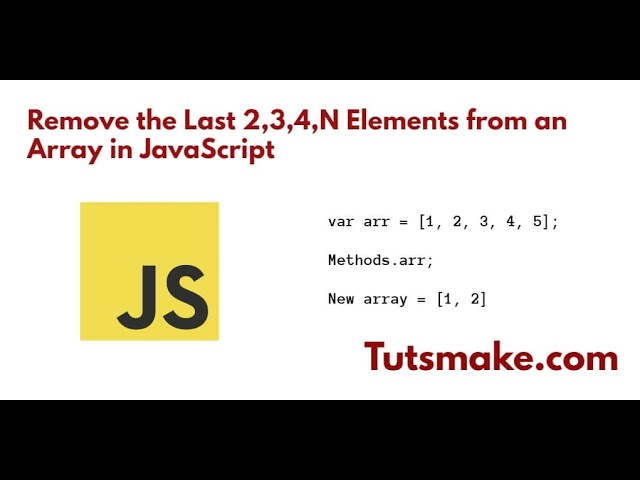
Показать описание
In JavaScript, manipulating arrays is a common task, and there are various ways to remove the last 2, 3, 4, or N elements. This tutorial will explore simple methods to achieve this flexibility.
Method 1: Using splice()
The splice() method allows for precise modifications to an array by specifying the starting index and the number of elements to remove.
function removeLastNElementsWithSplice(arr, n) {
// Use splice to remove the last n elements
}
// Example usage:
let myArray = [1, 2, 3, 4, 5, 6, 7];
removeLastNElementsWithSplice(myArray, 3);
Method 2: Using array slicing
The slice() method is versatile and allows you to create a new array by specifying the range of elements to include.
function removeLastNElementsWithSlice(arr, n) {
// Create a new array without the last n elements
return newArray;
}
// Example usage:
let myArray = [1, 2, 3, 4, 5, 6, 7];
let newArray = removeLastNElementsWithSlice(myArray, 3);
Method 3: Using Loop with pop()
You can use a loop with the pop() method to iteratively remove the last N elements in place.
function removeLastNElementsWithPop(arr, n) {
// Remove the last N elements using pop() in a loop
for (let i = 0; i = n; i++) {
}
}
// Example usage:
let myArray = [1, 2, 3, 4, 5, 6, 7];
removeLastNElementsWithPop(myArray, 3);
#ReadMore
#javascript #remove #delete #last #2 #3 #4 #N #element #item #from #array #shift #pop #filter #slice #splice #javascript_projects #javascript_tutorial #javascriptintamil #javascriptengineer #javascript_project #javascriptinbengali #javascriptinterview #javascriptinterviewquestions #javascripttutorials #javascripttutorial #javascripttips #javascripttricks #javascripttraining #javascriptlearning #javascriptcode #javascriptcoding
remove last 2 element from an array javascript
remove last 3 element from an array javascript
remove last 4 element from an array javascript
remove last N element from an array javascript
how to remove last 2,3 N element from an array javascript
How to remove n elements from the end of a given array in JavaScript
Remove last or last N elements from an Array in JavaScript
Removing the Last 3 Elements of an Array in JavaScript
Method 1: Using splice()
The splice() method allows for precise modifications to an array by specifying the starting index and the number of elements to remove.
function removeLastNElementsWithSplice(arr, n) {
// Use splice to remove the last n elements
}
// Example usage:
let myArray = [1, 2, 3, 4, 5, 6, 7];
removeLastNElementsWithSplice(myArray, 3);
Method 2: Using array slicing
The slice() method is versatile and allows you to create a new array by specifying the range of elements to include.
function removeLastNElementsWithSlice(arr, n) {
// Create a new array without the last n elements
return newArray;
}
// Example usage:
let myArray = [1, 2, 3, 4, 5, 6, 7];
let newArray = removeLastNElementsWithSlice(myArray, 3);
Method 3: Using Loop with pop()
You can use a loop with the pop() method to iteratively remove the last N elements in place.
function removeLastNElementsWithPop(arr, n) {
// Remove the last N elements using pop() in a loop
for (let i = 0; i = n; i++) {
}
}
// Example usage:
let myArray = [1, 2, 3, 4, 5, 6, 7];
removeLastNElementsWithPop(myArray, 3);
#ReadMore
#javascript #remove #delete #last #2 #3 #4 #N #element #item #from #array #shift #pop #filter #slice #splice #javascript_projects #javascript_tutorial #javascriptintamil #javascriptengineer #javascript_project #javascriptinbengali #javascriptinterview #javascriptinterviewquestions #javascripttutorials #javascripttutorial #javascripttips #javascripttricks #javascripttraining #javascriptlearning #javascriptcode #javascriptcoding
remove last 2 element from an array javascript
remove last 3 element from an array javascript
remove last 4 element from an array javascript
remove last N element from an array javascript
how to remove last 2,3 N element from an array javascript
How to remove n elements from the end of a given array in JavaScript
Remove last or last N elements from an Array in JavaScript
Removing the Last 3 Elements of an Array in JavaScript