filmov
tv
Creating and using objects in TypeScript - #12 #TypeScriptObjects#ObjectOrientedProgramming
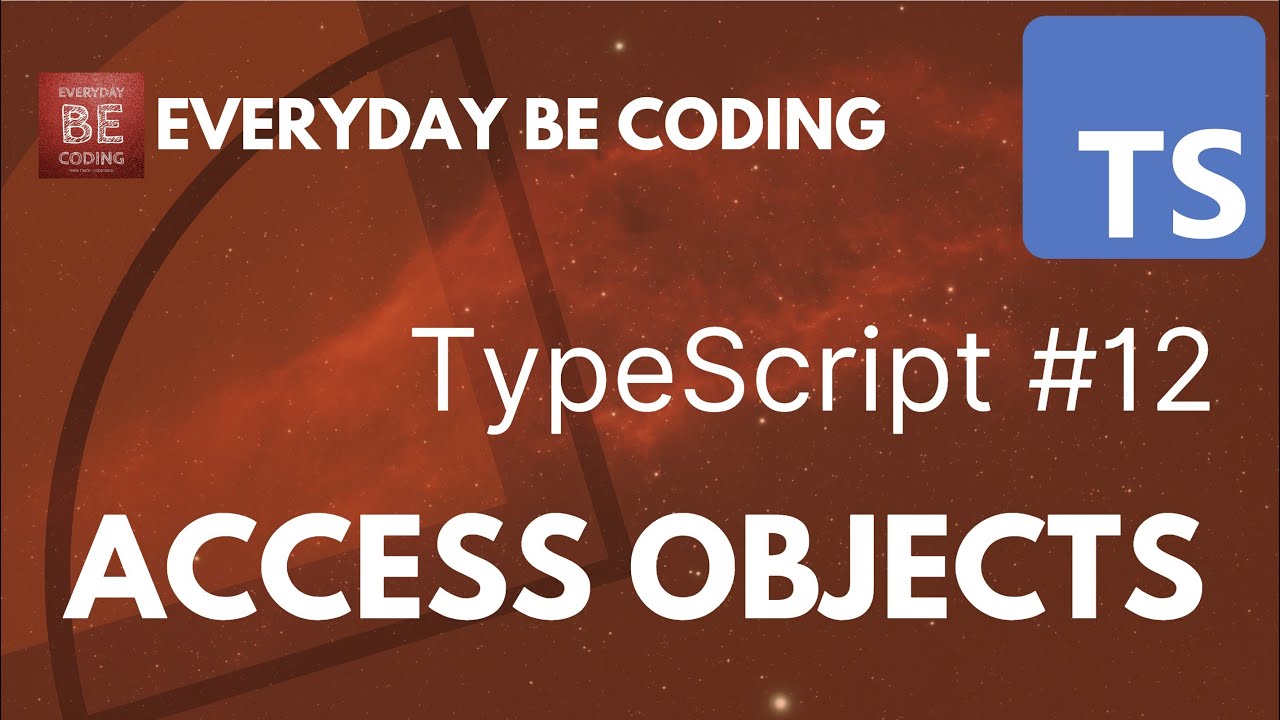
Показать описание
#techtutorial #TypeScript #JavaScript #WebDevelopment #Coding #Programming #TypeScriptTips #LearnTypeScript #TypeScriptTutorial
#FrontendDevelopment #CodeNewbie #DevCommunity
#SoftwareDevelopment #TypeScriptObjects #ObjectOrientedProgramming #TypeScriptLearning #CodeLearning #ProgrammingLanguages #TypeScriptDev #CodeEducation
#WebDevTips #FullStackDevelopment #TypeScriptCommunity
#CodeSharing #TypeScriptBeginner #TypeScriptAdvanced
#TechEducation #JavaScriptFrameworks #TypeSafety
#CodeWithMe #CodingJourney #TypeScriptProgramming
#TypeScriptCoders #TypeScriptForBeginners #TypeScriptExamples
Creating and using objects in TypeScript involves defining types or interfaces, creating instances, and using those instances. Here’s a guide to help you understand how to work with objects in TypeScript.
1. Defining Types and Interfaces
Types and interfaces are used to define the shape of an object.
Using Interfaces
Interfaces are often used to define the structure of an object.
typescript
Copy code
interface Person {
name: string;
age: number;
greet: () = void;
}
const person: Person = {
name: "Alice",
age: 30,
};
Using Type Aliases
Type aliases can also be used to define the shape of an object.
typescript code
type Car = {
brand: string;
model: string;
year: number;
};
const car: Car = {
brand: "Toyota",
model: "Corolla",
year: 2020,
};
2. Creating Objects
You can create objects directly or use classes to instantiate objects.
Direct Object Creation
Directly create objects by assigning values to a variable defined with a type or interface.
typescript code
const book: { title: string; author: string; pages: number } = {
title: "TypeScript Basics",
author: "John Doe",
pages: 200,
};
Using Classes
Classes provide a blueprint for creating objects with constructors and methods.
typescript code
class Animal {
constructor(public name: string, public species: string) {}
makeSound() {
}
}
const dog = new Animal("Buddy", "Dog");
3. Working with Objects
Once you have objects, you can access and modify their properties, and call their methods.
Accessing Properties
typescript code:
const user = {
username: "johndoe",
};
Modifying Properties
typescript
Copy code
Calling Methods
typescript code
const smartphone = {
brand: "Apple",
model: "iPhone 13",
ring() {
},
};
4. Optional Properties and Readonly Properties
You can define optional properties and readonly properties in interfaces and types.
Optional Properties
typescript code:
interface Employee {
id: number;
name: string;
department?: string; // Optional property
}
const emp1: Employee = { id: 1, name: "Alice" };
const emp2: Employee = { id: 2, name: "Bob", department: "HR" };
Readonly Properties
typescript code:
interface Product {
readonly id: number;
name: string;
}
const product: Product = { id: 101, name: "Laptop" };
5. Index Signatures
Index signatures allow you to define objects with dynamic property names.
typescript code:
interface Dictionary {
[key: string]: string;
}
const dictionary: Dictionary = {};
dictionary["hello"] = "A greeting";
dictionary["world"] = "The earth";
Conclusion
Creating and using objects in TypeScript is straightforward once you understand the basics of types, interfaces, and classes. This structure allows for robust type-checking and more maintainable code. By defining the shape of objects, you can leverage TypeScript’s static type-checking to catch errors early and ensure that your objects are used consistently throughout your code.
Chapter :
00:00 Introduction
00:09 Defining Types and Interfaces
01:10 Creating Objects
01:45 Working with Objects
02:01 Optional Properties and Read-only Properties
02:25 Index Signatures
02:37 Conclusion
Thank you for watching this video
Everyday Be coding
#FrontendDevelopment #CodeNewbie #DevCommunity
#SoftwareDevelopment #TypeScriptObjects #ObjectOrientedProgramming #TypeScriptLearning #CodeLearning #ProgrammingLanguages #TypeScriptDev #CodeEducation
#WebDevTips #FullStackDevelopment #TypeScriptCommunity
#CodeSharing #TypeScriptBeginner #TypeScriptAdvanced
#TechEducation #JavaScriptFrameworks #TypeSafety
#CodeWithMe #CodingJourney #TypeScriptProgramming
#TypeScriptCoders #TypeScriptForBeginners #TypeScriptExamples
Creating and using objects in TypeScript involves defining types or interfaces, creating instances, and using those instances. Here’s a guide to help you understand how to work with objects in TypeScript.
1. Defining Types and Interfaces
Types and interfaces are used to define the shape of an object.
Using Interfaces
Interfaces are often used to define the structure of an object.
typescript
Copy code
interface Person {
name: string;
age: number;
greet: () = void;
}
const person: Person = {
name: "Alice",
age: 30,
};
Using Type Aliases
Type aliases can also be used to define the shape of an object.
typescript code
type Car = {
brand: string;
model: string;
year: number;
};
const car: Car = {
brand: "Toyota",
model: "Corolla",
year: 2020,
};
2. Creating Objects
You can create objects directly or use classes to instantiate objects.
Direct Object Creation
Directly create objects by assigning values to a variable defined with a type or interface.
typescript code
const book: { title: string; author: string; pages: number } = {
title: "TypeScript Basics",
author: "John Doe",
pages: 200,
};
Using Classes
Classes provide a blueprint for creating objects with constructors and methods.
typescript code
class Animal {
constructor(public name: string, public species: string) {}
makeSound() {
}
}
const dog = new Animal("Buddy", "Dog");
3. Working with Objects
Once you have objects, you can access and modify their properties, and call their methods.
Accessing Properties
typescript code:
const user = {
username: "johndoe",
};
Modifying Properties
typescript
Copy code
Calling Methods
typescript code
const smartphone = {
brand: "Apple",
model: "iPhone 13",
ring() {
},
};
4. Optional Properties and Readonly Properties
You can define optional properties and readonly properties in interfaces and types.
Optional Properties
typescript code:
interface Employee {
id: number;
name: string;
department?: string; // Optional property
}
const emp1: Employee = { id: 1, name: "Alice" };
const emp2: Employee = { id: 2, name: "Bob", department: "HR" };
Readonly Properties
typescript code:
interface Product {
readonly id: number;
name: string;
}
const product: Product = { id: 101, name: "Laptop" };
5. Index Signatures
Index signatures allow you to define objects with dynamic property names.
typescript code:
interface Dictionary {
[key: string]: string;
}
const dictionary: Dictionary = {};
dictionary["hello"] = "A greeting";
dictionary["world"] = "The earth";
Conclusion
Creating and using objects in TypeScript is straightforward once you understand the basics of types, interfaces, and classes. This structure allows for robust type-checking and more maintainable code. By defining the shape of objects, you can leverage TypeScript’s static type-checking to catch errors early and ensure that your objects are used consistently throughout your code.
Chapter :
00:00 Introduction
00:09 Defining Types and Interfaces
01:10 Creating Objects
01:45 Working with Objects
02:01 Optional Properties and Read-only Properties
02:25 Index Signatures
02:37 Conclusion
Thank you for watching this video
Everyday Be coding
Комментарии