filmov
tv
How to Post an Array with Objects in Angular
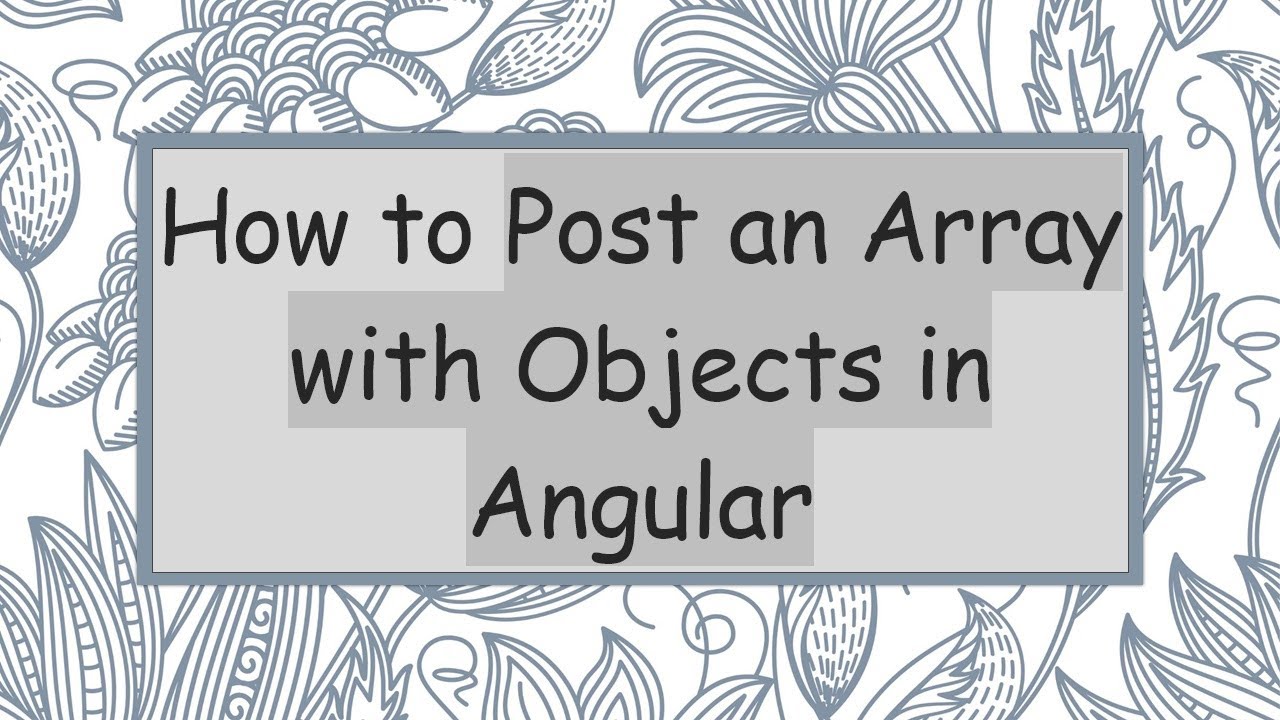
Показать описание
A comprehensive guide on how to post an array containing objects in Angular, using TypeScript to transform your data.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: how to post an array with some object when I have an array with id, using angular
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Post an Array with Objects in Angular
When building applications using Angular, you may often find yourself needing to send data to a backend API. One common scenario is posting an array of objects, especially if you have an array of IDs and you want to structure that data properly. This guide will walk you through how to achieve this in a clear and concise way.
The Challenge
Imagine you have selected multiple jobs, and each job has a unique identifier (ID). You wish to format this data for posting: every job ID should be an individual object with a specific structure that includes a state. Let's look at how you can transform your array of job IDs into the desired format.
Given Code Snippet
You start with this initial code, which collects the selected job IDs into an array:
[[See Video to Reveal this Text or Code Snippet]]
This results in an array like this:
[[See Video to Reveal this Text or Code Snippet]]
Next, you create a payload but notice that its structure isn't what you need:
[[See Video to Reveal this Text or Code Snippet]]
This payload appears as follows:
[[See Video to Reveal this Text or Code Snippet]]
However, you need a payload structured like this:
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To achieve the desired structure for your payload, you can utilize the map function in TypeScript. Here’s how to proceed:
Step-by-Step Transformation
Map the Job IDs to Required Object Structure:
Instead of creating a single object with an array of IDs, you need to create an object for each job ID.
Updated Code Implementation:
Replace your previous payload creation with the following code snippet:
[[See Video to Reveal this Text or Code Snippet]]
This code will iterate over each job ID in selectedJobsId and return a corresponding object with the required properties.
Resulting Payload
The output of the above transformation will give you a properly structured array:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In Angular, structuring your data correctly for API POST requests is critical for effective communication with your backend services. By utilizing the map function in TypeScript, you can convert an array of IDs into a well-structured array of objects, making your data handling straightforward and efficient.
Next time you face a task of formatting your data for posting, remember this approach! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: how to post an array with some object when I have an array with id, using angular
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Post an Array with Objects in Angular
When building applications using Angular, you may often find yourself needing to send data to a backend API. One common scenario is posting an array of objects, especially if you have an array of IDs and you want to structure that data properly. This guide will walk you through how to achieve this in a clear and concise way.
The Challenge
Imagine you have selected multiple jobs, and each job has a unique identifier (ID). You wish to format this data for posting: every job ID should be an individual object with a specific structure that includes a state. Let's look at how you can transform your array of job IDs into the desired format.
Given Code Snippet
You start with this initial code, which collects the selected job IDs into an array:
[[See Video to Reveal this Text or Code Snippet]]
This results in an array like this:
[[See Video to Reveal this Text or Code Snippet]]
Next, you create a payload but notice that its structure isn't what you need:
[[See Video to Reveal this Text or Code Snippet]]
This payload appears as follows:
[[See Video to Reveal this Text or Code Snippet]]
However, you need a payload structured like this:
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To achieve the desired structure for your payload, you can utilize the map function in TypeScript. Here’s how to proceed:
Step-by-Step Transformation
Map the Job IDs to Required Object Structure:
Instead of creating a single object with an array of IDs, you need to create an object for each job ID.
Updated Code Implementation:
Replace your previous payload creation with the following code snippet:
[[See Video to Reveal this Text or Code Snippet]]
This code will iterate over each job ID in selectedJobsId and return a corresponding object with the required properties.
Resulting Payload
The output of the above transformation will give you a properly structured array:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In Angular, structuring your data correctly for API POST requests is critical for effective communication with your backend services. By utilizing the map function in TypeScript, you can convert an array of IDs into a well-structured array of objects, making your data handling straightforward and efficient.
Next time you face a task of formatting your data for posting, remember this approach! Happy coding!