filmov
tv
Longest Common Subsequence | Recursion | Memo | Optimal | Leetcode 1143
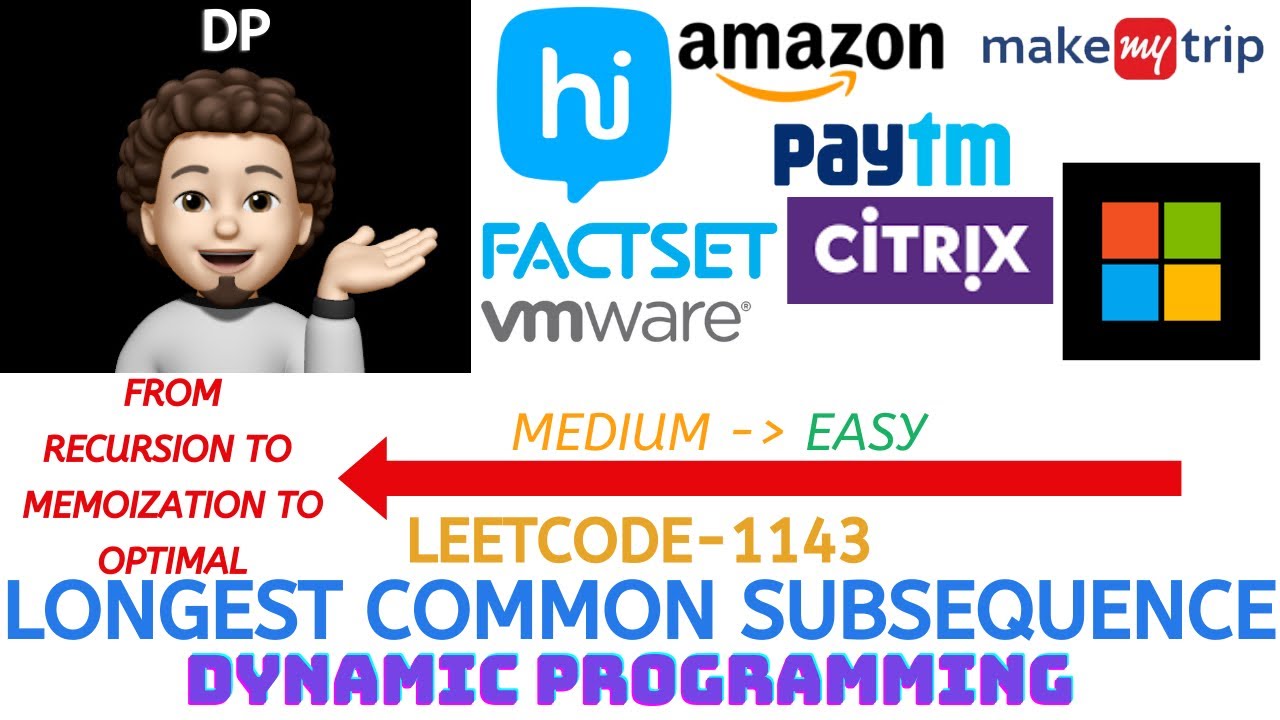
Показать описание
This is the 10th Video of our Playlist "Dynamic Programming : Popular Interview Problems".
In this video we will try to solve a very good and famous DP problem - Longest Common Subsequence (Leetcode 1143)
I will explain the intuition so easily that you will never forget and start seeing this as cakewalk EASYYY.
We will do live coding after explanation and see if we are able to pass all the test cases.
Also, please note that my Github solution link below contains both C++ as well as JAVA code.
Problem Name : Longest Common Subsequence (Leetcode 1143)
Company Tags : Microsoft, Amazon, FactSet, Hike, Citrix, MakeMyTrip, Paytm, VMWare
Approach-1 Summary : The approach uses a recursive approach with memoization to avoid redundant computations. The memoization is achieved by maintaining a 2D array t of size 1001x1001, initialized with -1. The function LCS recursively computes the length of the LCS by comparing characters of the two strings. If a subproblem's result is already memoized, it is returned directly; otherwise, the LCS is calculated, and the result is stored in the memoization table. The final result is obtained by calling the LCS function with the lengths of the input strings and initializing the memoization table before starting the computation.
Approach-2 Summary : The approach uses dynamic programming with a 2D vector t to store intermediate results. The code iterates through all possible pairs of indices, filling the table based on the recurrence relation for LCS. The final result is the length of the LCS for the entire input strings, stored in t[m][n], where m and n are the lengths of text1 and text2, respectively.
Approach-3 Summary : It utilizes a 2-row 2D vector (t) to store intermediate results, reducing space complexity. The code iterates through the strings, updating the table based on the LCS recurrence relation. The final result is stored in t[m % 2][n], where m and n are the lengths of the input strings. This optimization maintains the same functionality as the original code but with reduced memory usage.
╔═╦╗╔╦╗╔═╦═╦╦╦╦╗╔═╗
║╚╣║║║╚╣╚╣╔╣╔╣║╚╣═╣
╠╗║╚╝║║╠╗║╚╣║║║║║═╣
╚═╩══╩═╩═╩═╩╝╚╩═╩═╝
✨ Timelines✨
00:00 - Introduction
#coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge #leetcodequestions #leetcodechallenge #hindi #india #coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge#leetcodequestions #leetcodechallenge #hindi #india #hindiexplanation #hindiexplained #easyexplaination #interview#interviewtips #interviewpreparation #interview_ds_algo #hinglish #github #design #data #google #video #instagram #facebook #leetcode #computerscience #leetcodesolutions #leetcodequestionandanswers #code #learning #dsalgo #dsa #2024 #newyear
In this video we will try to solve a very good and famous DP problem - Longest Common Subsequence (Leetcode 1143)
I will explain the intuition so easily that you will never forget and start seeing this as cakewalk EASYYY.
We will do live coding after explanation and see if we are able to pass all the test cases.
Also, please note that my Github solution link below contains both C++ as well as JAVA code.
Problem Name : Longest Common Subsequence (Leetcode 1143)
Company Tags : Microsoft, Amazon, FactSet, Hike, Citrix, MakeMyTrip, Paytm, VMWare
Approach-1 Summary : The approach uses a recursive approach with memoization to avoid redundant computations. The memoization is achieved by maintaining a 2D array t of size 1001x1001, initialized with -1. The function LCS recursively computes the length of the LCS by comparing characters of the two strings. If a subproblem's result is already memoized, it is returned directly; otherwise, the LCS is calculated, and the result is stored in the memoization table. The final result is obtained by calling the LCS function with the lengths of the input strings and initializing the memoization table before starting the computation.
Approach-2 Summary : The approach uses dynamic programming with a 2D vector t to store intermediate results. The code iterates through all possible pairs of indices, filling the table based on the recurrence relation for LCS. The final result is the length of the LCS for the entire input strings, stored in t[m][n], where m and n are the lengths of text1 and text2, respectively.
Approach-3 Summary : It utilizes a 2-row 2D vector (t) to store intermediate results, reducing space complexity. The code iterates through the strings, updating the table based on the LCS recurrence relation. The final result is stored in t[m % 2][n], where m and n are the lengths of the input strings. This optimization maintains the same functionality as the original code but with reduced memory usage.
╔═╦╗╔╦╗╔═╦═╦╦╦╦╗╔═╗
║╚╣║║║╚╣╚╣╔╣╔╣║╚╣═╣
╠╗║╚╝║║╠╗║╚╣║║║║║═╣
╚═╩══╩═╩═╩═╩╝╚╩═╩═╝
✨ Timelines✨
00:00 - Introduction
#coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge #leetcodequestions #leetcodechallenge #hindi #india #coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge#leetcodequestions #leetcodechallenge #hindi #india #hindiexplanation #hindiexplained #easyexplaination #interview#interviewtips #interviewpreparation #interview_ds_algo #hinglish #github #design #data #google #video #instagram #facebook #leetcode #computerscience #leetcodesolutions #leetcodequestionandanswers #code #learning #dsalgo #dsa #2024 #newyear
Комментарии