filmov
tv
How to Merge Two Sorted Arrays in Java Without Duplicates?
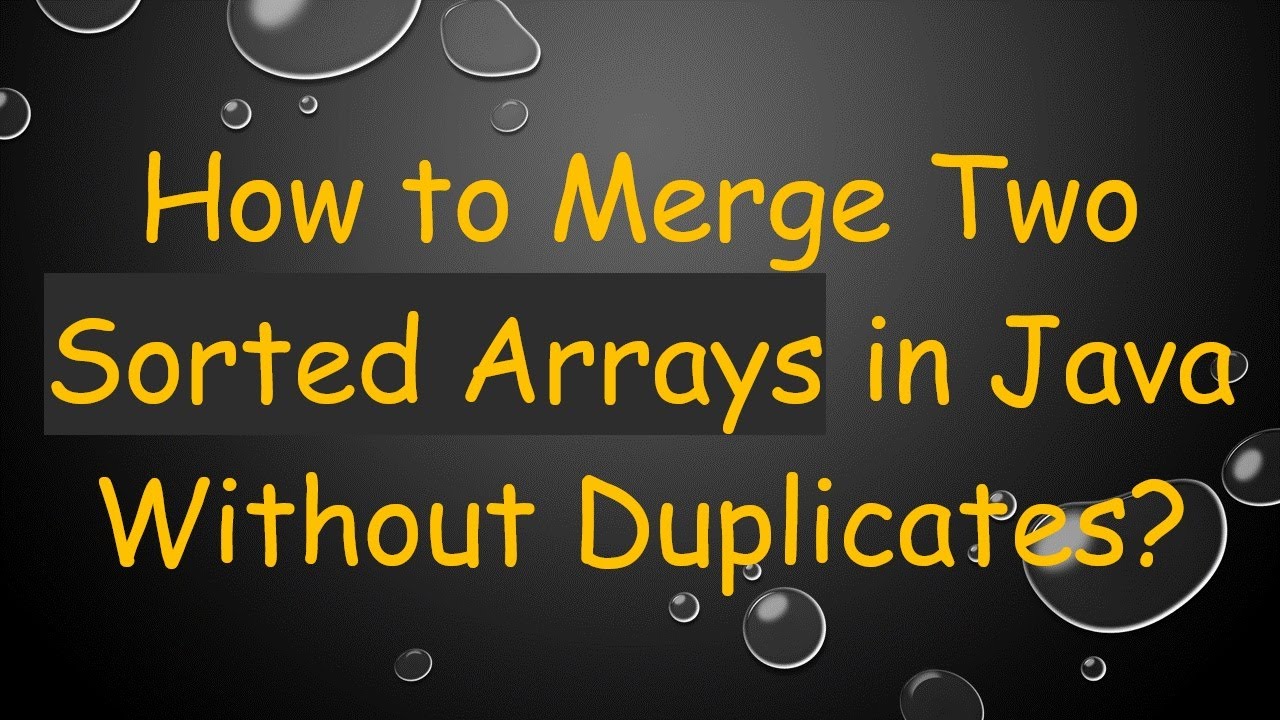
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to merge two sorted arrays in Java without introducing duplicates. This guide covers the essential steps and code examples for efficient merging.
---
How to Merge Two Sorted Arrays in Java Without Duplicates?
Merging two sorted arrays is a common problem that often appears in coding interviews and practical applications. While the task itself might sound straightforward, ensuring that there are no duplicates in the final merged array adds an additional layer of complexity. In this guide, we'll explore an efficient way to merge two sorted arrays in Java without duplicates.
Key Concepts
Before diving into the implementation, let's briefly discuss some key concepts:
Sorted Arrays: These are arrays where elements are arranged in a non-decreasing order.
Merging: Combining two arrays into one, while maintaining the sorted order.
Duplicates Removal: Ensuring that the merged array does not have any repeated elements.
Approach
Here's a step-by-step approach to solving the problem:
Initialization: Start by initializing pointers for both arrays and an empty list to store the merged elements.
Traverse and Compare: Traverse both arrays using the pointers, compare the elements, and add the smaller element to the merged list.
Skip Duplicates: While adding elements to the merged list, ensure that you skip any duplicates.
Add Remaining Elements: Once one of the arrays is exhausted, add the remaining elements from the other array, ensuring no duplicates are added.
Convert List to Array: Finally, convert the merged list back to an array if required.
Implementation in Java
Here is the Java code to merge two sorted arrays without duplicates:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
ArrayList Usage: An ArrayList is used to store the merged elements because it allows dynamic resizing.
addIfNotDuplicate() Method: This helper method ensures that only unique elements are added to the merged list.
Pointer Traversal: The pointers i and j traverse arr1 and arr2 respectively, ensuring that elements are added in a sorted manner and duplicates are skipped.
Conclusion
Merging two sorted arrays without duplicates in Java is a task that can be efficiently handled with careful pointer manipulation and duplicate checks. The implementation provided above ensures that the final merged array is both sorted and devoid of any duplicates.
Happy coding!
---
Summary: Learn how to merge two sorted arrays in Java without introducing duplicates. This guide covers the essential steps and code examples for efficient merging.
---
How to Merge Two Sorted Arrays in Java Without Duplicates?
Merging two sorted arrays is a common problem that often appears in coding interviews and practical applications. While the task itself might sound straightforward, ensuring that there are no duplicates in the final merged array adds an additional layer of complexity. In this guide, we'll explore an efficient way to merge two sorted arrays in Java without duplicates.
Key Concepts
Before diving into the implementation, let's briefly discuss some key concepts:
Sorted Arrays: These are arrays where elements are arranged in a non-decreasing order.
Merging: Combining two arrays into one, while maintaining the sorted order.
Duplicates Removal: Ensuring that the merged array does not have any repeated elements.
Approach
Here's a step-by-step approach to solving the problem:
Initialization: Start by initializing pointers for both arrays and an empty list to store the merged elements.
Traverse and Compare: Traverse both arrays using the pointers, compare the elements, and add the smaller element to the merged list.
Skip Duplicates: While adding elements to the merged list, ensure that you skip any duplicates.
Add Remaining Elements: Once one of the arrays is exhausted, add the remaining elements from the other array, ensuring no duplicates are added.
Convert List to Array: Finally, convert the merged list back to an array if required.
Implementation in Java
Here is the Java code to merge two sorted arrays without duplicates:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
ArrayList Usage: An ArrayList is used to store the merged elements because it allows dynamic resizing.
addIfNotDuplicate() Method: This helper method ensures that only unique elements are added to the merged list.
Pointer Traversal: The pointers i and j traverse arr1 and arr2 respectively, ensuring that elements are added in a sorted manner and duplicates are skipped.
Conclusion
Merging two sorted arrays without duplicates in Java is a task that can be efficiently handled with careful pointer manipulation and duplicate checks. The implementation provided above ensures that the final merged array is both sorted and devoid of any duplicates.
Happy coding!