filmov
tv
JAVA : What is a static variable in Java? SDET Automation Testing Interview Questions & Answers
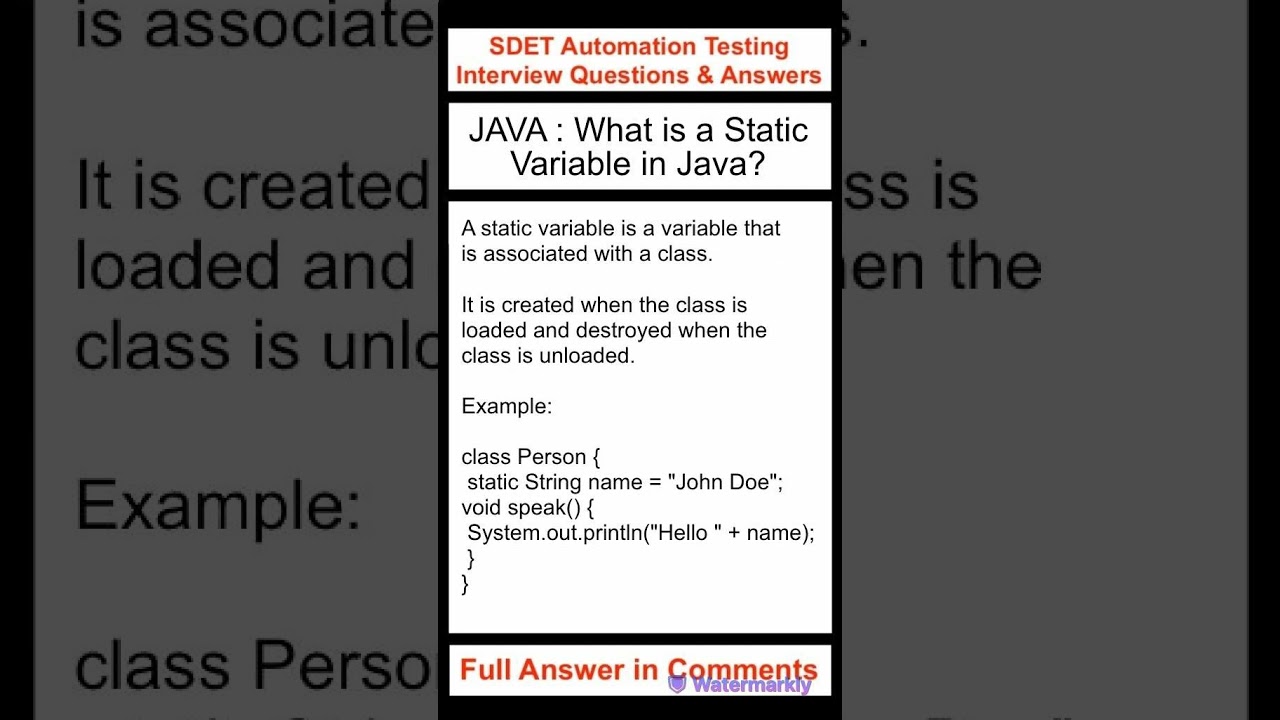
Показать описание
JAVA : What is a static variable in Java?
SDET Automation Testing Interview Questions & Answers
We will be covering a wide range of topics including QA manual testing, automation testing, Selenium, Java, Jenkins, Cucumber, Maven, and various testing frameworks.
JAVA : What is a static variable in Java?
In Java, a static variable is a class-level variable that belongs to the class itself, rather than to any particular instance of the class. It is also known as a class variable. Unlike instance variables, static variables are shared among all instances of a class. There is only one copy of a static variable, regardless of how many objects of that class are created.
Here are some key points about static variables:
1. Declaration: Static variables are declared within a class but outside any method, constructor, or instance block. They are typically declared at the top of the class and are marked with the `static` keyword.
2. Accessibility: Static variables can have different access modifiers (e.g., public, private, protected, or default) to control their visibility and access from other classes.
3. Initialization: Static variables can be initialized either directly at the point of declaration or within a static block of code. Static initialization blocks are executed when the class is loaded into memory.
4. Default values: If a static variable is not explicitly assigned a value, it will be assigned a default value based on its data type, similar to instance variables.
5. Lifetime: Static variables exist as long as the class is loaded into memory. They are shared among all instances of the class and can be accessed even without creating an object of the class.
6. Access within the class: Static variables can be accessed by any method or constructor within the class, including both static and non-static methods.
7. Access outside the class: The accessibility of static variables from outside the class depends on their access modifiers. Public static variables can be accessed directly using the class name, while private or default (package-private) static variables require accessor methods (getters and setters) or other public methods provided by the class.
Static variables are useful when you need to share data or maintain state across multiple instances of a class. They are commonly used for constants, global counters, shared resources, or configuration values that are common to all objects of a class.
Here's an example of declaring and using a static variable in Java:
public class MyClass {
private static int count; // Static variable
public void incrementCount() {
count++; // Accessing and modifying the static variable
}
public void displayCount() {
}
}
In the above example, the `count` variable is a static variable. It is shared among all instances of the `MyClass` class, and it can be accessed and modified through both static and non-static methods.
Remember that static variables should be used with caution, as they can introduce potential issues with thread safety and can make it more difficult to manage the state of your program.
SDET Automation Testing Interview Questions & Answers
We will be covering a wide range of topics including QA manual testing, automation testing, Selenium, Java, Jenkins, Cucumber, Maven, and various testing frameworks.
JAVA : What is a static variable in Java?
In Java, a static variable is a class-level variable that belongs to the class itself, rather than to any particular instance of the class. It is also known as a class variable. Unlike instance variables, static variables are shared among all instances of a class. There is only one copy of a static variable, regardless of how many objects of that class are created.
Here are some key points about static variables:
1. Declaration: Static variables are declared within a class but outside any method, constructor, or instance block. They are typically declared at the top of the class and are marked with the `static` keyword.
2. Accessibility: Static variables can have different access modifiers (e.g., public, private, protected, or default) to control their visibility and access from other classes.
3. Initialization: Static variables can be initialized either directly at the point of declaration or within a static block of code. Static initialization blocks are executed when the class is loaded into memory.
4. Default values: If a static variable is not explicitly assigned a value, it will be assigned a default value based on its data type, similar to instance variables.
5. Lifetime: Static variables exist as long as the class is loaded into memory. They are shared among all instances of the class and can be accessed even without creating an object of the class.
6. Access within the class: Static variables can be accessed by any method or constructor within the class, including both static and non-static methods.
7. Access outside the class: The accessibility of static variables from outside the class depends on their access modifiers. Public static variables can be accessed directly using the class name, while private or default (package-private) static variables require accessor methods (getters and setters) or other public methods provided by the class.
Static variables are useful when you need to share data or maintain state across multiple instances of a class. They are commonly used for constants, global counters, shared resources, or configuration values that are common to all objects of a class.
Here's an example of declaring and using a static variable in Java:
public class MyClass {
private static int count; // Static variable
public void incrementCount() {
count++; // Accessing and modifying the static variable
}
public void displayCount() {
}
}
In the above example, the `count` variable is a static variable. It is shared among all instances of the `MyClass` class, and it can be accessed and modified through both static and non-static methods.
Remember that static variables should be used with caution, as they can introduce potential issues with thread safety and can make it more difficult to manage the state of your program.
Комментарии