filmov
tv
How to Retrieve the Latest Message from WebSocket Queue in Python
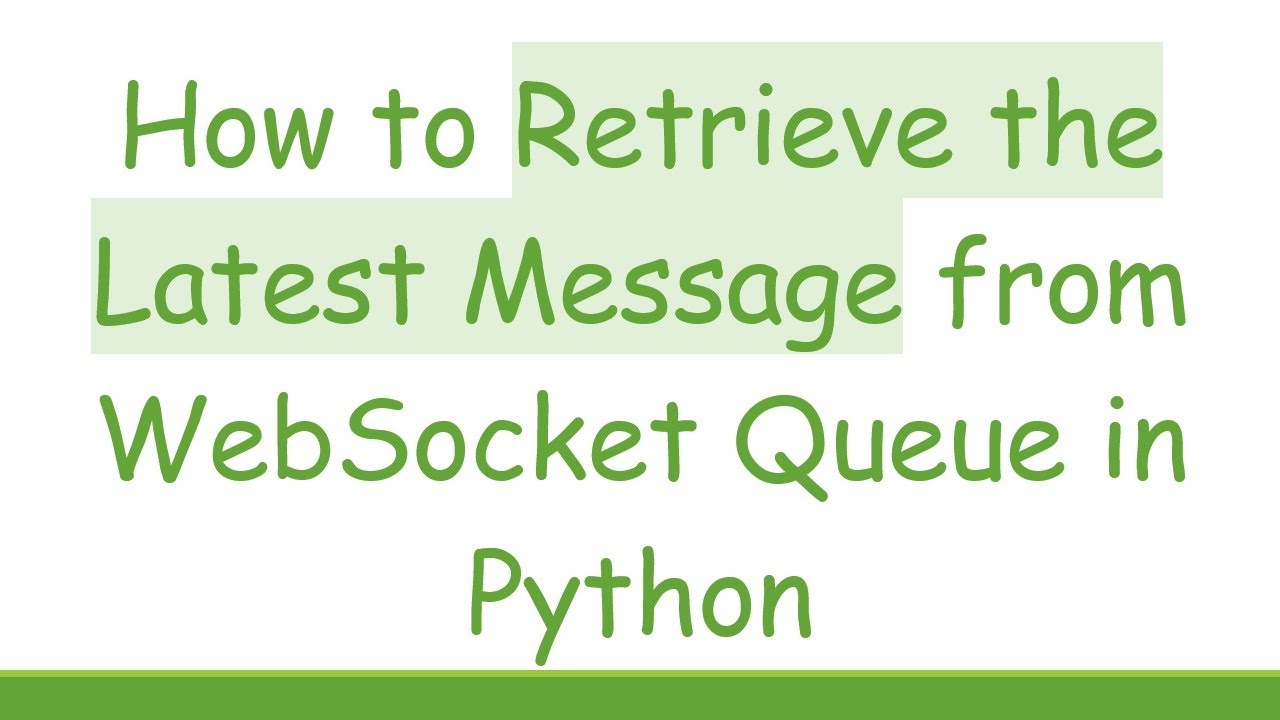
Показать описание
Learn how to efficiently get the latest message from the WebSocket queue in Python using asyncio. This guide also covers how to ignore unread messages for cleaner data handling.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Get latest message from websockets queue in Python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Retrieve the Latest Message from WebSocket Queue in Python
In the realm of real-time data communication, WebSockets are invaluable tools. They enable two-way communication between a client and server with low latency. However, one common challenge many developers encounter is how to efficiently retrieve only the latest message from a WebSocket queue while ignoring any unread messages that come in between. In this guide, we’ll explore how to achieve this using Python’s asyncio library.
The Problem: Retrieving the Latest Message
When utilizing WebSockets, messages can arrive rapidly, and clients may have a large stack of unread messages waiting to be processed. The requirement is simple yet crucial: you want to access only the most recent message and ignore the rest.
A Typical Approach
A common way to handle messages received from a WebSocket server is to use a continuous receiving loop like this:
[[See Video to Reveal this Text or Code Snippet]]
This approach may not suit scenarios where you only need the latest message while discarding any prior, unread messages.
The Solution: Using asyncio.LifoQueue
To efficiently retrieve the latest message while discarding previous ones, a LifoQueue from the asyncio library can be employed. This queue, which operates under a Last-In-First-Out (LIFO) principle, helps store messages and allows you to selectively process only the most recent one.
Implementation Steps
Set Up the LifoQueue: This queue will hold the incoming messages temporarily.
Create a Producer: This asynchronous function listens for incoming messages from the WebSocket server and places them in the queue.
Create a Consumer: This function processes messages from the queue. It retrieves the most recent message and clears the queue afterward to discard any old messages.
Here’s the Code to Implement This:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Code
Producer Function: This function runs continuously, listening for messages from the WebSocket server (ws_server) and placing each received message into the LifoQueue.
Consumer Function: This function runs in an infinite loop, waiting for messages in the queue. It retrieves the last message and uses a nested loop to empty the queue, ensuring all previous messages are discarded.
Conclusion
Managing WebSocket messaging can often feel complex, but with Python’s asyncio library and the right use of LifoQueue, fetching only the most recent message while ignoring others becomes straightforward. The code shared here will help streamline your WebSocket message handling, making your application more responsive and efficient.
Getting Started with Your WebSocket Implementation
If you're just jumping into working with WebSockets in Python, remember to install the required library:
[[See Video to Reveal this Text or Code Snippet]]
Now you’re equipped with both understanding and practical code to handle your WebSocket messages effectively! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Get latest message from websockets queue in Python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Retrieve the Latest Message from WebSocket Queue in Python
In the realm of real-time data communication, WebSockets are invaluable tools. They enable two-way communication between a client and server with low latency. However, one common challenge many developers encounter is how to efficiently retrieve only the latest message from a WebSocket queue while ignoring any unread messages that come in between. In this guide, we’ll explore how to achieve this using Python’s asyncio library.
The Problem: Retrieving the Latest Message
When utilizing WebSockets, messages can arrive rapidly, and clients may have a large stack of unread messages waiting to be processed. The requirement is simple yet crucial: you want to access only the most recent message and ignore the rest.
A Typical Approach
A common way to handle messages received from a WebSocket server is to use a continuous receiving loop like this:
[[See Video to Reveal this Text or Code Snippet]]
This approach may not suit scenarios where you only need the latest message while discarding any prior, unread messages.
The Solution: Using asyncio.LifoQueue
To efficiently retrieve the latest message while discarding previous ones, a LifoQueue from the asyncio library can be employed. This queue, which operates under a Last-In-First-Out (LIFO) principle, helps store messages and allows you to selectively process only the most recent one.
Implementation Steps
Set Up the LifoQueue: This queue will hold the incoming messages temporarily.
Create a Producer: This asynchronous function listens for incoming messages from the WebSocket server and places them in the queue.
Create a Consumer: This function processes messages from the queue. It retrieves the most recent message and clears the queue afterward to discard any old messages.
Here’s the Code to Implement This:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Code
Producer Function: This function runs continuously, listening for messages from the WebSocket server (ws_server) and placing each received message into the LifoQueue.
Consumer Function: This function runs in an infinite loop, waiting for messages in the queue. It retrieves the last message and uses a nested loop to empty the queue, ensuring all previous messages are discarded.
Conclusion
Managing WebSocket messaging can often feel complex, but with Python’s asyncio library and the right use of LifoQueue, fetching only the most recent message while ignoring others becomes straightforward. The code shared here will help streamline your WebSocket message handling, making your application more responsive and efficient.
Getting Started with Your WebSocket Implementation
If you're just jumping into working with WebSockets in Python, remember to install the required library:
[[See Video to Reveal this Text or Code Snippet]]
Now you’re equipped with both understanding and practical code to handle your WebSocket messages effectively! Happy coding!