filmov
tv
C Programming Tutorial for Beginners | C Programming Full Course
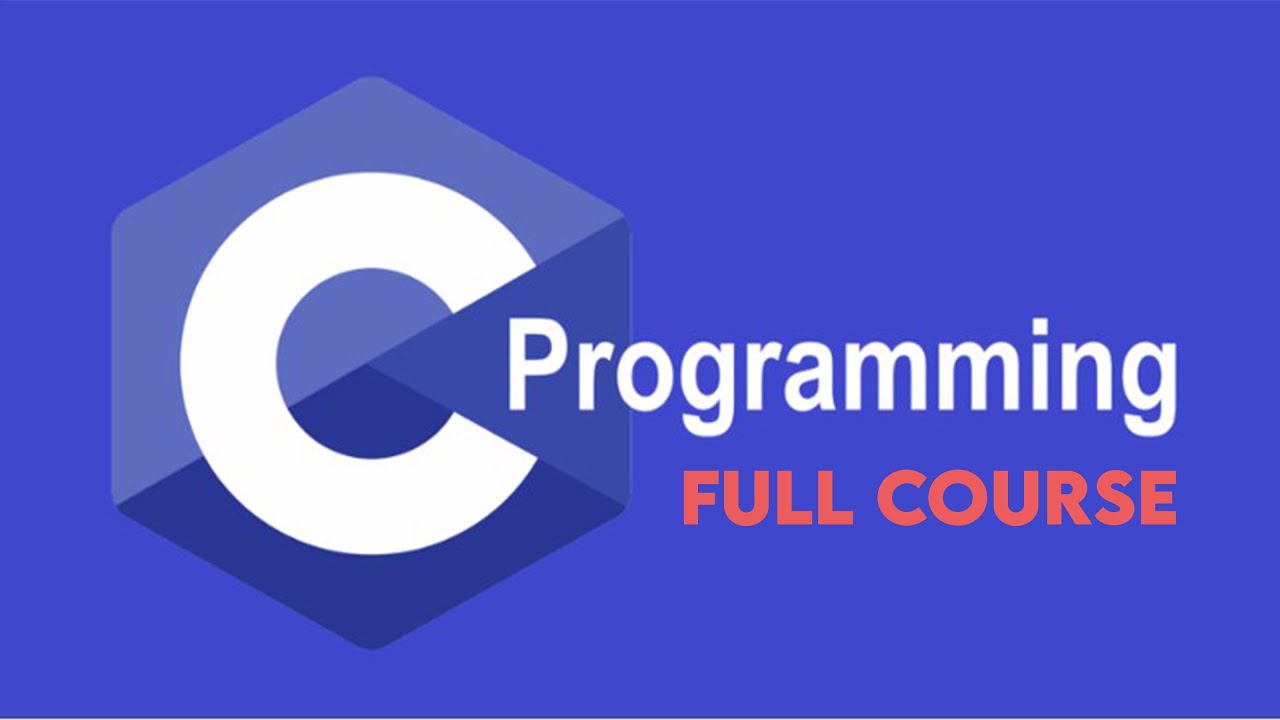
Показать описание
This specialization develops strong programming fundamentals for learners who want to solve complex problems by writing computer programs. Through four courses, you will learn to develop algorithms in a systematic way and read and write the C code to implement them. This will prepare you to pursue a career in software development or other computational fields.
Successful completion of this Specialization will be considered by admissions as a demonstration of your skill and enhance your master’s application to Duke’s Pratt School of Engineering.
⭐⭐⭐⭐🕑TIME STAMP📋⭐⭐⭐⭐⭐
💠C Programming Specialization💠
👉PROGRAMMING FUNDAMENTALS
0:00:00 Why you should learn to program
0:03:51 Stepping through an algorithm
0:08:11 Testing an algorithm for numerical sequence
0:11:26 A pattern of squares
0:16:12 Testing a pattern of squares
0:18:34 Drawing a rectangle
0:22:42 Closest point
0:27:50 Generalizing closest point
0:32:57 Why you should learn to read code
0:35:56 Declaring and assigning a variable
0:38:15 Example of expressions
0:40:34 Using functions for abstraction
0:44:47 Execution of function calls
0:49:24 printing example
0:52:17 Execution of if else
0:55:35 Execution of Switch case
0:58:41 While loops
1:02:04 Equivalent for and while loops
1:04:21 Execution of nested loops
1:07:57 Execution of continue
1:10:54 Introduction to types
1:11:53 Types and formatted output
1:16:04 Types conversion
1:18:18 Everything is a number
1:21:07 Struct for a rectangle
1:23:20 Uses of typedef
1:26:33 Enumerated types
1:29:57 A Duke Software engineering student on the importance of planning
1:33:32 Importance of writing a specific algorithms
1:35:56 Introduction to sorting
👉WRITING, RUNNING , AND FIXING CODE IN C
1:37:56 Introduction to writing code
1:41:02 Intersection of two rectangles
1:46:06 Translating the intersection algorithm to code
1:51:31 Introduction to the programming environment
1:57:33 Editing files with emacs
2:05:42 More about git
2:10:11 Now we need to compile
2:11:53 Hello world
2:15:09 Planning is prime
2:18:58 Generalizing is prime
2:24:10 Translating is prime to code
2:26:35 Comparing output with diff
2:30:28 Build tool make
2:33:44 Compiling with a makefile
2:36:38 Testing means finding bugs
2:38:57 Test driven development
2:40:45 Code review
2:43:24 Finding problems with valgrind
2:48:30 Gathering information with gdb
2:55:18 Advice from a Duke software engineering student don,t give up
2:57:22 Introduction to the poker project
3:03:45 Poker project roadmap
👉POINTER, ARRAYS, AND RECURSION
3:05:28 Introduction to pointers arrays and recursion
3:07:17 Naive Swap
3:09:44 Pointers
3:12:20 Corrected swap
3:14:58 swap with hardware
3:21:09 Array access with pointer arithmetic
3:23:48 Array access with pointer indexing
3:25:37 Index of largest element
3:32:15 Closest point step through
3:35:39 Dangling pointers
3:39:19 Compare two strings
3:46:46 Copy a string
3:51:33 Incompatible representations
3:56:38 Buffer string attacks instructions
4:00:54 Executing recursive factorial by hand
4:03:00 Writing factorial recursively
4:09:26 Translating recursive factorial to code
4:10:46 Writing fibonacci recursively
4:17:42 Translate recursive fabonacci to code
4:22:03 Duplication of computation in fibonacci
4:23:29 Execution of the tail recursive implementation of factorial
4:28:17 Execution of mutually recursive is odd and is even
4:29:43 advice from a Duke software engineering alum solve real world problems
👉INTERACTING WITH THE SYSTEM AND MANAGING MEMORY
4:34:52 why we need interactivity and to manage memory
4:36:29 Reading a file with f getc
4:40:55 Reading a file with f gets
4:45:57 Writing to a file
4:48:17 Closing a file
4:50:54 Simple call to malloc
4:53:08 Mechanics of free
4:56:06 Code with a memory leak
4:58:46 Three common problems when using free
5:00:45 Call to realloc
5:05:06 Reading a file with getline
5:11:04 Combining getline and realloc
5:16:06 Roster planning
5:21:46 Poker project Final part
___________________________________________________________
⭐ Important Links and Notes ⭐
⌨️ This course is created in collaboration with Duke University
✨✨PLEASE IGNORE THESE TAGS✨✨
#cprogrammingforbeginners #cprogrammingtutorials #cprogramminglanguagebeginners #cprogramminglanguagevideos
c programming tutorial,
c programming full course,
c programming language,
c programming projects,
c programming for beginners,
c programming tutorial for beginners,
c programming pointers,
structure of a c programming,
basic structure of a c programming,
c programming basics,
c programming basics for beginners in english,
c programming btech,
c programming course,
c programming crash course,
c programming for beginners in english,
c programming full course in english
Successful completion of this Specialization will be considered by admissions as a demonstration of your skill and enhance your master’s application to Duke’s Pratt School of Engineering.
⭐⭐⭐⭐🕑TIME STAMP📋⭐⭐⭐⭐⭐
💠C Programming Specialization💠
👉PROGRAMMING FUNDAMENTALS
0:00:00 Why you should learn to program
0:03:51 Stepping through an algorithm
0:08:11 Testing an algorithm for numerical sequence
0:11:26 A pattern of squares
0:16:12 Testing a pattern of squares
0:18:34 Drawing a rectangle
0:22:42 Closest point
0:27:50 Generalizing closest point
0:32:57 Why you should learn to read code
0:35:56 Declaring and assigning a variable
0:38:15 Example of expressions
0:40:34 Using functions for abstraction
0:44:47 Execution of function calls
0:49:24 printing example
0:52:17 Execution of if else
0:55:35 Execution of Switch case
0:58:41 While loops
1:02:04 Equivalent for and while loops
1:04:21 Execution of nested loops
1:07:57 Execution of continue
1:10:54 Introduction to types
1:11:53 Types and formatted output
1:16:04 Types conversion
1:18:18 Everything is a number
1:21:07 Struct for a rectangle
1:23:20 Uses of typedef
1:26:33 Enumerated types
1:29:57 A Duke Software engineering student on the importance of planning
1:33:32 Importance of writing a specific algorithms
1:35:56 Introduction to sorting
👉WRITING, RUNNING , AND FIXING CODE IN C
1:37:56 Introduction to writing code
1:41:02 Intersection of two rectangles
1:46:06 Translating the intersection algorithm to code
1:51:31 Introduction to the programming environment
1:57:33 Editing files with emacs
2:05:42 More about git
2:10:11 Now we need to compile
2:11:53 Hello world
2:15:09 Planning is prime
2:18:58 Generalizing is prime
2:24:10 Translating is prime to code
2:26:35 Comparing output with diff
2:30:28 Build tool make
2:33:44 Compiling with a makefile
2:36:38 Testing means finding bugs
2:38:57 Test driven development
2:40:45 Code review
2:43:24 Finding problems with valgrind
2:48:30 Gathering information with gdb
2:55:18 Advice from a Duke software engineering student don,t give up
2:57:22 Introduction to the poker project
3:03:45 Poker project roadmap
👉POINTER, ARRAYS, AND RECURSION
3:05:28 Introduction to pointers arrays and recursion
3:07:17 Naive Swap
3:09:44 Pointers
3:12:20 Corrected swap
3:14:58 swap with hardware
3:21:09 Array access with pointer arithmetic
3:23:48 Array access with pointer indexing
3:25:37 Index of largest element
3:32:15 Closest point step through
3:35:39 Dangling pointers
3:39:19 Compare two strings
3:46:46 Copy a string
3:51:33 Incompatible representations
3:56:38 Buffer string attacks instructions
4:00:54 Executing recursive factorial by hand
4:03:00 Writing factorial recursively
4:09:26 Translating recursive factorial to code
4:10:46 Writing fibonacci recursively
4:17:42 Translate recursive fabonacci to code
4:22:03 Duplication of computation in fibonacci
4:23:29 Execution of the tail recursive implementation of factorial
4:28:17 Execution of mutually recursive is odd and is even
4:29:43 advice from a Duke software engineering alum solve real world problems
👉INTERACTING WITH THE SYSTEM AND MANAGING MEMORY
4:34:52 why we need interactivity and to manage memory
4:36:29 Reading a file with f getc
4:40:55 Reading a file with f gets
4:45:57 Writing to a file
4:48:17 Closing a file
4:50:54 Simple call to malloc
4:53:08 Mechanics of free
4:56:06 Code with a memory leak
4:58:46 Three common problems when using free
5:00:45 Call to realloc
5:05:06 Reading a file with getline
5:11:04 Combining getline and realloc
5:16:06 Roster planning
5:21:46 Poker project Final part
___________________________________________________________
⭐ Important Links and Notes ⭐
⌨️ This course is created in collaboration with Duke University
✨✨PLEASE IGNORE THESE TAGS✨✨
#cprogrammingforbeginners #cprogrammingtutorials #cprogramminglanguagebeginners #cprogramminglanguagevideos
c programming tutorial,
c programming full course,
c programming language,
c programming projects,
c programming for beginners,
c programming tutorial for beginners,
c programming pointers,
structure of a c programming,
basic structure of a c programming,
c programming basics,
c programming basics for beginners in english,
c programming btech,
c programming course,
c programming crash course,
c programming for beginners in english,
c programming full course in english
Комментарии