filmov
tv
How to Count Array Element Occurrences in JavaScript Efficiently
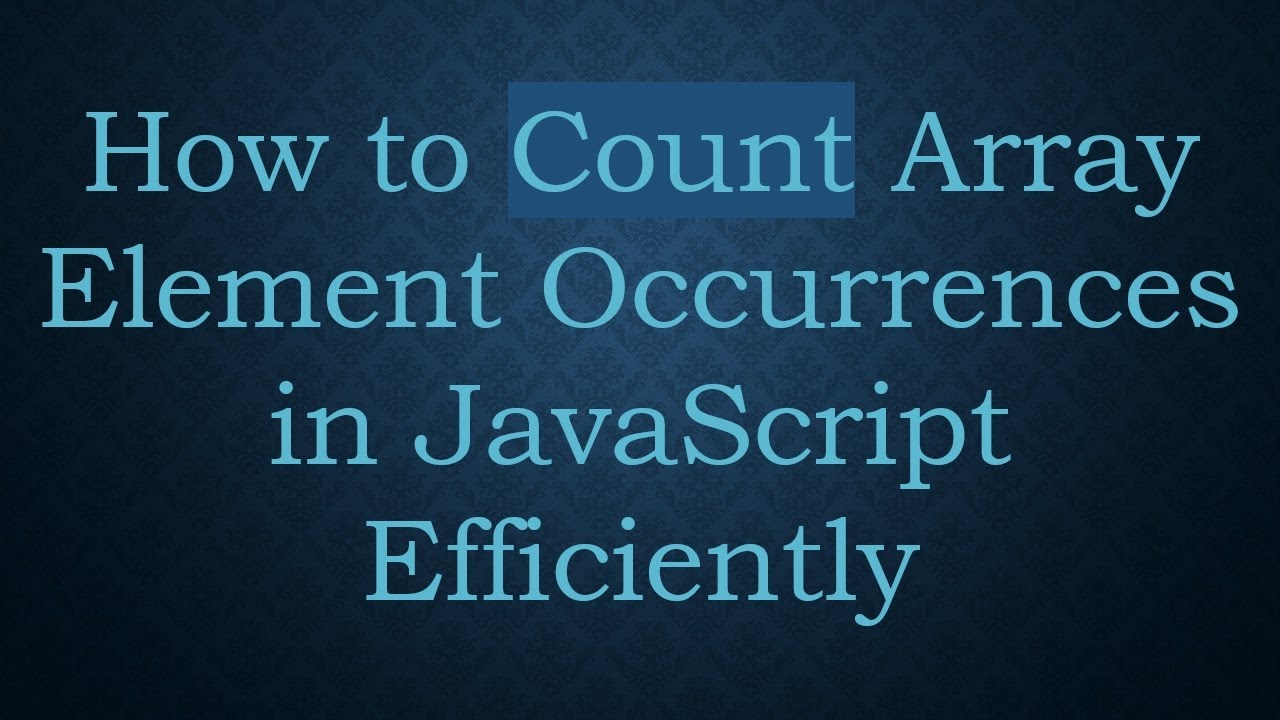
Показать описание
Learn how to count the occurrences of array elements in JavaScript, highlighting elements that appear more than once, all with a time complexity of O(n).
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Counting the occurrences of array elements and print only the element value is greater than 1 in javascript
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Counting Array Element Occurrences in JavaScript
In the world of data manipulation and programming, one common task is counting the occurrences of elements in an array. This is especially important when you want to analyze datasets or perform operations based on frequency. In this guide, we’re going to tackle a problem that many face: How do we count the occurrences of array elements and print only those whose count is greater than one while keeping our solution efficient?
The Problem
Imagine you have an array of numbers, and you want to find out which numbers occur more than once, as well as how many times they appear. For example, given the array:
[[See Video to Reveal this Text or Code Snippet]]
The expected output would be:
[[See Video to Reveal this Text or Code Snippet]]
Where 1 appears 3 times and 3 appears 2 times. The challenge is to achieve this with a time complexity of O(n), meaning our algorithm should run in linear time regardless of the size of the input array.
The Solution
To solve this problem efficiently, we can utilize a JavaScript object to store the count of each element as we iterate through the array. Below, we break down the solution into straightforward steps.
Step 1: Initialize an Object for Counting
We'll create an empty object that will serve as a reference to store the counts of each element.
Step 2: Count Occurrences
As we loop through each element of the array, we will check if it already exists in our count object. If it does, we increment its value; if not, we initialize it to 1.
Step 3: Filter and Print Results
Once we have our counts for each unique element, we will loop through our count object to print only those elements whose counts are greater than 1.
The Code
Here's the complete code that implements the above logic:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Time Complexity
The time complexity of the provided solution is O(n):
Counting Phase: The first loop iterates through the input array once, performing constant-time operations (checking and updating object properties) leading to O(n).
Printing Phase: The second loop goes through the counted elements in our object (in the worst case, as many as unique elements), which also runs in O(n).
When you sum these operations, it gives you O(n + n), which simplifies to O(n). This ensures our solution is linear, making it efficient for large datasets.
Conclusion
Counting the occurrences of elements in an array is a common yet essential task in programming, and with JavaScript, we can achieve this efficiently with a time complexity of O(n). By leveraging objects for counting, we not only simplify our code but also enhance performance.
Now you have the skills to tackle similar problems and optimize your code to handle arrays effectively. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Counting the occurrences of array elements and print only the element value is greater than 1 in javascript
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Counting Array Element Occurrences in JavaScript
In the world of data manipulation and programming, one common task is counting the occurrences of elements in an array. This is especially important when you want to analyze datasets or perform operations based on frequency. In this guide, we’re going to tackle a problem that many face: How do we count the occurrences of array elements and print only those whose count is greater than one while keeping our solution efficient?
The Problem
Imagine you have an array of numbers, and you want to find out which numbers occur more than once, as well as how many times they appear. For example, given the array:
[[See Video to Reveal this Text or Code Snippet]]
The expected output would be:
[[See Video to Reveal this Text or Code Snippet]]
Where 1 appears 3 times and 3 appears 2 times. The challenge is to achieve this with a time complexity of O(n), meaning our algorithm should run in linear time regardless of the size of the input array.
The Solution
To solve this problem efficiently, we can utilize a JavaScript object to store the count of each element as we iterate through the array. Below, we break down the solution into straightforward steps.
Step 1: Initialize an Object for Counting
We'll create an empty object that will serve as a reference to store the counts of each element.
Step 2: Count Occurrences
As we loop through each element of the array, we will check if it already exists in our count object. If it does, we increment its value; if not, we initialize it to 1.
Step 3: Filter and Print Results
Once we have our counts for each unique element, we will loop through our count object to print only those elements whose counts are greater than 1.
The Code
Here's the complete code that implements the above logic:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Time Complexity
The time complexity of the provided solution is O(n):
Counting Phase: The first loop iterates through the input array once, performing constant-time operations (checking and updating object properties) leading to O(n).
Printing Phase: The second loop goes through the counted elements in our object (in the worst case, as many as unique elements), which also runs in O(n).
When you sum these operations, it gives you O(n + n), which simplifies to O(n). This ensures our solution is linear, making it efficient for large datasets.
Conclusion
Counting the occurrences of elements in an array is a common yet essential task in programming, and with JavaScript, we can achieve this efficiently with a time complexity of O(n). By leveraging objects for counting, we not only simplify our code but also enhance performance.
Now you have the skills to tackle similar problems and optimize your code to handle arrays effectively. Happy coding!