filmov
tv
LeetCode 30 day Challenge | Day 7 | Counting Elements | LeetCode #1426
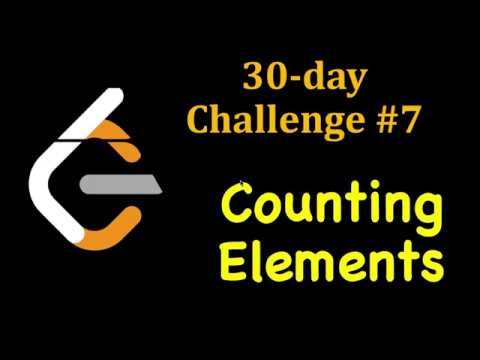
Показать описание
**** Best Books For Data Structures & Algorithms for Interviews:**********
*****************************************************************************
LeetCode 30 day Challenge | Problem 7 | Counting Elements | 7 April
Facebook Coding Interview question,
google coding interview question,
leetcode,
counting elements,
#Amazon #Facebook #CodingInterview #LeetCode #CountingElements #30DayChallenge #Google
*****************************************************************************
LeetCode 30 day Challenge | Problem 7 | Counting Elements | 7 April
Facebook Coding Interview question,
google coding interview question,
leetcode,
counting elements,
#Amazon #Facebook #CodingInterview #LeetCode #CountingElements #30DayChallenge #Google
3 Months of Learning Leetcode
I solved 541 Leetcode problems. But you need only 150.
30-Day LeetCoding Challenge Speedrun (1:00:38.53)
Daily LeetCode. A 30-day LeetCoding Challenge. Day 1: Single Number
LeetCode 30 Day Challenge Day 1: Single Number
LeetCode April Challenge Day 1 - Easy Start
LeetCode 30 Day Challenge: Single Number
Leetcode coding - Day 1 (30 day challenge)
Create Hello World Function (Closure - Day1) - Leetcode 2667 - JavaScript 30-Day Challenge
First Unique Number (LeetCode 30 Day Challenge) | Programming Tutorials
How to use Leetcode effectively | 30 days challenge
Leetcode coding - Day 2 (30 day challenge)
Hello World in Javascript - 30-Day Leetcode Challenge #1
LeetCode 30-Days JavaScript Challenge: Solving 'To Be Or Not To Be' (2704) #leetcode #java...
Counter (Closure - Day2) - Leetcode 2620 - JavaScript 30-Day Challenge
LeetCode 30 Days of JavaScript - Day 1: Hello World
Leetcode coding - Day 3 (30 day challenge)
Leetcode coding - Day 6 (30 day challenge)
769. Max Chunks To Make Sorted | leetcode daily challenge | shashcode30
2415. Reverse Odd Levels of Binary Tree | leetcode daily challenge | shashcode30
Leftmost Column with at Least a One | LeetCode 30 day Challenge | Day 21 | (C++, Java, Python)
Curry - Leetcode 2632 - JavaScript 30-Day Challenge
30 Days Javascript LeetCode Challenge | Day-2 | Vishwa Mohan
Leetcode coding - Day 7 (30 day challenge)
Комментарии