filmov
tv
How to Convert a Boolean to an Int in Java
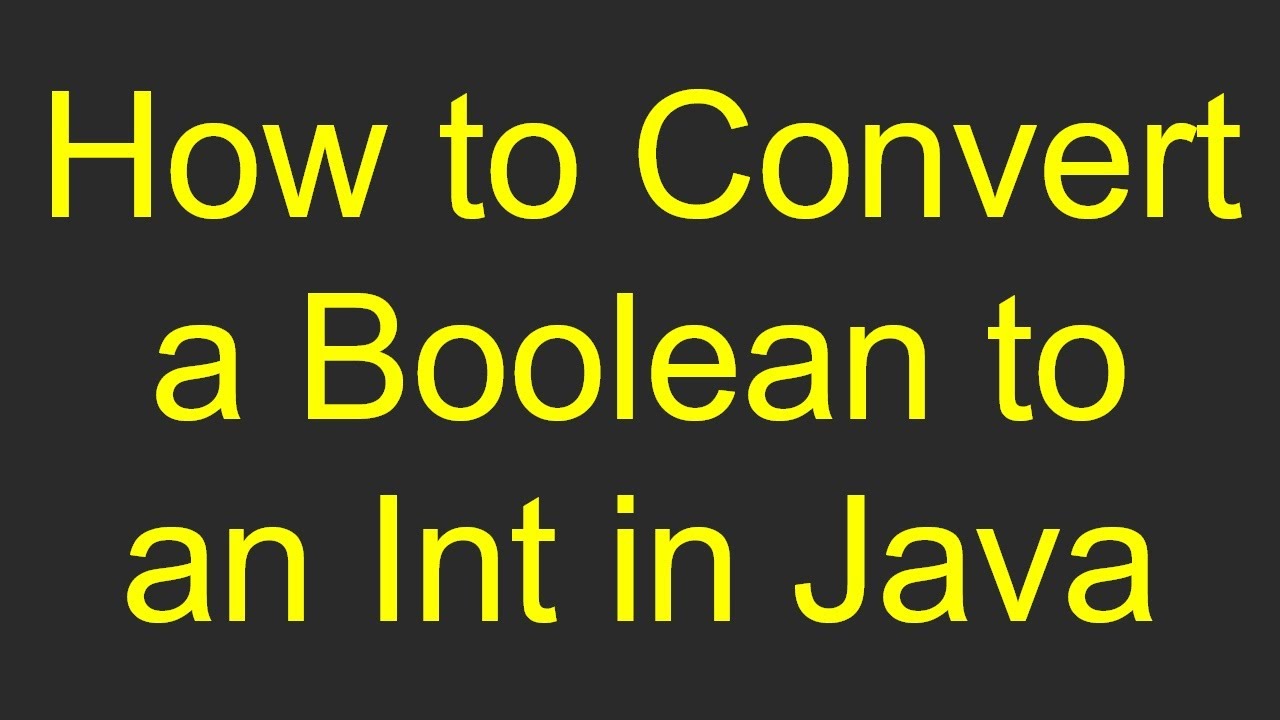
Показать описание
Learn how to convert a boolean value to an integer in Java. Explore simple techniques to achieve this conversion effectively and understand the implications for your Java programs.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
How to Convert a Boolean to an Int in Java
In Java, you may occasionally find the need to convert a boolean value (true or false) to an integer (1 or 0). This need can arise in various scenarios, such as data processing, interfacing with databases, or systems that require numerical representation of truth values. Java, being a statically typed language, doesn't offer direct casting for boolean to int as some other languages might. However, there are several straightforward ways to achieve this conversion.
Method 1: Using an If-Else Statement
The simplest way to convert a boolean to an integer is by using an if-else statement. This method is clear and easy to understand:
[[See Video to Reveal this Text or Code Snippet]]
This method explicitly checks the boolean value and assigns the corresponding integer manually. It’s particularly useful when the logic involves more than just a simple conversion, allowing additional operations to be easily integrated within the if and else branches.
Method 2: Ternary Operator
A more concise approach uses the ternary operator, which is a shorthand for the if-else statement. This method is preferred for its brevity and inline capability:
[[See Video to Reveal this Text or Code Snippet]]
The ternary operator (? :) evaluates the boolean expression, returning the value immediately after the question mark if true, and the value after the colon if false. This one-liner is efficient and clean, ideal for quick conversions within more complex expressions.
Method 3: Using Bitwise Operators
While not common, it is possible to use bitwise operators to achieve the same. This method leverages the fact that in bitwise operations, true and false are often treated as 1 and 0, respectively:
[[See Video to Reveal this Text or Code Snippet]]
This approach, while interesting, essentially simplifies to the ternary method in terms of functionality and readability.
Conclusion
Converting a boolean to an integer in Java can't be done via direct casting but can be efficiently achieved using simple conditional statements or the ternary operator. Each method has its place, depending on the context in which you're working. Whether you need clarity and explicit control or prefer concise and elegant code, Java provides the flexibility to suit your coding style and requirements.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
How to Convert a Boolean to an Int in Java
In Java, you may occasionally find the need to convert a boolean value (true or false) to an integer (1 or 0). This need can arise in various scenarios, such as data processing, interfacing with databases, or systems that require numerical representation of truth values. Java, being a statically typed language, doesn't offer direct casting for boolean to int as some other languages might. However, there are several straightforward ways to achieve this conversion.
Method 1: Using an If-Else Statement
The simplest way to convert a boolean to an integer is by using an if-else statement. This method is clear and easy to understand:
[[See Video to Reveal this Text or Code Snippet]]
This method explicitly checks the boolean value and assigns the corresponding integer manually. It’s particularly useful when the logic involves more than just a simple conversion, allowing additional operations to be easily integrated within the if and else branches.
Method 2: Ternary Operator
A more concise approach uses the ternary operator, which is a shorthand for the if-else statement. This method is preferred for its brevity and inline capability:
[[See Video to Reveal this Text or Code Snippet]]
The ternary operator (? :) evaluates the boolean expression, returning the value immediately after the question mark if true, and the value after the colon if false. This one-liner is efficient and clean, ideal for quick conversions within more complex expressions.
Method 3: Using Bitwise Operators
While not common, it is possible to use bitwise operators to achieve the same. This method leverages the fact that in bitwise operations, true and false are often treated as 1 and 0, respectively:
[[See Video to Reveal this Text or Code Snippet]]
This approach, while interesting, essentially simplifies to the ternary method in terms of functionality and readability.
Conclusion
Converting a boolean to an integer in Java can't be done via direct casting but can be efficiently achieved using simple conditional statements or the ternary operator. Each method has its place, depending on the context in which you're working. Whether you need clarity and explicit control or prefer concise and elegant code, Java provides the flexibility to suit your coding style and requirements.