filmov
tv
'Unlock the Power of Python: Build Your Own Calculator Today!' 🔥
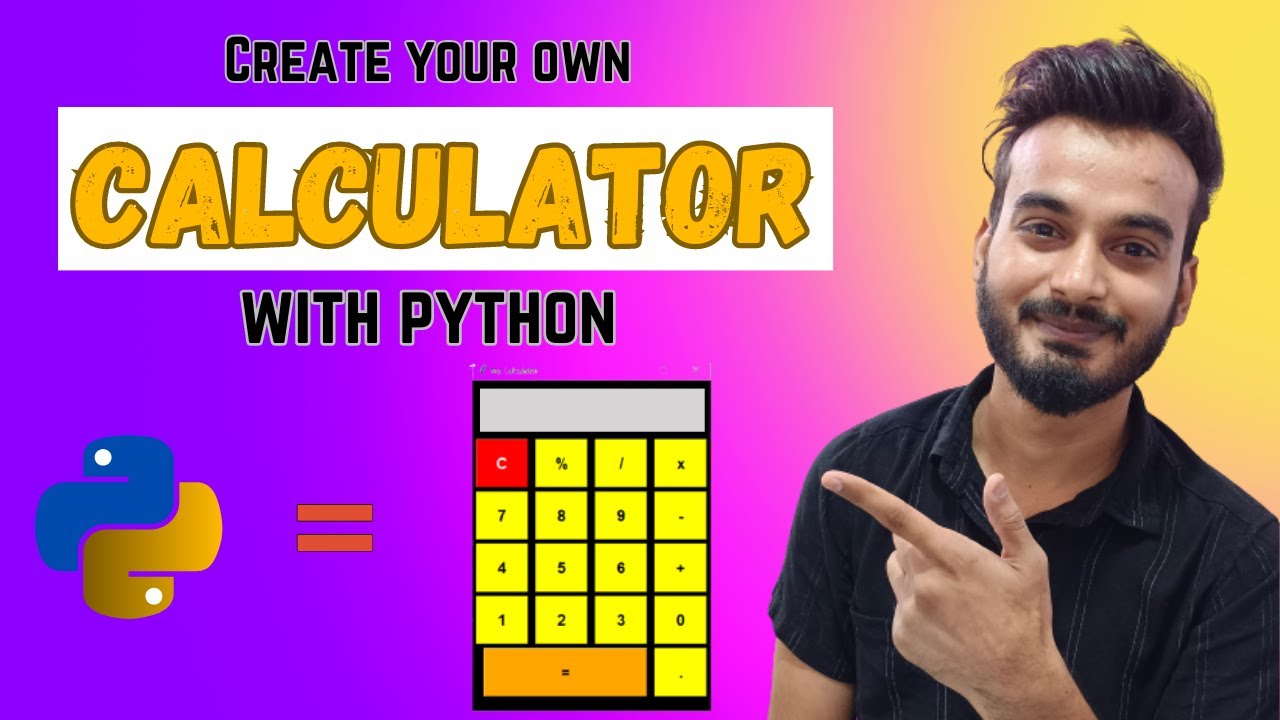
Показать описание
"Unlock the Power of Python: Build Your Own Calculator Today!" 🔥 WITH SOURCE CODE 😊
In this tutorial, i will teach you how to build a simple calculator using python with a very simple python coding along with step by step explanation.
For this project we are using only 1 Library - Tkinter Library( Inbuild Python Library), which is very easy to use. Hope you Enjoy this video.
►Don't forget to subscribe to my channel. By the way, it's completely FREE!
🆓🆓🆓🆓🆓🆓🆓🆓🆓🆓🆓🆓
☑ Watched the video!
☐ Liked?
☐ Subscribed?
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~Source Code ~~~~~~~~~~~~~~~~~~~~
from tkinter import *
win = Tk()
#Buttons Function
equation = ''
def show(value):
global equation
equation += value
# Clear Button Function
def clear():
global equation
equation = ''
# Calculate Button Function
def calculate():
global equation
result = ''
if equation != '':
try:
result = eval(equation)
except:
result = ' Error'
equation = ''
#Result Label
result_lbl = Label(win,text='',font='impack 20 bold',bg='black',fg='white',bd=15)
#Buttons
btn_c = Button(win,text='C',bg='red',fg='white',width=5,height=2,font='impack 17 bold',cursor='hand2',command=lambda: clear())
btn_p = Button(win,text='%',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('%'))
btn_d = Button(win,text='/',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('/'))
btn_m = Button(win,text='x',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('*'))
btn_7 = Button(win,text='7',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('7'))
btn_8 = Button(win,text='8',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('8'))
btn_9 = Button(win,text='9',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('9'))
btn_mn = Button(win,text='-',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('-'))
btn_4 = Button(win,text='4',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('4'))
btn_5 = Button(win,text='5',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('5'))
btn_6 = Button(win,text='6',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('6'))
btn_pl = Button(win,text='+',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('+'))
btn_1 = Button(win,text='1',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('1'))
btn_2 = Button(win,text='2',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('2'))
btn_3 = Button(win,text='3',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('3'))
btn_0 = Button(win,text='0',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('0'))
btn_dot = Button(win,text='.',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('.'))
btn_eq = Button(win,text="=",bg='orange',width=17,height=2,font='impack 17 bold',cursor='hand2',command= lambda: calculate())
mainloop()
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Related Questions People Also Asks :-
calculator with python
calculator with python tkinter
Can you make a calculator with Python?
Which is the best GUI calculator for Python?
How to do calculation in Python?
क्या आप पाइथन से कैलकुलेटर बना सकते हैं?
#python
#pythonforbeginners
#calculator
#pythontutorial
#pythonsimpleprojects
#pythonprogramming
#pythonprojects
#tkinter
#tkinterprojects
In this tutorial, i will teach you how to build a simple calculator using python with a very simple python coding along with step by step explanation.
For this project we are using only 1 Library - Tkinter Library( Inbuild Python Library), which is very easy to use. Hope you Enjoy this video.
►Don't forget to subscribe to my channel. By the way, it's completely FREE!
🆓🆓🆓🆓🆓🆓🆓🆓🆓🆓🆓🆓
☑ Watched the video!
☐ Liked?
☐ Subscribed?
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~Source Code ~~~~~~~~~~~~~~~~~~~~
from tkinter import *
win = Tk()
#Buttons Function
equation = ''
def show(value):
global equation
equation += value
# Clear Button Function
def clear():
global equation
equation = ''
# Calculate Button Function
def calculate():
global equation
result = ''
if equation != '':
try:
result = eval(equation)
except:
result = ' Error'
equation = ''
#Result Label
result_lbl = Label(win,text='',font='impack 20 bold',bg='black',fg='white',bd=15)
#Buttons
btn_c = Button(win,text='C',bg='red',fg='white',width=5,height=2,font='impack 17 bold',cursor='hand2',command=lambda: clear())
btn_p = Button(win,text='%',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('%'))
btn_d = Button(win,text='/',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('/'))
btn_m = Button(win,text='x',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('*'))
btn_7 = Button(win,text='7',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('7'))
btn_8 = Button(win,text='8',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('8'))
btn_9 = Button(win,text='9',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('9'))
btn_mn = Button(win,text='-',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('-'))
btn_4 = Button(win,text='4',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('4'))
btn_5 = Button(win,text='5',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('5'))
btn_6 = Button(win,text='6',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('6'))
btn_pl = Button(win,text='+',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('+'))
btn_1 = Button(win,text='1',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('1'))
btn_2 = Button(win,text='2',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('2'))
btn_3 = Button(win,text='3',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('3'))
btn_0 = Button(win,text='0',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('0'))
btn_dot = Button(win,text='.',bg='yellow',width=5,height=2,font='impack 17 bold',cursor='hand2',command= lambda: show('.'))
btn_eq = Button(win,text="=",bg='orange',width=17,height=2,font='impack 17 bold',cursor='hand2',command= lambda: calculate())
mainloop()
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Related Questions People Also Asks :-
calculator with python
calculator with python tkinter
Can you make a calculator with Python?
Which is the best GUI calculator for Python?
How to do calculation in Python?
क्या आप पाइथन से कैलकुलेटर बना सकते हैं?
#python
#pythonforbeginners
#calculator
#pythontutorial
#pythonsimpleprojects
#pythonprogramming
#pythonprojects
#tkinter
#tkinterprojects