filmov
tv
SDET Automation Testing Interview Questions & Answers
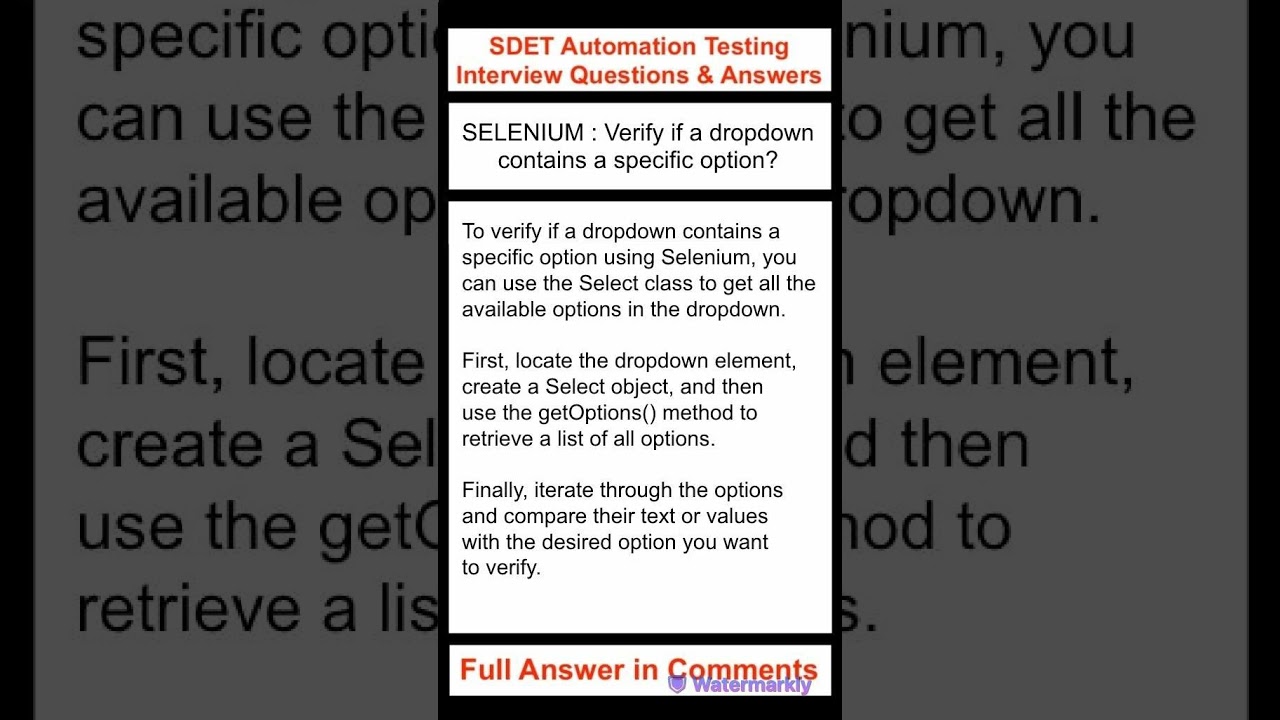
Показать описание
SDET Automation Testing Interview Questions & Answers
We will be covering a wide range of topics including QA manual testing, automation testing, Selenium, Java, Jenkins, Cucumber, Maven, and various testing frameworks.
To verify if a dropdown contains a specific option using Selenium, you can use the `Select` class provided by the Selenium WebDriver. The `Select` class has methods that allow you to interact with dropdown elements and retrieve information about the available options. Here's an example of how to verify if a dropdown contains a specific option:
public class DropdownVerificationExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
// Locate the dropdown element
// Create a Select object with the dropdown element
Select dropdown = new Select(dropdownElement);
// Verify if the dropdown contains a specific option by visible text
if (isOptionPresent) {
} else {
}
}
}
In the above example, the `Select` class is used to interact with the dropdown element. The `getOptions()` method returns a list of all available options in the dropdown. You can then use Java 8+ Stream API to iterate through the options and check if the desired option is present.
In the example, we use the `anyMatch()` method to iterate through the options and check if any option's visible text matches the desired option text ("Option 1" in this case). If there is a match, `isOptionPresent` will be set to `true`; otherwise, it will be `false`.
Make sure to have the appropriate WebDriver initialized and the necessary dependencies imported in your project.
We will be covering a wide range of topics including QA manual testing, automation testing, Selenium, Java, Jenkins, Cucumber, Maven, and various testing frameworks.
To verify if a dropdown contains a specific option using Selenium, you can use the `Select` class provided by the Selenium WebDriver. The `Select` class has methods that allow you to interact with dropdown elements and retrieve information about the available options. Here's an example of how to verify if a dropdown contains a specific option:
public class DropdownVerificationExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
// Locate the dropdown element
// Create a Select object with the dropdown element
Select dropdown = new Select(dropdownElement);
// Verify if the dropdown contains a specific option by visible text
if (isOptionPresent) {
} else {
}
}
}
In the above example, the `Select` class is used to interact with the dropdown element. The `getOptions()` method returns a list of all available options in the dropdown. You can then use Java 8+ Stream API to iterate through the options and check if the desired option is present.
In the example, we use the `anyMatch()` method to iterate through the options and check if any option's visible text matches the desired option text ("Option 1" in this case). If there is a match, `isOptionPresent` will be set to `true`; otherwise, it will be `false`.
Make sure to have the appropriate WebDriver initialized and the necessary dependencies imported in your project.
Комментарии