filmov
tv
How to Resolve ValueError in Python for Element-wise Multiplication of Matrices?
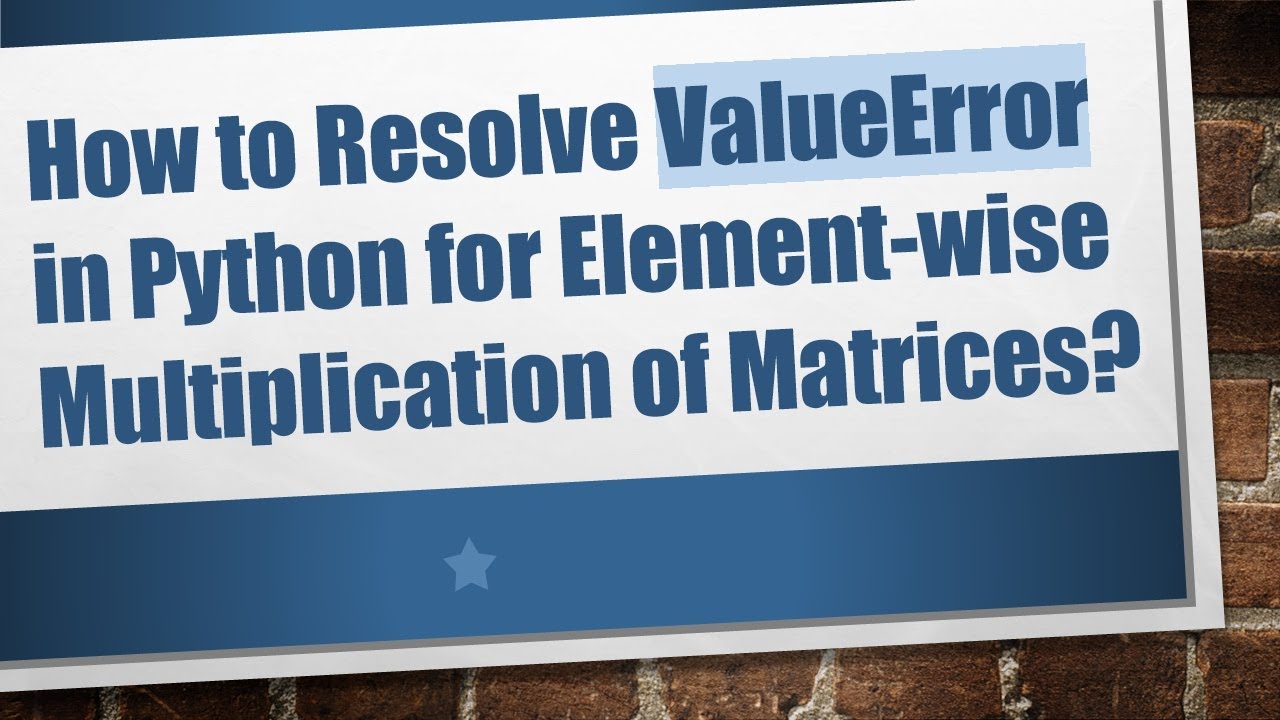
Показать описание
Learn how to effectively resolve `ValueError` in Python when performing element-wise multiplication of matrices using numpy.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
How to Resolve ValueError in Python for Element-wise Multiplication of Matrices?
Encountering a ValueError when performing element-wise multiplication in Python can be particularly frustrating, especially if you're working with matrices. This error essentially indicates a problem with the shapes of the arrays or matrices being operated on. Let's delve into how you can resolve this issue using the numpy library, which provides a rich set of mathematical functions and is highly optimized for matrix operations.
Understanding the ValueError
When you attempt to perform element-wise multiplication on matrices with incompatible shapes, Python throws a ValueError. The numpy library requires that the matrices involved in element-wise operations have the same shape.
Example of ValueError
Here’s a quick example to illustrate the issue:
[[See Video to Reveal this Text or Code Snippet]]
In this case, Matrix A has a shape of (2, 3), and Matrix B has a shape of (3, 2). These shapes are incompatible for element-wise multiplication, leading to a ValueError.
Resolving the ValueError
Ensure the Matrices Have the Same Shape
To perform element-wise multiplication, you must ensure the matrices have the same shape. You can achieve this by reshaping one or both matrices to align their shapes.
Example Solution
Here’s an example using the correct shapes:
[[See Video to Reveal this Text or Code Snippet]]
In this corrected example, both Matrix A and Matrix B have the same shape (2, 3), thus avoiding the ValueError and successfully performing element-wise multiplication.
Broadcasting with Numpy
numpy also provides broadcasting capabilities to handle certain shape mismatches. Broadcasting involves expanding smaller arrays to match the shape of larger ones for operations. However, it comes with its own rules and limitations, and both arrays must still be compatible in certain dimensions.
[[See Video to Reveal this Text or Code Snippet]]
In this example, Matrix A is of shape (2, 1), and Matrix B is of shape (1, 3). Broadcasting expands these matrices to a common shape of (2, 3) for the element-wise multiplication.
Conclusion
Resolving a ValueError in Python due to element-wise multiplication of matrices often involves ensuring that the matrices have compatible shapes. Using the numpy library, you can either reshape the matrices or leverage broadcasting to perform the desired operations. Understanding these fundamental concepts will help you efficiently handle mathematical operations involving matrices in Python.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
How to Resolve ValueError in Python for Element-wise Multiplication of Matrices?
Encountering a ValueError when performing element-wise multiplication in Python can be particularly frustrating, especially if you're working with matrices. This error essentially indicates a problem with the shapes of the arrays or matrices being operated on. Let's delve into how you can resolve this issue using the numpy library, which provides a rich set of mathematical functions and is highly optimized for matrix operations.
Understanding the ValueError
When you attempt to perform element-wise multiplication on matrices with incompatible shapes, Python throws a ValueError. The numpy library requires that the matrices involved in element-wise operations have the same shape.
Example of ValueError
Here’s a quick example to illustrate the issue:
[[See Video to Reveal this Text or Code Snippet]]
In this case, Matrix A has a shape of (2, 3), and Matrix B has a shape of (3, 2). These shapes are incompatible for element-wise multiplication, leading to a ValueError.
Resolving the ValueError
Ensure the Matrices Have the Same Shape
To perform element-wise multiplication, you must ensure the matrices have the same shape. You can achieve this by reshaping one or both matrices to align their shapes.
Example Solution
Here’s an example using the correct shapes:
[[See Video to Reveal this Text or Code Snippet]]
In this corrected example, both Matrix A and Matrix B have the same shape (2, 3), thus avoiding the ValueError and successfully performing element-wise multiplication.
Broadcasting with Numpy
numpy also provides broadcasting capabilities to handle certain shape mismatches. Broadcasting involves expanding smaller arrays to match the shape of larger ones for operations. However, it comes with its own rules and limitations, and both arrays must still be compatible in certain dimensions.
[[See Video to Reveal this Text or Code Snippet]]
In this example, Matrix A is of shape (2, 1), and Matrix B is of shape (1, 3). Broadcasting expands these matrices to a common shape of (2, 3) for the element-wise multiplication.
Conclusion
Resolving a ValueError in Python due to element-wise multiplication of matrices often involves ensuring that the matrices have compatible shapes. Using the numpy library, you can either reshape the matrices or leverage broadcasting to perform the desired operations. Understanding these fundamental concepts will help you efficiently handle mathematical operations involving matrices in Python.