filmov
tv
The openai python api introduction example code
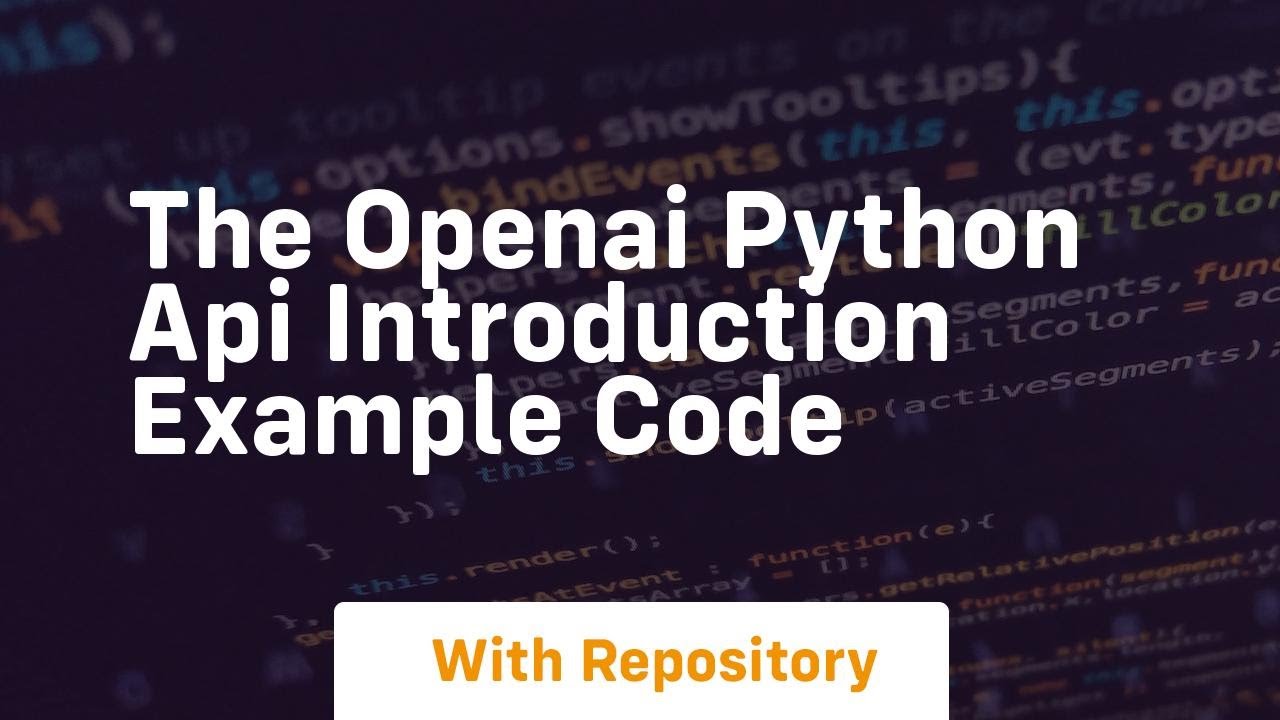
Показать описание
certainly! the openai api allows you to interact with openai's language models, including capabilities for text generation, summarization, translation, and more. in this tutorial, i'll walk you through the basics of using the openai api in python, including how to set it up and make a simple request.
### step 1: setting up your environment
1. **sign up for openai api access**:
2. **install required libraries**:
- you'll need the `openai` python package. you can install it using pip:
### step 2: writing your first code example
here's a simple python script that demonstrates how to use the openai api to generate text.
#### example code
### explanation of the code
1. **import the library**:
- `import openai`: this imports the openai library to your script.
2. **set your api key**:
- replace `'your_api_key_here'` with your actual openai api key. this key is crucial for authenticating your requests.
3. **generate text function**:
- **function definition**: the `generate_text` function takes a `prompt` (the text you want to base the generation on) as an argument.
- `model`: specifies which openai model to use (e.g., `gpt-3.5-turbo`).
- `messages`: contains a list of messages in a conversation format. here, we only send one message from the user.
- `max_tokens`: controls the length of the output. you can adjust this number based on your needs.
- **response handling**: the function extracts the generated text from the api response and returns it.
4. **main block**:
- the script checks if it is being run as the main module and then defines a prompt. it calls the `generate_text` function and prints the result.
### step 3: running the script
- save the script in a file, e.g., `openai_examp ...
#python api server
#python api call
#python api framework
#python api gateway
#python api tutorial
python api server
python api call
python api framework
python api gateway
python api tutorial
python api
python api request
python api development
python api library
python api practice
python code tester
python code compiler
python code
python code runner
python code generator
python coder
python code editor
python code checker
### step 1: setting up your environment
1. **sign up for openai api access**:
2. **install required libraries**:
- you'll need the `openai` python package. you can install it using pip:
### step 2: writing your first code example
here's a simple python script that demonstrates how to use the openai api to generate text.
#### example code
### explanation of the code
1. **import the library**:
- `import openai`: this imports the openai library to your script.
2. **set your api key**:
- replace `'your_api_key_here'` with your actual openai api key. this key is crucial for authenticating your requests.
3. **generate text function**:
- **function definition**: the `generate_text` function takes a `prompt` (the text you want to base the generation on) as an argument.
- `model`: specifies which openai model to use (e.g., `gpt-3.5-turbo`).
- `messages`: contains a list of messages in a conversation format. here, we only send one message from the user.
- `max_tokens`: controls the length of the output. you can adjust this number based on your needs.
- **response handling**: the function extracts the generated text from the api response and returns it.
4. **main block**:
- the script checks if it is being run as the main module and then defines a prompt. it calls the `generate_text` function and prints the result.
### step 3: running the script
- save the script in a file, e.g., `openai_examp ...
#python api server
#python api call
#python api framework
#python api gateway
#python api tutorial
python api server
python api call
python api framework
python api gateway
python api tutorial
python api
python api request
python api development
python api library
python api practice
python code tester
python code compiler
python code
python code runner
python code generator
python coder
python code editor
python code checker