filmov
tv
How to Convert a String to UTF-8 Bytes in Python
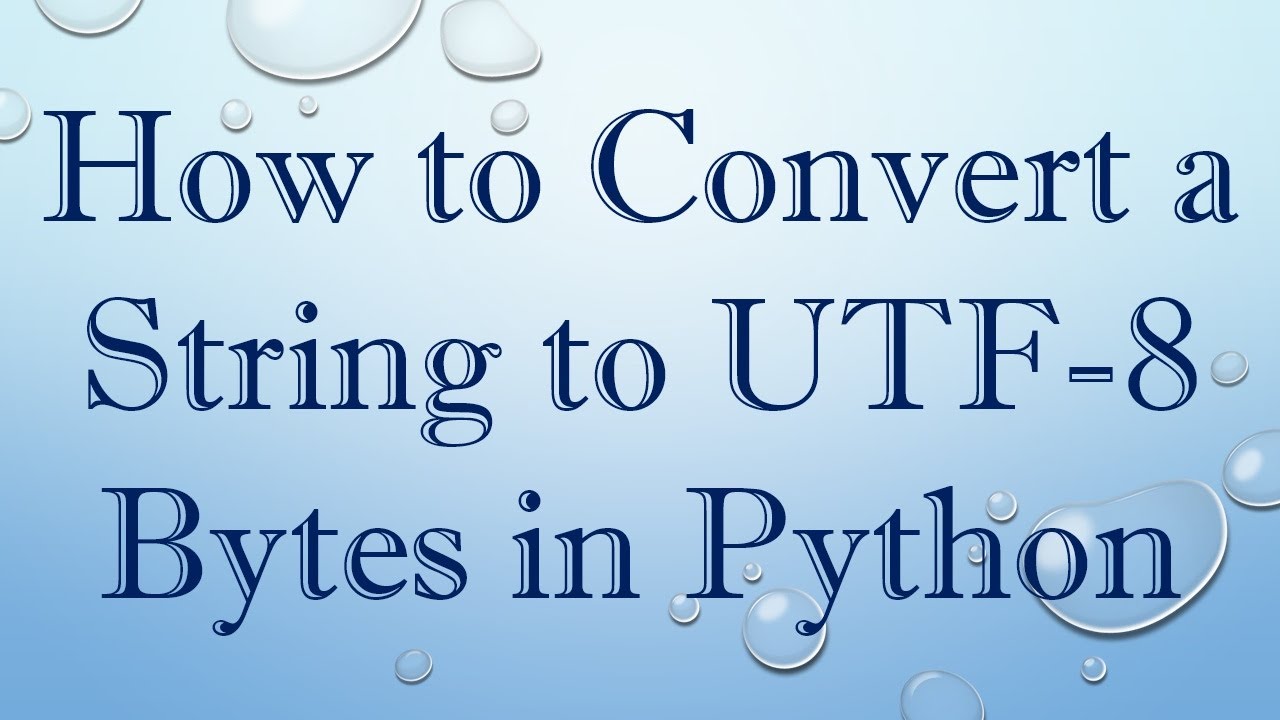
Показать описание
Learn how to convert a string to UTF-8 bytes in Python efficiently using built-in methods and techniques. Discover the importance of encoding and decoding strings to maintain data integrity and compatibility across different systems and applications.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
When working with strings in Python, especially when dealing with encoding and decoding to ensure compatibility across different systems and applications, it's essential to understand how to convert a string to UTF-8 bytes. UTF-8 is a widely used encoding format that represents Unicode characters efficiently and is compatible with most modern systems. Here's how you can do it in Python:
[[See Video to Reveal this Text or Code Snippet]]
In the above code:
We first define a string my_string containing the text "Hello, world!".
We then use the encode() method on the string, specifying the encoding format 'utf-8'. This method converts the string to bytes using the UTF-8 encoding.
The resulting bytes are stored in the variable utf8_bytes.
Finally, we print utf8_bytes to see the UTF-8 representation of the original string.
It's important to note that when working with text in Python, you're often dealing with Unicode strings internally. However, when interacting with external systems or files, it's common to encode the strings into a specific byte representation, such as UTF-8, to ensure proper handling of characters.
Conversely, if you have UTF-8 bytes and want to convert them back to a string, you can use the decode() method:
[[See Video to Reveal this Text or Code Snippet]]
This code will decode the UTF-8 bytes back into a string using the specified encoding ('utf-8').
By understanding how to convert strings to UTF-8 bytes and vice versa, you can ensure proper handling of text data in your Python applications, maintaining compatibility and integrity across different platforms and environments.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
When working with strings in Python, especially when dealing with encoding and decoding to ensure compatibility across different systems and applications, it's essential to understand how to convert a string to UTF-8 bytes. UTF-8 is a widely used encoding format that represents Unicode characters efficiently and is compatible with most modern systems. Here's how you can do it in Python:
[[See Video to Reveal this Text or Code Snippet]]
In the above code:
We first define a string my_string containing the text "Hello, world!".
We then use the encode() method on the string, specifying the encoding format 'utf-8'. This method converts the string to bytes using the UTF-8 encoding.
The resulting bytes are stored in the variable utf8_bytes.
Finally, we print utf8_bytes to see the UTF-8 representation of the original string.
It's important to note that when working with text in Python, you're often dealing with Unicode strings internally. However, when interacting with external systems or files, it's common to encode the strings into a specific byte representation, such as UTF-8, to ensure proper handling of characters.
Conversely, if you have UTF-8 bytes and want to convert them back to a string, you can use the decode() method:
[[See Video to Reveal this Text or Code Snippet]]
This code will decode the UTF-8 bytes back into a string using the specified encoding ('utf-8').
By understanding how to convert strings to UTF-8 bytes and vice versa, you can ensure proper handling of text data in your Python applications, maintaining compatibility and integrity across different platforms and environments.