filmov
tv
#5_Program_in_PythonE#python Group Elements of Same Indices Using in Python #smw_official #smw_media
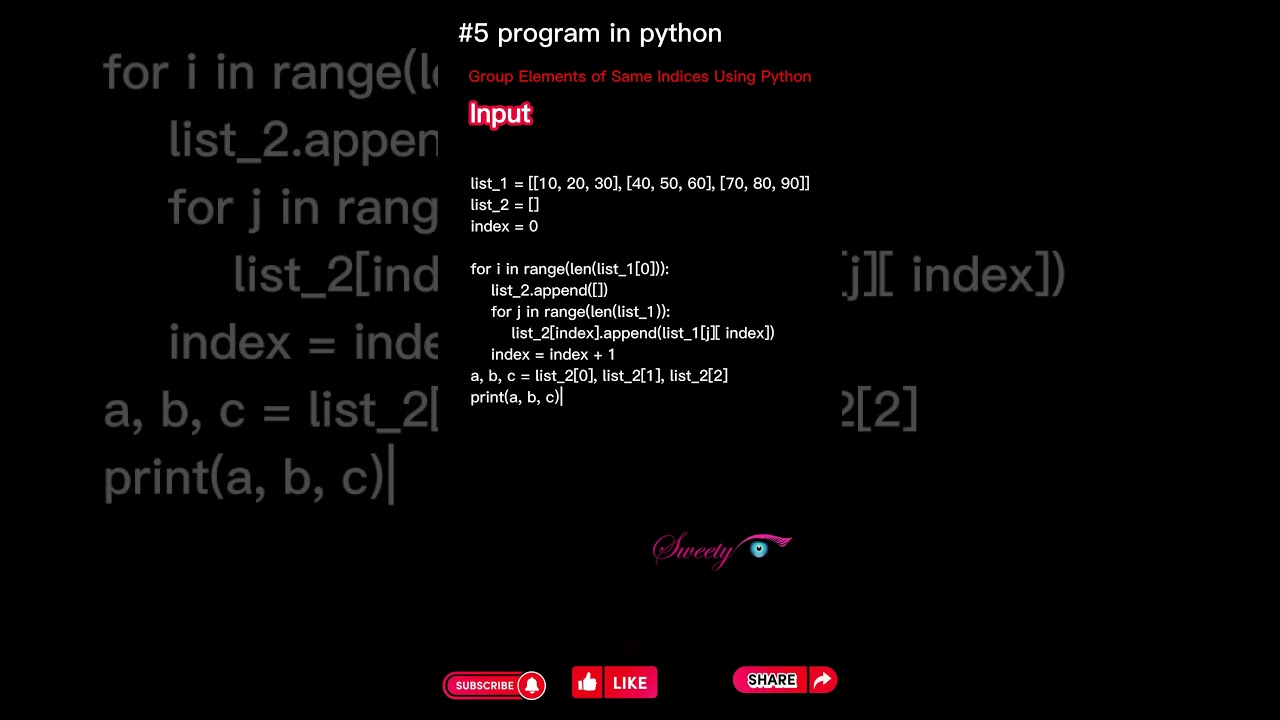
Показать описание
Here's a line-by-line explanation of the code:
full code
```
list_1 = [[10, 20, 30], [40, 50, 60], [70, 80, 90]]
list_2 = []
index = 0
```
This sets up the input list `list_1`, an empty output list `list_2`, and a variable `index` that will be used to keep track of the current column being processed.
```
for i in range(len(list_1[0])):
for j in range(len(list_1)):
list_2[index].append(list_1[j][ index])
index = index + 1
```
This loop iterates over the columns of `list_1`, using the `len(list_1[0])` expression to determine the number of iterations. For each iteration, a new empty list is appended to `list_2`, and then the elements of the current column are appended to this new list using a nested loop. The nested loop iterates over the rows of `list_1`, using the `len(list_1)` expression to determine the number of iterations. For each iteration, the value at the current row and column is appended to the new list in `list_2`. After all the rows have been processed, the `index` variable is incremented to move to the next column.
```
a, b, c = list_2[0], list_2[1], list_2[2]
print(a, b, c)
```
This code extracts the three columns of `list_2` and assigns them to variables `a`, `b`, and `c`. Then, it prints the values of `a`, `b`, and `c` to the console.
Overall, this code is a fairly straightforward implementation of a matrix transpose operation, where the rows and columns of a 2D array are swapped. The resulting transposed array is stored in a new list.
full code
```
list_1 = [[10, 20, 30], [40, 50, 60], [70, 80, 90]]
list_2 = []
index = 0
```
This sets up the input list `list_1`, an empty output list `list_2`, and a variable `index` that will be used to keep track of the current column being processed.
```
for i in range(len(list_1[0])):
for j in range(len(list_1)):
list_2[index].append(list_1[j][ index])
index = index + 1
```
This loop iterates over the columns of `list_1`, using the `len(list_1[0])` expression to determine the number of iterations. For each iteration, a new empty list is appended to `list_2`, and then the elements of the current column are appended to this new list using a nested loop. The nested loop iterates over the rows of `list_1`, using the `len(list_1)` expression to determine the number of iterations. For each iteration, the value at the current row and column is appended to the new list in `list_2`. After all the rows have been processed, the `index` variable is incremented to move to the next column.
```
a, b, c = list_2[0], list_2[1], list_2[2]
print(a, b, c)
```
This code extracts the three columns of `list_2` and assigns them to variables `a`, `b`, and `c`. Then, it prints the values of `a`, `b`, and `c` to the console.
Overall, this code is a fairly straightforward implementation of a matrix transpose operation, where the rows and columns of a 2D array are swapped. The resulting transposed array is stored in a new list.