filmov
tv
Python Append to List: Practical Techniques Explained
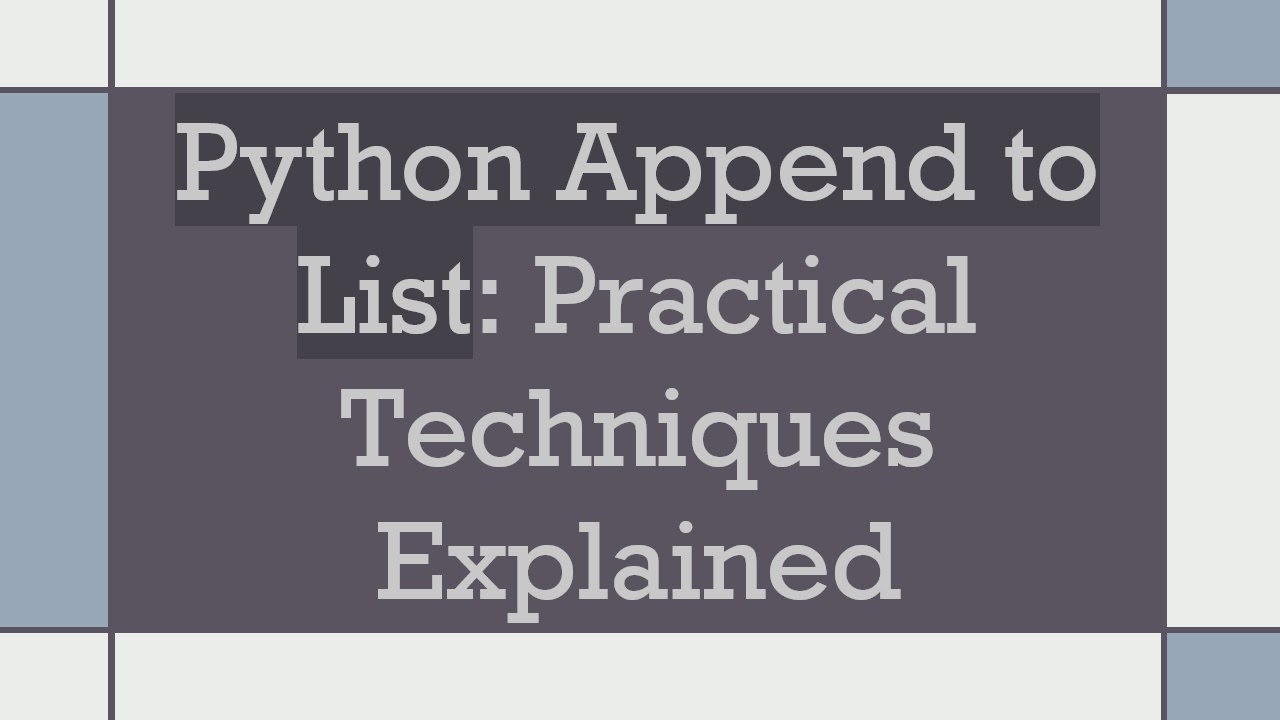
Показать описание
Summary: This guide explores various techniques to append items to a list in Python, including checking for duplicates and handling None values. Detailed explanations and code examples are provided for Python programmers.
---
Python Append to List: Practical Techniques Explained
Appending items to a list is a fundamental operation in Python. Whether you're just starting or you're a seasoned developer, mastering list operations can significantly enhance your coding efficiency. This post covers different techniques for appending items to a list, including conditional appending and avoiding duplicates.
Basic append() Method
The simplest way to add an item to a list in Python is by using the append method.
[[See Video to Reveal this Text or Code Snippet]]
This method always appends the item at the end of the list.
Append to List if the Item is Not in the List
Sometimes, you might want to add an item only if it is not already present in the list. Here’s how you can achieve that.
[[See Video to Reveal this Text or Code Snippet]]
The if item not in my_list condition ensures that the item is appended only if it does not exist in the list.
Append to List if the Item is Not None
In some cases, you might want to append an item only if it is not None. This is particularly useful when dealing with optional data.
[[See Video to Reveal this Text or Code Snippet]]
This condition checks whether the item is not None before appending it to the list.
Append Without Duplicates
If you need to append multiple items to a list while ensuring there are no duplicates, you might find it helpful to use a set for intermediate storage. This way, you can filter out duplicates efficiently.
[[See Video to Reveal this Text or Code Snippet]]
For larger data sets, consider converting the list to a set, adding the new items, and then converting it back to a list:
[[See Video to Reveal this Text or Code Snippet]]
This approach ensures that all duplicates are removed efficiently.
Conclusion
Appending items to a list in Python is a versatile and straightforward task, but when combined with conditions such as avoiding duplicates or checking for None, it adds an extra layer of robustness to your code. By leveraging Python's expressive syntax and data structures, you can write cleaner and more reliable code.
Feel free to experiment with these techniques to find what best fits your specific use case. Happy coding!
---
Python Append to List: Practical Techniques Explained
Appending items to a list is a fundamental operation in Python. Whether you're just starting or you're a seasoned developer, mastering list operations can significantly enhance your coding efficiency. This post covers different techniques for appending items to a list, including conditional appending and avoiding duplicates.
Basic append() Method
The simplest way to add an item to a list in Python is by using the append method.
[[See Video to Reveal this Text or Code Snippet]]
This method always appends the item at the end of the list.
Append to List if the Item is Not in the List
Sometimes, you might want to add an item only if it is not already present in the list. Here’s how you can achieve that.
[[See Video to Reveal this Text or Code Snippet]]
The if item not in my_list condition ensures that the item is appended only if it does not exist in the list.
Append to List if the Item is Not None
In some cases, you might want to append an item only if it is not None. This is particularly useful when dealing with optional data.
[[See Video to Reveal this Text or Code Snippet]]
This condition checks whether the item is not None before appending it to the list.
Append Without Duplicates
If you need to append multiple items to a list while ensuring there are no duplicates, you might find it helpful to use a set for intermediate storage. This way, you can filter out duplicates efficiently.
[[See Video to Reveal this Text or Code Snippet]]
For larger data sets, consider converting the list to a set, adding the new items, and then converting it back to a list:
[[See Video to Reveal this Text or Code Snippet]]
This approach ensures that all duplicates are removed efficiently.
Conclusion
Appending items to a list in Python is a versatile and straightforward task, but when combined with conditions such as avoiding duplicates or checking for None, it adds an extra layer of robustness to your code. By leveraging Python's expressive syntax and data structures, you can write cleaner and more reliable code.
Feel free to experiment with these techniques to find what best fits your specific use case. Happy coding!