filmov
tv
Python Tutorial : Constants and variables
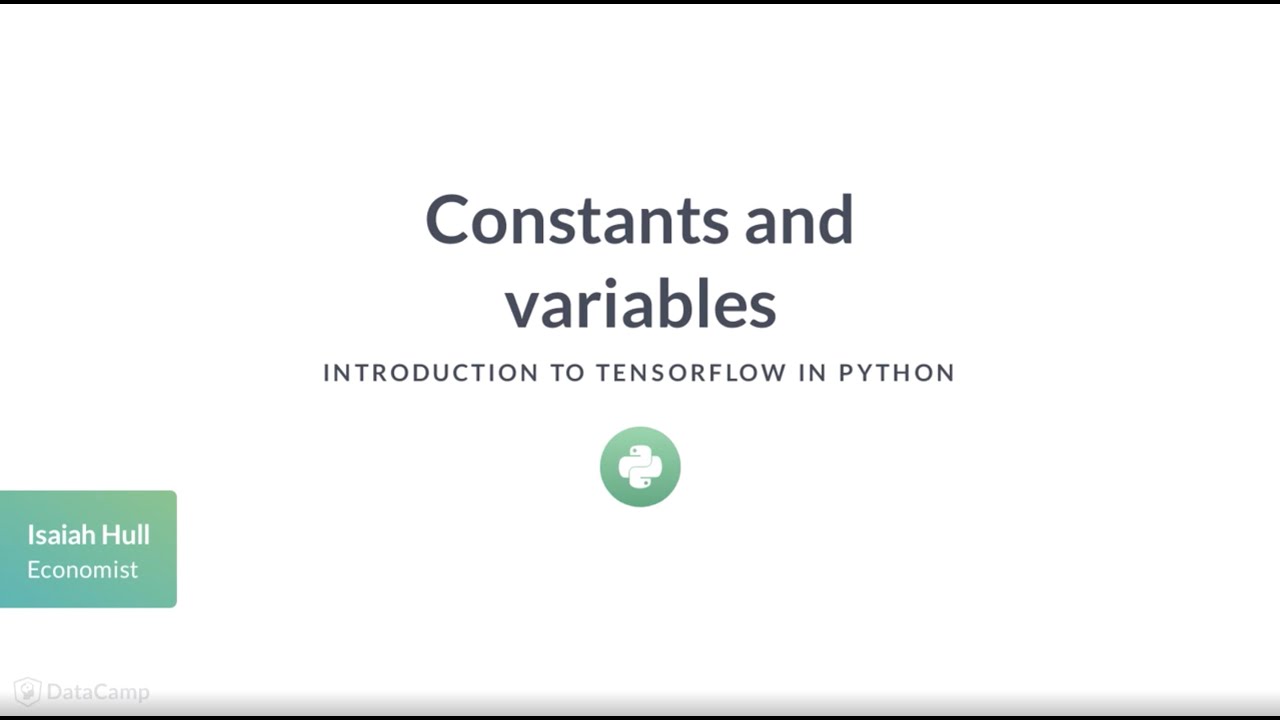
Показать описание
---
Hi! My name is Isaiah Hull and this is a course on the fundamentals of the TensorFlow API in Python. In our first video, we will briefly introduce TensorFlow and then discuss its two basic objects of computation: constants and variables.
TensorFlow is an open-source library for graph-based numerical computation.
It was developed by the Google Brain Team.
It has both low and high level APIs.
You can use TensorFlow to perform addition, multiplication, and differentiation.
You can also use it to design and train machine learning models.
TensorFlow two point zero brought with it substantial changes.
Eager execution is now enabled by default, which allows users to write simpler and more intuitive code.
Additionally, model building is now centered around the Keras and Estimator's high-level APIs.
The TensorFlow documentation describes a tensor as "a generalization of vectors and matrices to potentially higher dimensions." Now, if you are not familiar with linear algebra, you can simply think of a tensor as a collection of numbers, which is arranged into a particular shape.
As an example, let's say you have a slice of bread and you cut it into 9 pieces. One of those 9 pieces is a 0-dimensional tensor. This corresponds to a single number. A collection of 3 pieces that form a row or column is a 1-dimensional tensor. All 9 pieces together are a 2-dimensional tensor. And the whole loaf, which contains many slices, is a 3-dimensional tensor.
Now that you know what a tensor is, let's define a few. We will start by importing TensorFlow as tf. We will then define 0-, 1-, 2-, and 3-dimensional tensors.
Note that each object will be a tf dot Tensor object.
If we want to print the array contained in that object, we can apply the dot numpy method and pass the resulting object to the print function.
We next move on to constants, which are the simplest category of tensor in TensorFlow.
A constant does not change and cannot be trained.
It can, however, have any dimension.
In the code block, we've defined two constants. The constant a is a 2x3 tensor of 3s.
The constant b is a 2x2 tensor, which is constructed from the 1-dimensional tensor: 1, 2, 3, 4.
In the previous slide, we worked exclusively with the constant operation. However, in some cases, there are more convenient options for defining certain types of special tensors. You can use the zeros or ones operations to generate a tensor of arbitrary dimension that is populated entirely with zeros or ones. You can use the zeros_like or ones_like operations to populate tensors with zeros and ones, copying the dimension of some input tensor. Finally, you can use the fill operation to populate a tensor of arbitrary dimension with the same scalar value in each element.
Unlike a constant, a variable's value can change during computation. The value of a variable is shared, persistent, and modifiable. However, its data type and shape are fixed.
Let's take a look at how variables are constructed and used in TensorFlow. In the code, we first define a variable, a0, which is a 1-dimensional tensor with 6 elements. We can set its datatype to a 32-bit float or something else, such as a 16-bit int, as we have for a1.
We then define a constant, b.
It's now time to put what you've learned to use in some exercises.
#DataCamp #PythonTutorial #TensorFlowinPython