filmov
tv
How to Split a String in Java
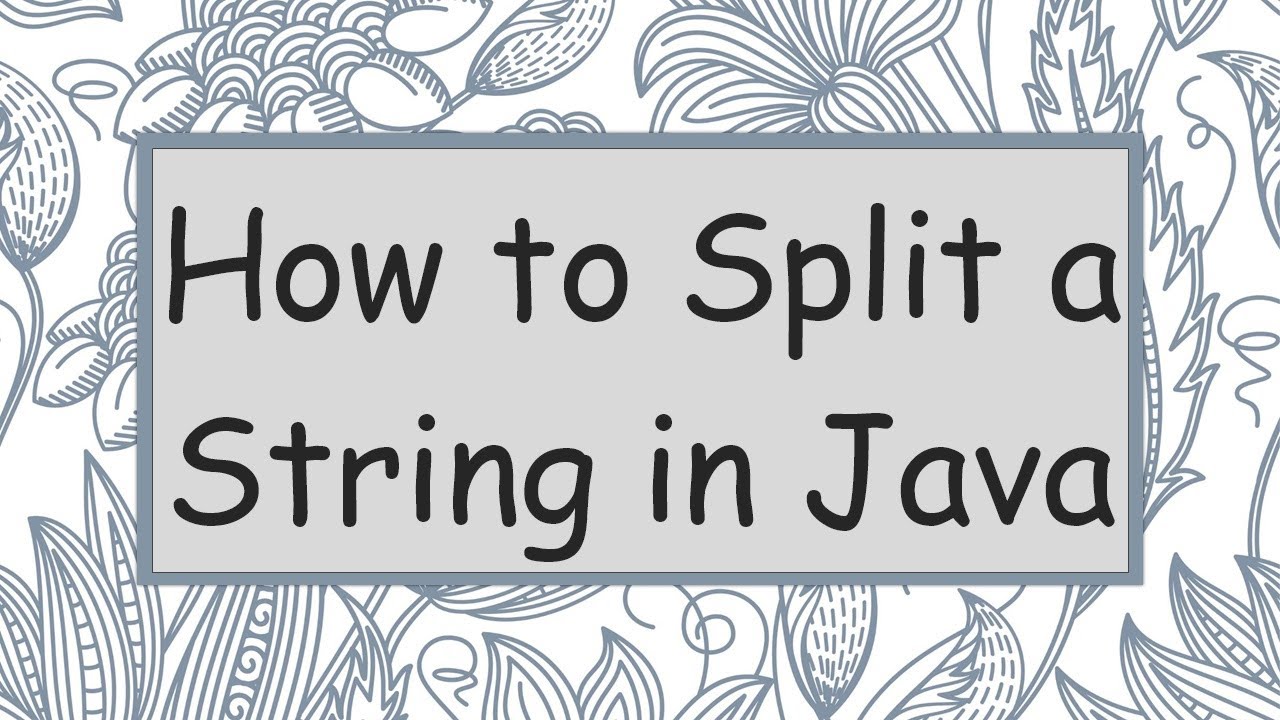
Показать описание
Summary: Learn various methods to split a string in Java, including using the `split` method, `StringTokenizer`, and other advanced techniques for efficient string manipulation.
---
Splitting a string is a common task in Java programming, often needed for processing data, parsing input, or manipulating text. Java provides several ways to split a string, each with its own use cases and advantages. This guide covers the most common and effective methods.
Using the split Method
The split method is part of the String class and is the most straightforward way to split a string based on a specified delimiter.
Syntax
[[See Video to Reveal this Text or Code Snippet]]
Example
[[See Video to Reveal this Text or Code Snippet]]
In this example, the string "apple,orange,banana" is split at each comma, resulting in an array of strings: ["apple", "orange", "banana"].
Using the split Method with Limit
You can also limit the number of splits by providing a second argument to the split method.
Syntax
[[See Video to Reveal this Text or Code Snippet]]
Example
[[See Video to Reveal this Text or Code Snippet]]
In this case, the string is split into a maximum of three parts: ["one", "two", "three:four"].
Using StringTokenizer
StringTokenizer is a legacy class in Java, but it can still be useful for simple string splitting tasks.
Example
[[See Video to Reveal this Text or Code Snippet]]
Using Pattern and Matcher
Example
[[See Video to Reveal this Text or Code Snippet]]
In this example, the string is split using multiple delimiters (comma, semicolon, and pipe), and the result is trimmed for extra spaces.
Conclusion
Java provides multiple ways to split a string, each suitable for different scenarios. The split method is versatile and easy to use, while StringTokenizer offers a simpler approach for basic tasks. For more advanced requirements, Pattern and Matcher give you the power to handle complex delimiters and regular expressions. Understanding these methods allows you to choose the best tool for your specific needs and ensures efficient string manipulation in your Java applications.
---
Splitting a string is a common task in Java programming, often needed for processing data, parsing input, or manipulating text. Java provides several ways to split a string, each with its own use cases and advantages. This guide covers the most common and effective methods.
Using the split Method
The split method is part of the String class and is the most straightforward way to split a string based on a specified delimiter.
Syntax
[[See Video to Reveal this Text or Code Snippet]]
Example
[[See Video to Reveal this Text or Code Snippet]]
In this example, the string "apple,orange,banana" is split at each comma, resulting in an array of strings: ["apple", "orange", "banana"].
Using the split Method with Limit
You can also limit the number of splits by providing a second argument to the split method.
Syntax
[[See Video to Reveal this Text or Code Snippet]]
Example
[[See Video to Reveal this Text or Code Snippet]]
In this case, the string is split into a maximum of three parts: ["one", "two", "three:four"].
Using StringTokenizer
StringTokenizer is a legacy class in Java, but it can still be useful for simple string splitting tasks.
Example
[[See Video to Reveal this Text or Code Snippet]]
Using Pattern and Matcher
Example
[[See Video to Reveal this Text or Code Snippet]]
In this example, the string is split using multiple delimiters (comma, semicolon, and pipe), and the result is trimmed for extra spaces.
Conclusion
Java provides multiple ways to split a string, each suitable for different scenarios. The split method is versatile and easy to use, while StringTokenizer offers a simpler approach for basic tasks. For more advanced requirements, Pattern and Matcher give you the power to handle complex delimiters and regular expressions. Understanding these methods allows you to choose the best tool for your specific needs and ensures efficient string manipulation in your Java applications.